Go by Example: Hello World
Learn how to write your first Go program! In this tutorial, we'll guide you through the process of creating a "Hello, World!" program in Go. Get started with the basics of Go programming and explore the syntax and structure of a Go program.
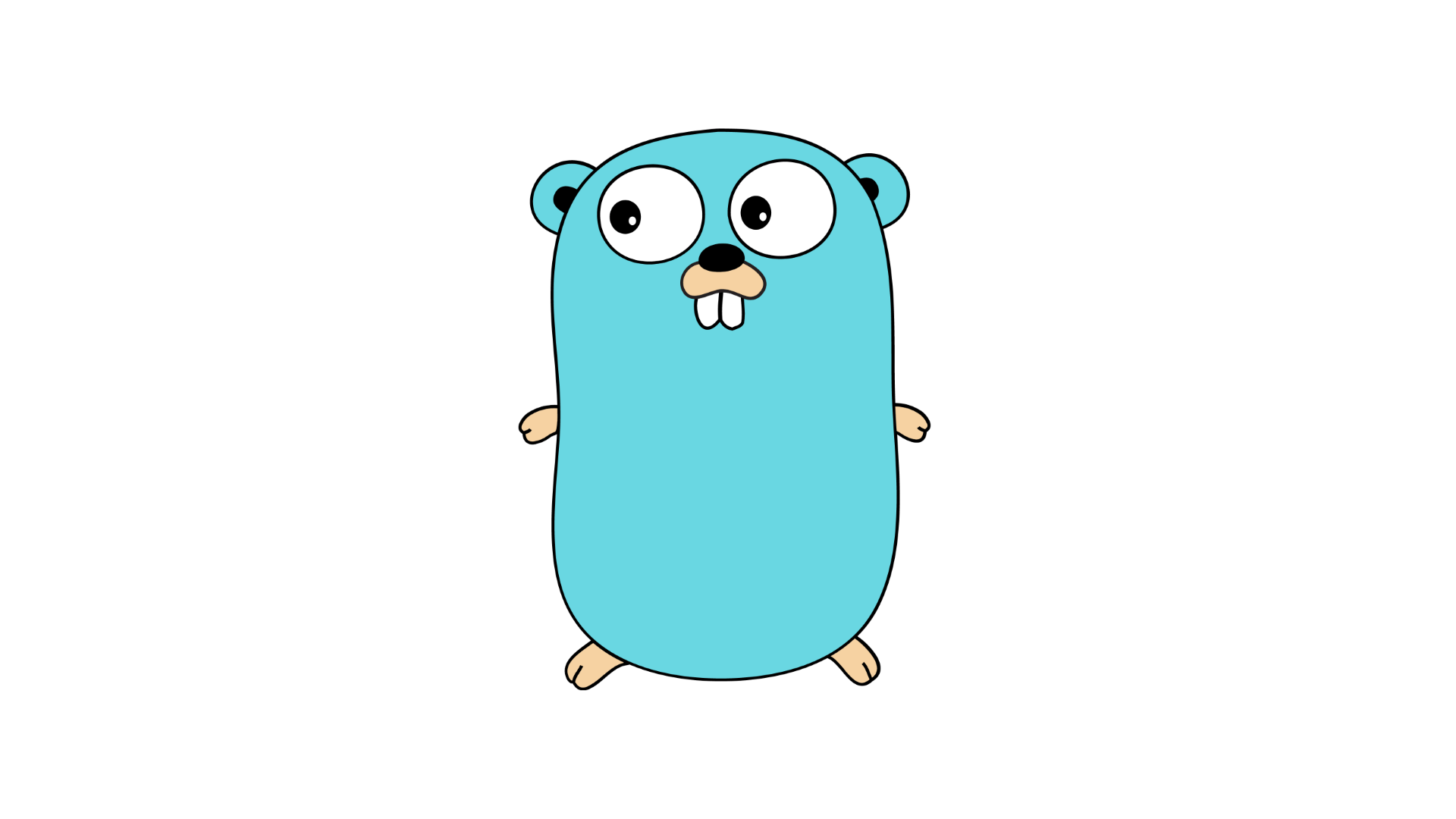
Introduction
Welcome to the first part of our Go by Example tutorial series! In this tutorial, we'll be exploring the basics of Go programming by starting with the classic "Hello, World!" program. Whether you're new to programming or just getting started with Go, this article will guide you through the process of writing your first Go program.
Prerequisites
Before we begin, make sure you have Go installed on your machine. You can download and install Go by following the instructions on the official Go website: https://golang.org/ Once you have Go installed, you're ready to go!
Writing Your First Go Program
Now that you have Go installed, let's dive in and write our first Go program. Follow the steps below:
Step 1: Create a New Directory
Start by creating a new directory for your Go program. Open your terminal or command prompt and navigate to the location where you want to create the directory. Use the following command to create a new directory:
mkdir hello-world
cd hello-world
Step 2: Create a New File
Next, create a new file inside the directory you just created. Use any text editor of your choice. For example, if you're using the command line, you can use the touch
command to create a new file:
touch main.go
Step 3: Write the Code
Open the main.go
file in your text editor and add the following code:
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
Let's break down the code. First, we declare the package name as "main" – this tells Go that this is the main package and that it should be compiled as an executable program. Then, we import the "fmt" package, which provides functions for formatted I/O. Finally, we define the main
function, which is the entry point of our program. Inside the main
function, we use the fmt.Println
function to print the string "Hello, World!" to the console.
Step 4: Compile and Run the Program
To compile and run the program, open your terminal or command prompt and navigate to the directory where the main.go
file is located. Then, use the following command to compile the program:
go build
This will create an executable file named hello-world
in the same directory. Now, to run the program, simply type the following command:
./hello-world
And voila! You should see the output:
Hello, World!
Conclusion
Congratulations! You've successfully written and run your first Go program. "Hello, World!" might be a simple program, but it serves as a solid foundation for understanding the basics of Go programming. Now that you've got your feet wet, you're ready to explore more complex Go programs and delve into the vast world of Go programming.
In the next part of our tutorial series, we'll delve deeper into Go syntax, data types, and variables. Stay tuned for more Go by Example tutorials!