Writing Clean and Maintainable Code in Golang: Best Practices
Learn the best practices for writing clean and maintainable code in Go. From naming conventions to error handling, these tips will level up your coding skills and improve code quality. Build robust and scalable applications with simplicity and efficiency in mind.
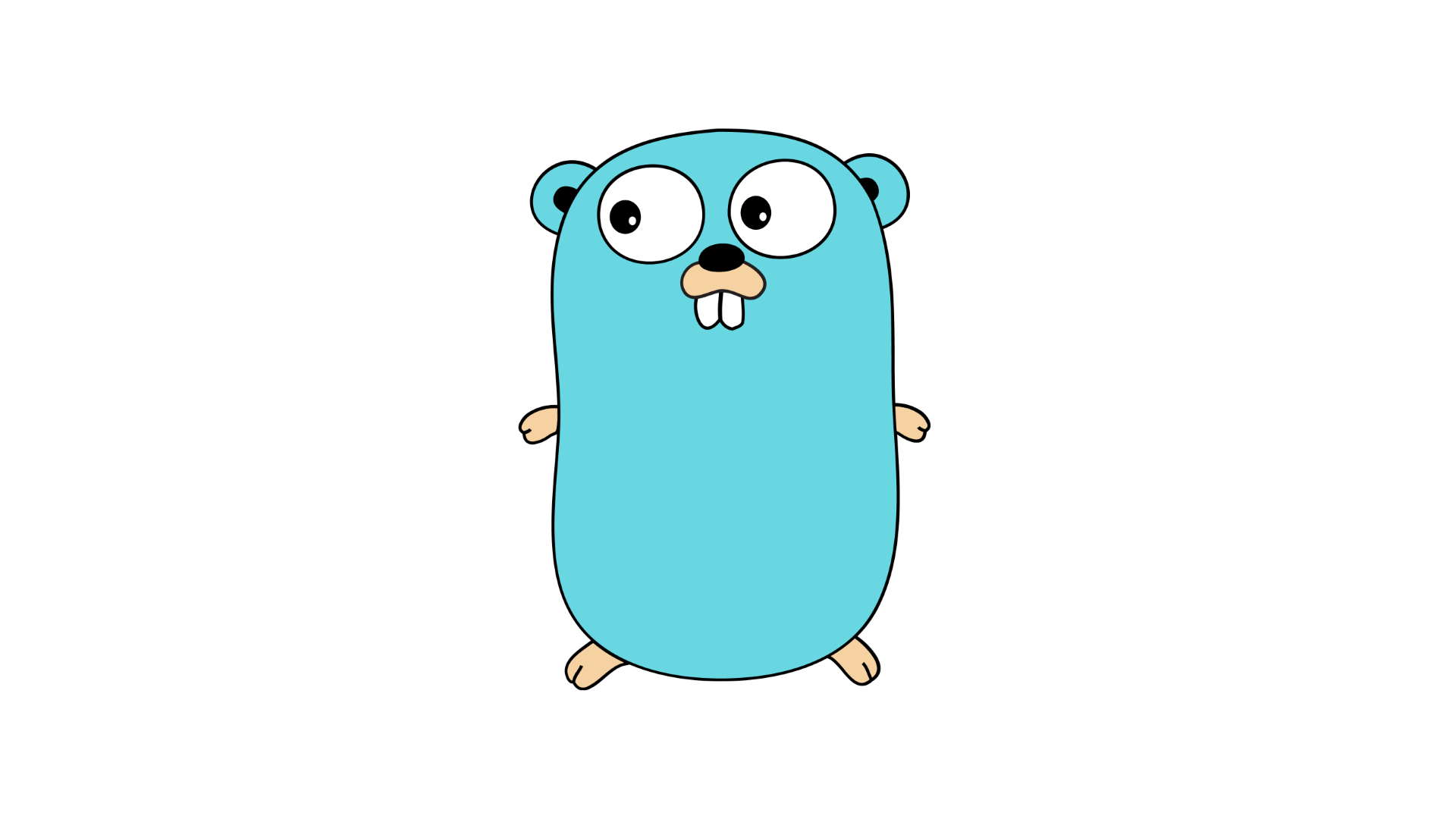
Introduction
Writing clean and maintainable code is crucial for every developer, regardless of their programming language. In the world of Go (Golang), this principle is no different. As a statically typed language, Go emphasizes simplicity, readability, and efficiency. In this blog post, we'll explore some best practices for writing clean and maintainable code in Go, enabling you to build robust and scalable applications. Let's get started!
