Zero Allocation Garbage Collection in Golang: How It Works
Zero Allocation Garbage Collection in Go is a powerful feature that minimizes memory allocation, improves performance, and simplifies code maintenance. Learn how it works and its advantages for building high-performance applications.
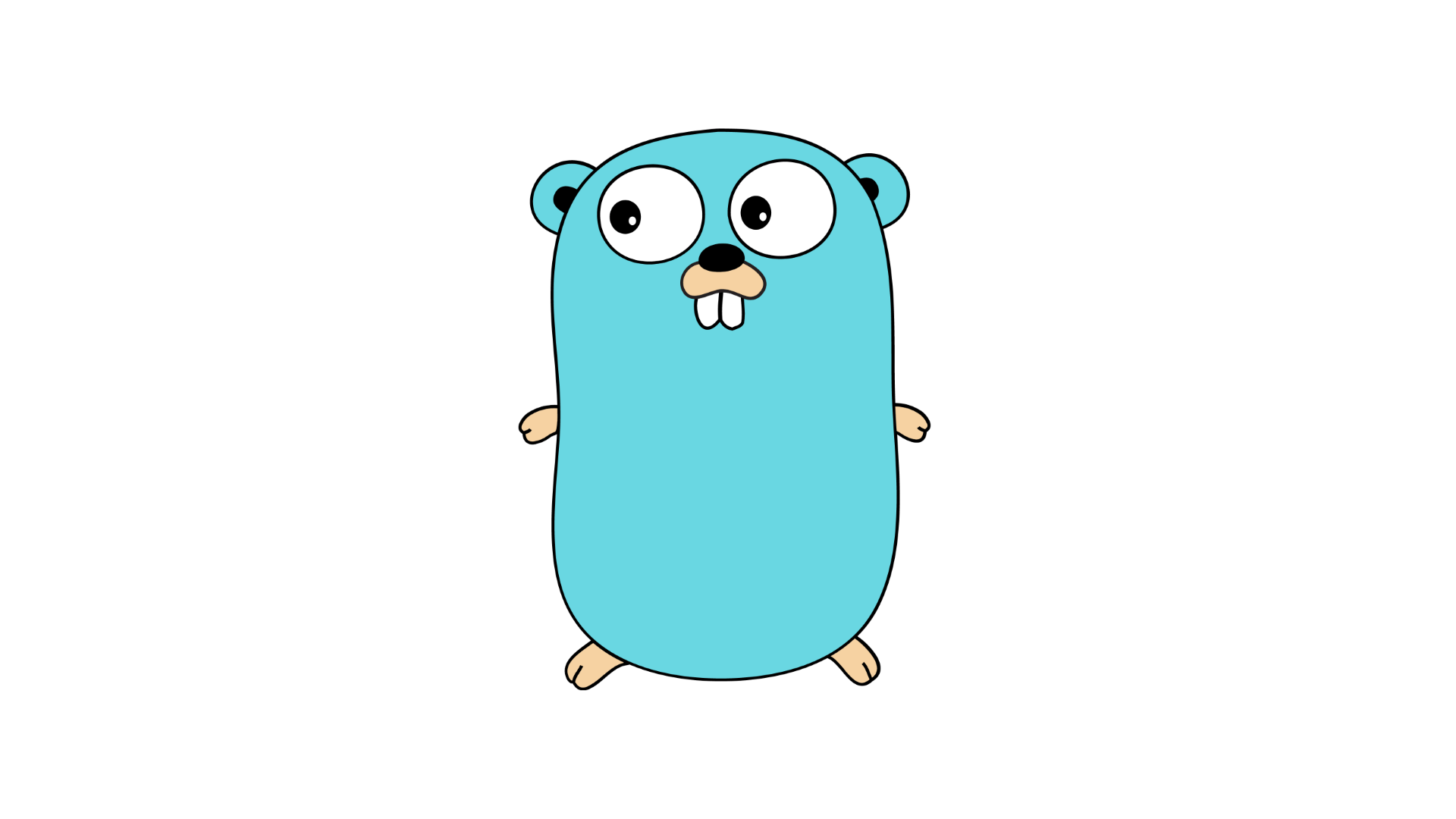
Introduction
Zero Allocation Garbage Collection (GC) in Go is one of the language's most powerful features. It helps developers build high-performance applications by efficiently managing memory allocation and deallocation. Understanding how Zero Allocation GC works is crucial for optimizing your Go code and improving your application's overall performance.
