Zero Allocation in Golang vs. Traditional Memory Management Techniques
Learn how zero allocation in Go (Golang) can optimize memory usage, reduce garbage collection overhead, and create more efficient and scalable applications.
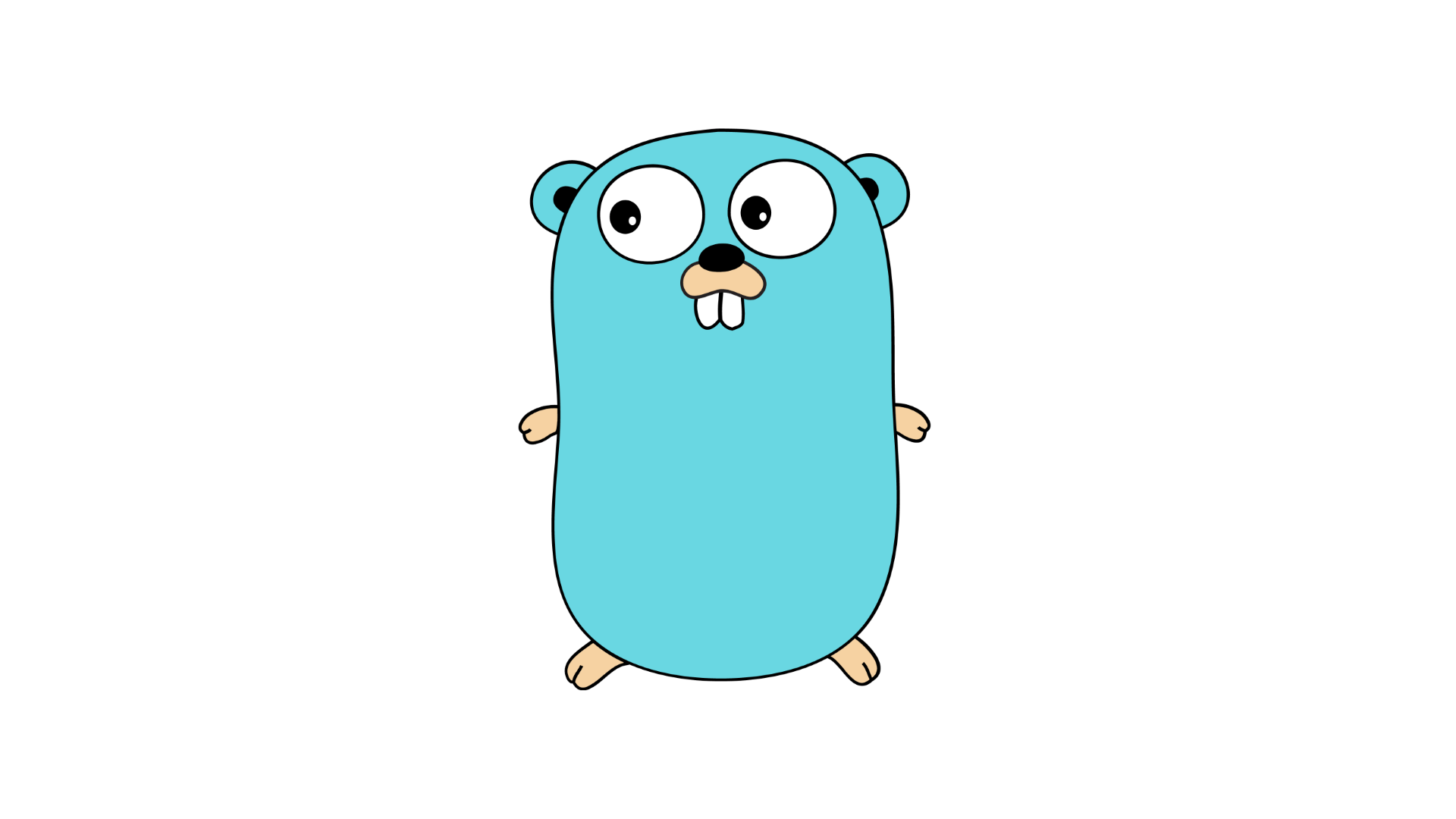
Zero Allocation in Golang vs. Traditional Memory Management Techniques
As a developer, you're probably aware of the challenges involved in memory management. Efficient memory allocation and deallocation can significantly impact the performance of your applications. In this article, we'll discuss the concept of zero allocation in Go (Golang) and compare it with traditional memory management techniques. By understanding the advantages of zero allocation, you can optimize your code and create more performant applications.
What is Zero Allocation?
