Understanding Zero Allocation in Golang: A Comprehensive Guide
Understanding zero allocation is essential for writing efficient and scalable Go code. Minimizing memory allocations and reducing strain on the garbage collector can significantly improve performance. Learn how to achieve zero allocation in Go with these techniques.
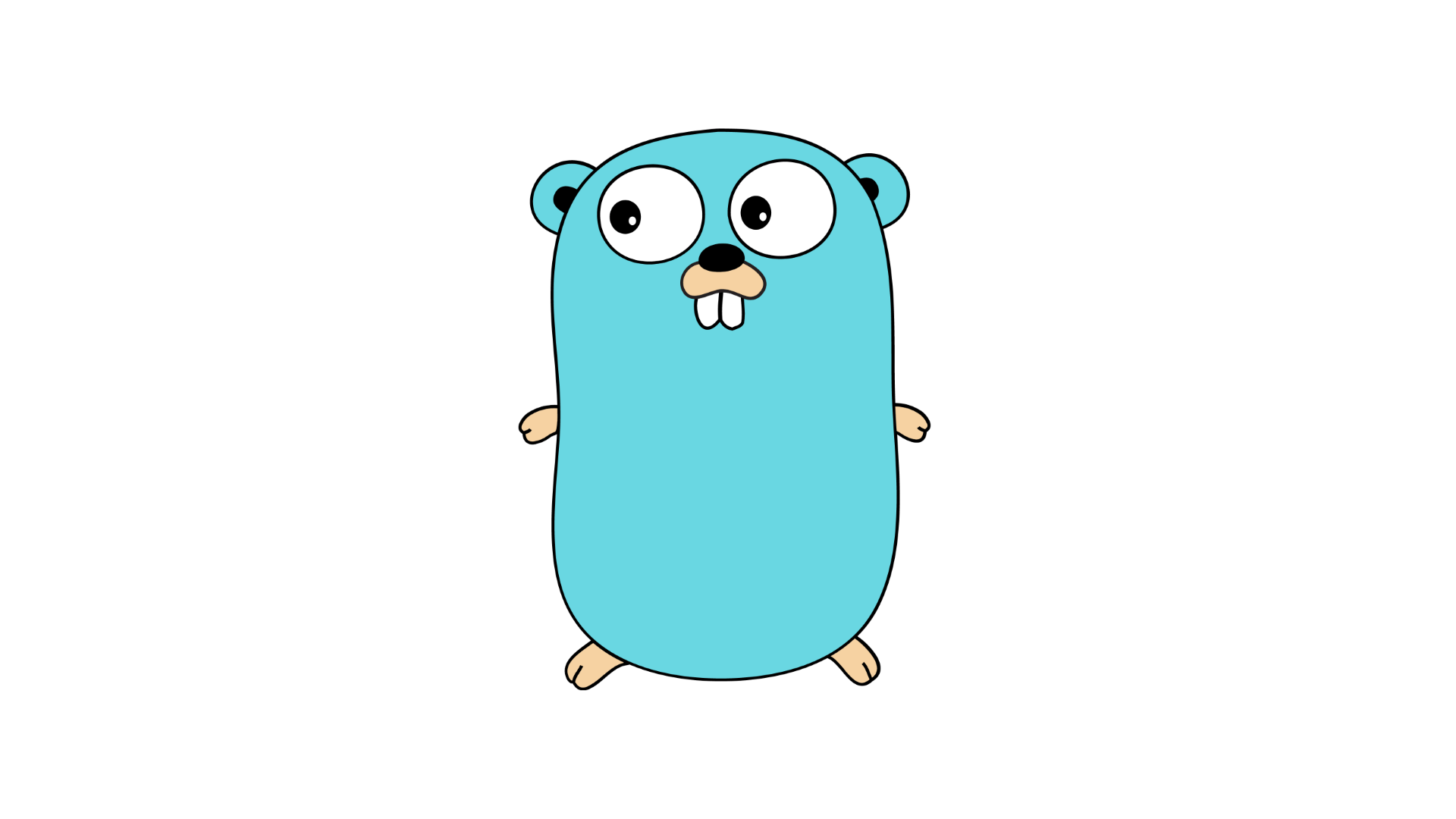
Introduction
Zero allocation is a key concept in the Go programming language (Golang) that plays a crucial role in optimizing memory usage and improving performance. Understanding zero allocation is essential for writing efficient and scalable Go code. This comprehensive guide will take you through everything you need to know about zero allocation in Golang, from what it is to how to achieve it in your code. Let's dive in!