What is Garbage Collection?
In computer science, garbage collection refers to the automatic memory management process. It involves tracking and reclaiming memory that is no longer in use by a program. The primary goal of garbage collection is to free up memory and prevent memory leaks.
Why is Memory Management Important in Go?
Go is a statically-typed language with automatic memory management. In other words, Go takes care of memory allocation and deallocation automatically, eliminating the need for manual memory management. This feature simplifies code and reduces the likelihood of memory-related errors such as leaks and dangling pointers.
How Does Garbage Collection Work in Go?
Go uses a concurrent, tri-color, mark-and-sweep algorithm for garbage collection. Let's break down each step of the process:
1. Tracing
During the tracing phase, the garbage collector traverses the object graph to identify all reachable objects. Starting from the root objects (global variables, stack frames, etc.), it follows pointers to discover all live objects and marks them as reachable. Any objects not reached by the traversal are considered unreachable and will be eligible for garbage collection.
2. Marking
Once the tracing phase is complete, the garbage collector marks all reachable objects as "black". It accomplishes this by setting a bit in the object's header to indicate it is in use. This marking prevents the objects from being freed during the sweep phase.
3. Sweeping
In the sweeping phase, the garbage collector scans the entire heap and reclaims all "white" objects. These objects have not been marked during the tracing phase and are considered garbage. The memory occupied by the garbage objects is returned to the operating system, making it available for future allocations.
4. Cycle Detection
Go's garbage collector has a cycle detection mechanism to handle objects that have circular references. In situations where objects reference each other in a cyclic manner, the garbage collector identifies these cycles and ensures they are not prematurely freed.
What is Zero Allocation Garbage Collection?
In traditional garbage collectors, objects often need to be allocated and deallocated, resulting in memory overhead. Zero Allocation GC aims to minimize memory allocation by reusing memory from objects that are no longer in use instead of deallocating and reallocating memory.
How Does Zero Allocation Garbage Collection Work in Go?
Zero Allocation GC in Go achieves memory reuse through a technique called "stack allocation". It leverages the knowledge that objects residing on the stack are short-lived and can be safely reused without incurring additional memory overhead.
Here's how the process works in more detail:
1. Escaping
In Go, when an object is created within a function, it can either escape or stay on the stack. An object is said to "escape" if it is referenced after the function returns, meaning it needs to be allocated on the heap. If an object doesn't escape, it can be safely allocated on the stack.
2. Stack Allocation
Objects that don't escape are allocated on the stack instead of the heap. This allows for quick allocation and deallocation of memory, as the stack frame is automatically managed by the Go runtime. Once the function returns, the memory occupied by the object is automatically reclaimed without any additional overhead.
3. Eliminating Garbage Collection Overhead
By using stack allocation for short-lived objects that don't escape, Zero Allocation GC significantly reduces the number of object allocations and deallocations, leading to improved performance. This technique minimizes the amount of work the garbage collector needs to do, as it eliminates the need to scan and sweep short-lived objects.
Advantages of Zero Allocation Garbage Collection
Zero Allocation Garbage Collection offers several benefits for Go developers:
- Improved Performance: By minimizing memory allocation and deallocation, Zero Allocation GC reduces overhead and improves application performance.
- Reduced Memory Fragmentation: Zero Allocation GC helps prevent memory fragmentation by reusing short-lived objects, resulting in more efficient memory utilization.
- Easier Maintenance: With Zero Allocation GC, developers don't need to manually manage memory, reducing the likelihood of memory-related bugs and simplifying code maintenance.
Limitations of Zero Allocation Garbage Collection
While Zero Allocation GC offers significant advantages, it also has some limitations:
- Limited Reuse: Zero Allocation GC works best for short-lived objects that don't escape. Objects with longer lifetimes or objects that frequently escape may still need to be allocated on the heap.
- Complexity: Zero Allocation GC introduces additional complexity to the memory management process, as developers need to consider object lifetimes and escape analysis to maximize memory reuse.
Conclusion
Zero Allocation Garbage Collection in Go is a powerful feature that enables developers to optimize memory allocation and deallocation. By leveraging stack allocation for short-lived objects that don't escape, developers can minimize memory overhead and improve their application's performance. While Zero Allocation GC has some limitations, its advantages make it a valuable tool for building high-performance Go applications.
Now that you understand how Zero Allocation GC works in Go, you can start applying this knowledge to optimize your own code and create faster, more efficient applications. Happy coding!