1. Use Proper Naming Conventions
One of the key elements of writing clean and maintainable code is using consistent and meaningful naming conventions. By following these conventions, your code becomes more readable and easier to understand. Here are some best practices when it comes to naming:
- Use lowercase letters to define package names.
- Use concise and descriptive names for variables, functions, and types.
- Avoid unnecessary abbreviations and acronyms.
- Use camelCase (initial lowercase) for local variables and PascalCase (initial uppercase) for exported variables.
- Be consistent with naming conventions across your codebase.
By adhering to proper naming conventions, you'll enhance code readability and maintainability, making it easier for other developers (including yourself) to understand your code.
2. Write Short and Readable Functions
Function length plays a significant role in code maintainability. Ideally, functions should be short and focused, performing a single task. This approach enhances code readability and makes it easier to test and debug. Here are some tips for writing short and readable functions:
- Aim for functions with a maximum of 15 lines of code.
- Break down complex logic into smaller, reusable functions.
- Use clear and descriptive function names that reflect their purpose.
- Avoid deep nesting and excessive if-else statements.
- Use comments to explain the purpose and functionality of the function.
By following these practices, you'll create functions that are easier to understand, test, and maintain.
3. Encapsulate and Abstract
Encapsulation and abstraction are fundamental concepts in software development, and they play a significant role in writing clean and maintainable code in Go. By encapsulating data and functionality, you enhance code modularity and prevent direct access to internal implementation details. Here's how you can achieve encapsulation and abstraction:
- Use structs to encapsulate related data and behavior.
- Use private fields to hide implementation details.
- Expose only the necessary methods to interact with the struct.
- Separate concerns and delegate responsibilities to different structs.
- Avoid exposing global variables or functions.
By encapsulating and abstracting your code, you improve code maintainability by reducing dependencies and increasing modularity.
4. Error Handling
Proper error handling is vital for writing clean and maintainable code in Go. It helps prevent unexpected crashes and improves code reliability. Here are some best practices for error handling:
- Always check and handle errors returned by functions.
- Use the
errors
package to create custom error types. - Handle errors as early as possible, closer to the source of the error.
- Use
defer
for cleanup actions and closing resources.
By following these practices, you'll create more robust applications that gracefully handle errors and provide better feedback to users.
5. Write Clear and Meaningful Comments
Comments are an essential part of any codebase, as they provide additional context and explanations for your code. Clear and meaningful comments can significantly improve code maintainability. Here are some tips for writing effective comments:
- Comment every non-trivial function and method to explain its purpose and functionality.
- Use comments to clarify complex logic or edge cases.
- Provide comments for exported types, methods, and variables.
- Update comments along with code changes to keep them relevant.
By writing clear and meaningful comments, you'll make your codebase more accessible to other developers and help them understand the purpose and functionality of your code.
6. Keep Functions and Packages Small
Maintaining small functions and packages is critical for code readability and maintainability. Here's why:
- Small functions are easier to read, test, and debug.
- Small packages are more focused and have fewer dependencies.
- Small packages can be reused in different projects.
- Small packages encourage modularity and separation of concerns.
Avoid creating large functions or packages that perform multiple tasks. Instead, aim for small, focused functions that perform a single task and small, reusable packages that encapsulate related functionality.
7. Version Control and Code Review
Version control and code review are essential practices for maintaining clean and maintainable code in Go. Here's why:
- Version control allows you to track changes and rollback if necessary.
- Code review helps identify potential issues and improve code quality.
Utilize version control systems like Git to track changes, collaborate with other developers, and manage code iterations. Perform code reviews to get feedback from other developers and ensure code quality and adherence to best practices.
8. Unit Testing
Unit testing is another vital aspect of writing clean and maintainable code in Go. By writing unit tests, you can verify the correctness of your code and catch bugs early on. Here are some best practices for unit testing:
- Write tests for every non-trivial function and method.
- Use the
testing
package from the standard library for writing tests. - Organize tests into separate test files and packages.
- Include edge cases and handle error scenarios in your tests.
- Run tests regularly as part of your development workflow.
By incorporating unit testing into your development process, you'll build more reliable and maintainable code.
Wrapping Up
Writing clean and maintainable code in Go requires following best practices and adopting a mindset of simplicity and efficiency. By using proper naming conventions, writing short and readable functions, encapsulating and abstracting your code, handling errors effectively, writing clear comments, keeping functions and packages small, utilizing version control and code review, and incorporating unit testing, you'll create high-quality code that is easier to maintain and scale.
Remember, writing clean and maintainable code is an ongoing effort. Continuously improve your coding skills and stay up to date with Go best practices to ensure your codebase remains clean and efficient.
Happy coding!