What is Zero Allocation?
Zero allocation refers to the process of minimizing or completely eliminating the creation of new heap-allocated objects in your code. In other words, it's about reducing memory allocation during the execution of your program in order to improve performance and reduce the burden on the garbage collector.
Why is Zero Allocation Important?
Zero allocation is critical in performance-sensitive applications where minimizing memory allocations and garbage collection overhead can significantly improve execution speed and reduce latency. By reducing the number of objects created on the heap, you can optimize memory usage and prevent unnecessary garbage collection pauses.
How Does Allocation Work in Golang?
In Golang, memory allocation generally occurs on the heap. When a new object is created using the new
keyword or the make
function, it is allocated on the heap. The heap is a region of memory governed by the garbage collector that is responsible for managing object lifecycles.
Here's an example:
```go func createUser() *User { user := new(User) // Allocates a new User struct on the heap user.ID = 1 user.Name = "John Doe" return user } ```
Achieving Zero Allocation
While complete zero allocation may not always be feasible or practical, there are several techniques you can employ in Golang to minimize memory allocations and reduce the strain on the garbage collector. Let's explore these techniques:
Stack Allocation
One way to achieve zero allocation is by leveraging stack allocation. Unlike heap allocation, where objects persist beyond their scope, stack allocation creates objects with a limited lifespan that are automatically deallocated when they go out of scope.
Golang, however, doesn't provide direct control over stack allocation like low-level languages do. It relies heavily on the compiler to optimize stack allocation. By following best practices like creating small and simple functions, you can increase the chances of objects being allocated on the stack rather than the heap.
Here's an example:
```go func processRequest() { buffer := [512]byte{} // buffer is allocated on the stack // perform operations using buffer } ```
Sync.Pool
The sync.Pool
package in Golang provides a mechanism for recycling objects, reducing the number of allocations and deallocations. It is particularly useful in scenarios where objects are frequently created and discarded.
By using a sync.Pool
, you can reuse objects instead of creating new ones, reducing allocation overhead and freeing up memory. It's important to note that objects obtained from a pool should be properly reset before use to avoid potential data corruption.
Here's an example:
```go var userPool = sync.Pool{ New: func() interface{} { return &User{} }, } func getUser() *User { user := userPool.Get().(*User) // perform operations on user object return user } func releaseUser(user *User) { // reset user object userPool.Put(user) } ```
Byte Slice Pooling
Byte slice pooling is a technique where you use a sync.Pool specifically for byte slices. This technique is particularly effective in scenarios where you frequently allocate and deallocate byte slices, such as when working with network connections or parsing large amounts of data.
By reusing byte slices, you can reduce memory allocations and avoid unnecessary garbage collection. It's important to remember that pooled byte slices should be cleared or reset before use to avoid potential data leaks or corruption.
Here's an example:
```go var bufferPool = sync.Pool{ New: func() interface{} { return make([]byte, 0, 1024) }, } func processRequest(data []byte) { buffer := bufferPool.Get().([]byte) buffer = append(buffer[:0], data...) // perform operations using buffer bufferPool.Put(buffer[:0]) } ```
Memory Pooling Libraries
Aside from the built-in sync.Pool
, Golang offers several third-party memory pooling libraries that provide more advanced memory pooling capabilities. These libraries provide additional features and optimizations for different use cases, allowing you to fine-tune memory allocation and improve performance.
Some popular memory pooling libraries in Golang include:
- bytebufferpool: A byte buffer pooling library
- klauspost/compress: A compression library with built-in memory pooling
- offheap: An off-heap memory allocation library
Measuring Allocation
To effectively optimize memory allocation in Golang, you need to measure the impact of your changes. Fortunately, Golang provides tools and techniques for measuring memory allocation and profiling your code.
The runtime
package in Golang provides functions for allocating and analyzing memory usage. You can use the runtime.MemStats
struct to collect memory allocation data and identify potential bottlenecks in your code.
Additionally, Golang comes with a built-in benchmarking tool, the testing
package, which allows you to measure the performance of your code over time. By benchmarking your code, you can track the impact of optimizations on memory allocation and overall performance.
Conclusion
Congratulations! You now have a comprehensive understanding of zero allocation in Golang. By minimizing memory allocations and optimizing memory usage, you can significantly improve the performance and efficiency of your Golang applications. Remember to measure the impact of your changes and experiment with different techniques to find the optimal balance between memory usage and performance.
Stay tuned for more in-depth guides on Golang performance and optimization. Happy coding!