Working with JSON in Golang: Encoding, Decoding, and Validation
Learn how to work with JSON in Golang. Discover how to encode, decode, and validate JSON data using built-in packages. Master the essential techniques for efficient JSON handling in your Golang applications.
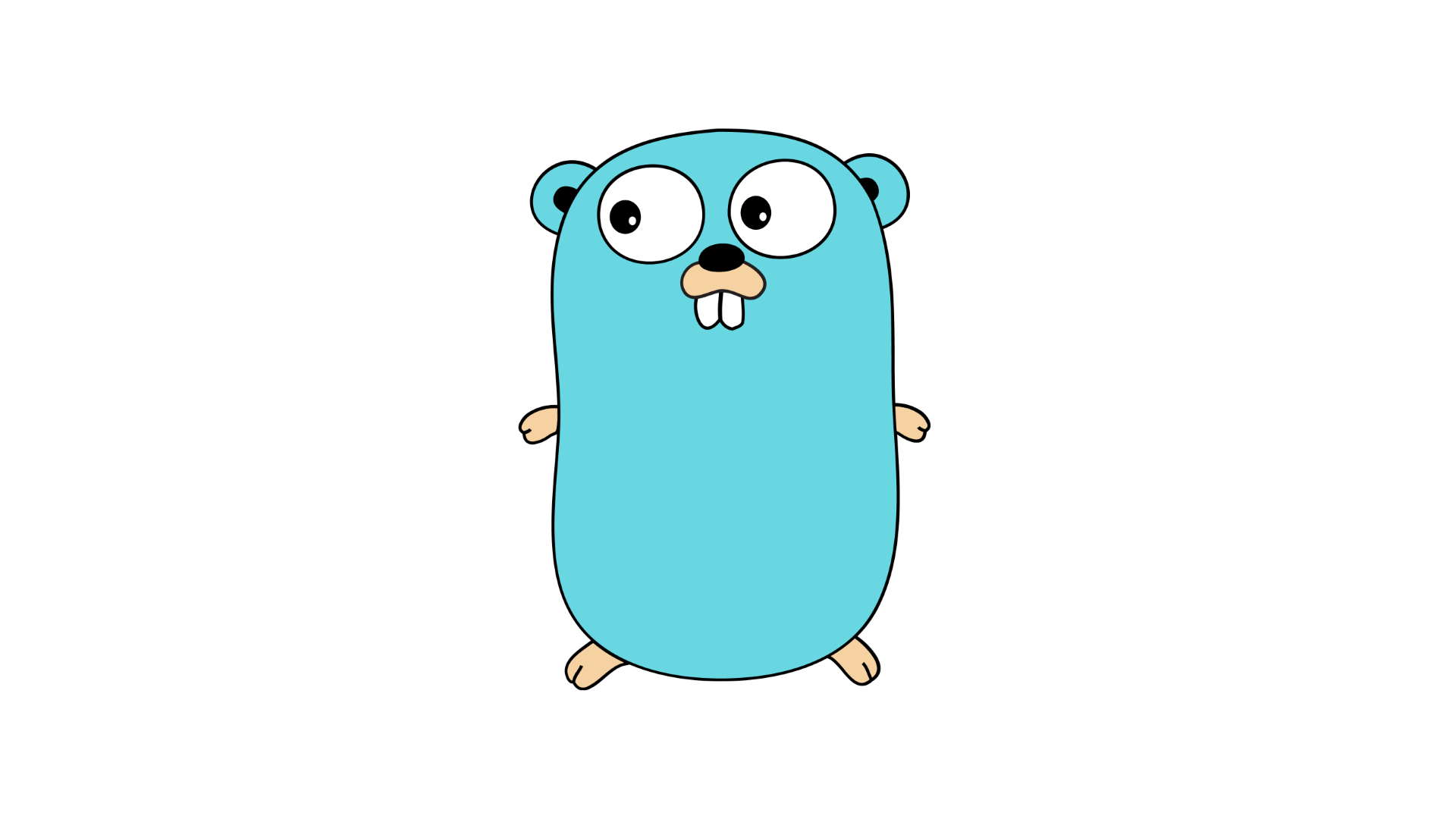
Introduction
Working with JSON (JavaScript Object Notation) in Golang is a common task for developers who need to exchange data between different systems or services. JSON is a lightweight and easy-to-read data format that is widely used in web development and API integrations.
What is JSON?
JSON is a text-based data format that is designed to be human-readable and easy to parse. It originated from JavaScript and has become a popular choice for representing structured data. JSON data is organized in key-value pairs and supports various data types such as strings, numbers, booleans, arrays, and objects.
Why Use JSON in Golang?
Golang, also known as Go, is a programming language developed by Google. It is known for its simplicity, efficiency, and strong support for concurrency. Golang provides built-in support for working with JSON, making it a natural choice for handling JSON data in your applications.
Whether you are building a web application, a microservice, or a command-line tool, you will often need to encode or decode JSON data. Golang provides a set of standard packages that make it easy to work with JSON, including encoding/json and json.Unmarshal.
Encoding JSON in Golang
Encoding JSON in Golang involves converting Go data structures into JSON strings. This is useful when you want to send data as JSON over a network or store it in a file. The encoding/json package provides the necessary functions to encode Go data structures into JSON.
To encode a Go data structure into JSON, you first need to define the structure and populate it with data. Once you have the data, you can use the json.Marshal function to convert it into a JSON string.
Decoding JSON in Golang
Decoding JSON in Golang is the process of converting a JSON string into Go data structures. This is useful when you receive JSON data from an external source, such as an API, and need to work with it in your application. The encoding/json package provides the necessary functions to decode JSON into Go data structures.
To decode a JSON string into Go data structures, you first need to define the structure of the data by creating a Go struct. The struct should have fields that match the keys in the JSON data. You can then use the json.Unmarshal function to decode the JSON string into the Go struct.
Validating JSON in Golang
Validating JSON in Golang involves checking whether a given JSON string conforms to a specific schema or set of rules. This is useful when you want to ensure that the data you receive or process is in the expected format and meets certain criteria.
Golang does not have built-in support for JSON validation, but there are third-party packages available that provide this functionality. One popular package is gojsonschema, which allows you to define a JSON schema and validate JSON data against it.
Summary
In this article, we explored the basics of working with JSON in Golang. We discussed the encoding and decoding of JSON data using the encoding/json package. We also touched upon the topic of JSON validation, highlighting the availability of third-party packages for this purpose.
By mastering the principles of encoding, decoding, and validating JSON in Golang, you'll be able to efficiently exchange data with external systems, build robust APIs, and handle data-driven applications effectively.
Stay tuned for more articles on Golang development and best practices! Happy coding!