In programming, memory allocation refers to reserving memory for storing variables, data structures, and other objects. When memory is allocated, the operating system assigns a specific chunk of memory to hold the data. Over time, as applications allocate and deallocate memory, fragmentation can occur, leading to inefficient memory usage.
Zero allocation, on the other hand, refers to an approach that minimizes or eliminates unnecessary memory allocations. In a language like Go, where memory allocation is managed automatically, zero allocation becomes particularly relevant.
Zero allocation doesn't mean that memory is never allocated. Instead, it aims to minimize allocations by reusing memory whenever possible. By avoiding unnecessary memory allocations and reducing garbage collection, zero allocation can improve the overall performance of your Go programs.
Traditional Memory Management Techniques
1. Manual Memory Management
In low-level programming languages like C or C++, manual memory management is the norm. Developers must explicitly allocate and deallocate memory using functions like malloc
and free
.
While manual memory management provides fine-grained control over memory, it can be error-prone and time-consuming. Developers need to carefully manage the lifecycle of allocated memory, ensuring it's freed when no longer needed. Incorrect memory management can lead to memory leaks, dangling pointers, or segmentation faults.
2. Automatic Garbage Collection (GC)
In languages like Java or Python, automatic garbage collection (GC) handles memory management. GC works by tracking allocated memory and periodically freeing memory that is no longer being used.
Automatic GC relieves developers from explicitly managing memory, but it introduces overhead and can impact performance. GC relies on algorithms like mark-and-sweep or generational garbage collection, which can cause pauses in program execution and impact real-time or low-latency applications.
Zero Allocation in Go
Go takes a different approach to memory management compared to traditional languages. It combines automatic memory management with a focus on reducing unnecessary allocations.
By utilizing features like value semantics and escape analysis, Go minimizes object allocations and reuses memory more efficiently. Let's explore some techniques that enable zero allocation in Go:
1. Using Value Semantics
Value semantics in Go allows you to pass data by value rather than by reference. When passing data as values, Go creates copies of the data instead of passing pointers to the original data. This approach reduces the need for allocations and garbage collection.
By leveraging value semantics, you can optimize memory usage and improve the performance of your Go programs. However, it's essential to be mindful of large data structures that may cause excessive copying.
2. Stack Allocation
In Go, small objects are typically allocated on the stack, which is faster than heap allocation. The stack is a region of memory that stores local variables and function call information.
Go's escape analysis determines whether an object can be allocated on the stack or needs to be allocated on the heap. Objects that escape the scope of their defining function are allocated on the heap and handled by the garbage collector. Objects that do not escape can be allocated on the stack.
By taking advantage of stack allocation, you can reduce the overhead of heap allocation and improve the performance of your Go programs.
3. Using sync.Pool
The sync.Pool
package in Go provides a mechanism for implementing object pools. An object pool is a cache of reusable objects that can be shared across goroutines.
By using sync.Pool
, you can avoid unnecessary memory allocations by reusing objects instead. This technique is particularly useful for frequently created and discarded objects, such as temporary buffers or database connections.
Benefits of Zero Allocation
Zero allocation brings several benefits to your Go programs:
- Better Performance: By minimizing memory allocations, zero allocation reduces the overhead of garbage collection and improves the overall performance of your applications.
- Reduced GC Pauses: With fewer allocations, garbage collection pauses become shorter and less frequent, resulting in more responsive and reliable applications.
- Improved Scalability: By optimizing memory usage, zero allocation allows applications to handle more requests concurrently, enhancing scalability.
Limitations and Considerations
While zero allocation can greatly improve the performance of your Go programs, it's important to be aware of some limitations and considerations:
- Trade-off with Complexity: Zero allocation techniques often require more careful thought and design decisions, adding complexity to your code. It's crucial to measure the performance gains against the increased complexity to ensure it's worth the trade-off.
- Avoid Premature Optimization: It's essential to focus on readability, maintainability, and correctness first. Only optimize for zero allocation when you've identified it as a performance bottleneck through profiling or benchmarking.
- Benchmarking and Profiling: To verify the performance gains of your zero allocation optimizations, use benchmarking and profiling tools to measure the impact on memory usage, CPU usage, and execution time.
Conclusion
Zero allocation in Go offers a compelling approach to memory management that can significantly improve the performance of your applications. By minimizing unnecessary memory allocations, you can optimize memory usage, reduce garbage collection overhead, and create more efficient and scalable Go programs.
Keep in mind that zero allocation is not a silver bullet and should be used judiciously, considering the trade-offs and the specific needs of your application. With careful consideration and benchmarking, you can leverage zero allocation techniques to create high-performing Go applications.
Happy coding!