Achieving Cross-Platform Interoperability with gRPC
Discover the wonders of gRPC - seamless cross-platform communication between client and server apps, with efficiency, performance, and type safety.
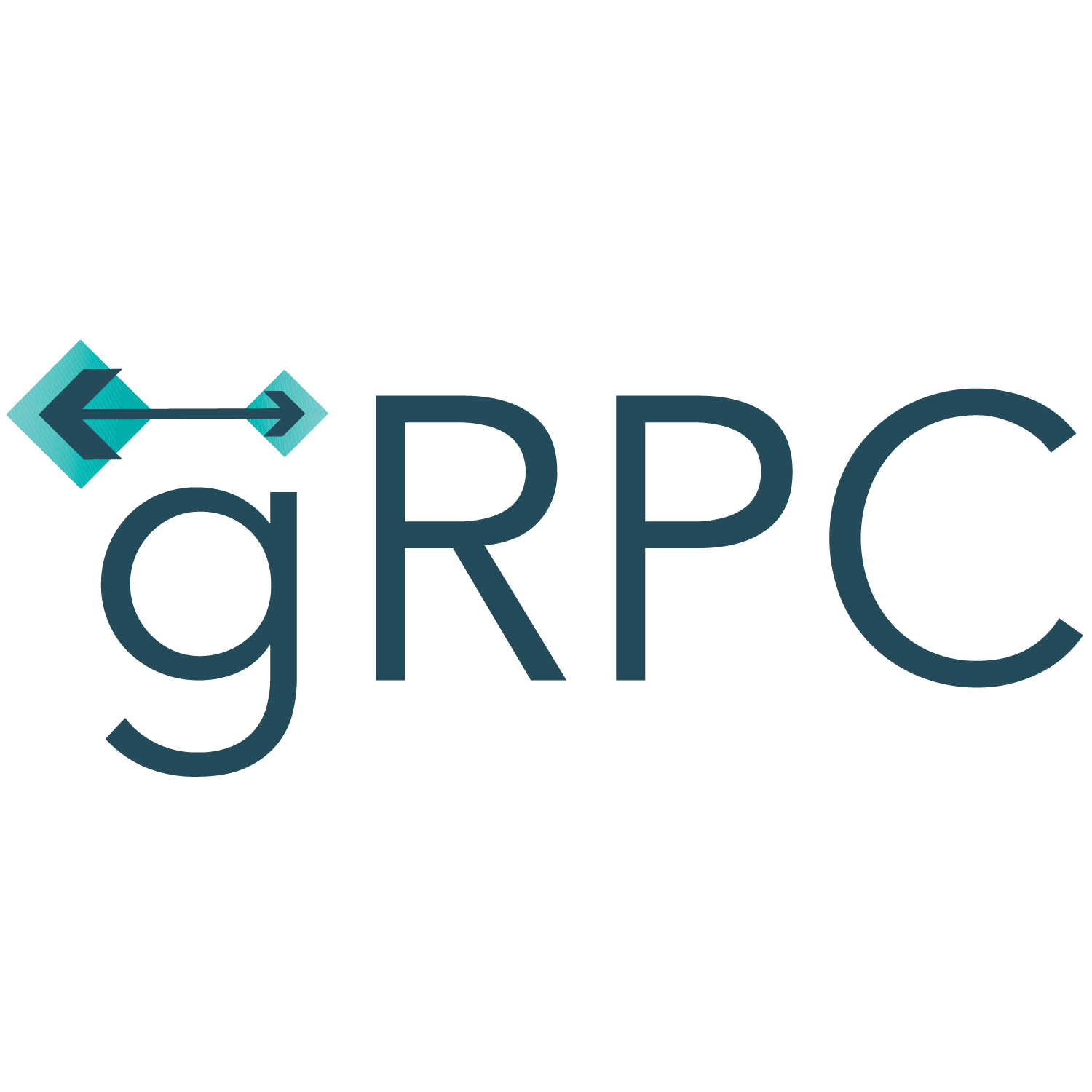
Introduction
As technology evolves and businesses expand globally, achieving cross-platform interoperability is becoming increasingly important. One tool that facilitates seamless communication between different programming languages and platforms is gRPC. In this blog post, we'll explore the power of gRPC and learn how it enables efficient and reliable cross-platform data exchange. Let's dive in and discover the wonders of gRPC!
What is gRPC?
gRPC, short for Google Remote Procedure Call, is an open-source framework that enables cross-platform communication between client and server applications. It uses Protocol Buffers (protobuf) as its interface definition language (IDL) for defining services, messages, and API contracts. With gRPC, you can define and implement efficient and type-safe remote procedure calls, enabling seamless communication between different programming languages and platforms.
How Does gRPC Work?
gRPC employs a client-server architecture to enable remote procedure calls. It uses HTTP/2 as its transport protocol, providing features such as multiplexing, flow control, and header compression. The communication between client and server is done using Protocol Buffers for serializing structured data.
Here's a simplified overview of how gRPC works:
- The service provider defines the service and message types using the Protocol Buffers syntax.
- Using the protobuf compiler, the service provider generates client and server stubs in different programming languages.
- The client application makes remote procedure calls to the server using the generated stub, while the server application implements the service methods defined in the protobuf files.
- gRPC handles all the communication and serialization/deserialization of data between the client and server, making it easy to invoke remote methods and exchange data efficiently.
Advantages of gRPC
gRPC offers several key advantages over other communication frameworks:
- Efficiency: gRPC uses binary serialization with Protocol Buffers, resulting in compact payload sizes and efficient data transfer.
- Performance: gRPC leverages HTTP/2, enabling multiplexing and reducing latency through features like connection multiplexing and server push.
- Language Independence: With gRPC, you can write your client and server application in different programming languages, enabling seamless cross-platform communication.
- Type Safety: gRPC uses Protocol Buffers as its IDL, ensuring type safety during the communication process and reducing the chances of runtime errors.
- Automatic Code Generation: gRPC provides automatic code generation for client and server stubs, reducing boilerplate code and enabling quick integration.
- Bi-directional Streaming: gRPC supports both unary and streaming RPCs, allowing bi-directional streaming of data between client and server applications.
Working with gRPC
Now that we understand the basics of gRPC, let's dive into the key concepts and steps involved in working with gRPC:
1. Defining the Service
The first step in using gRPC is defining the service and message type using Protocol Buffers. The service definition includes the methods that the server will implement and the data structures used for communication.
Here's an example of a simple service definition:
syntax = "proto3";
package myservice;
service MyService {
rpc SayHello (HelloRequest) returns (HelloResponse) {}
}
message HelloRequest {
string name = 1;
}
message HelloResponse {
string message = 1;
}
2. Generating Code
Once the service definition is complete, you can use the protobuf compiler to generate the client and server stubs in your desired programming language. This step automatically generates all the necessary code to handle the communication between the client and server.
For example, to generate code in Python, you can use the following command:
protoc -I=<proto_files_dir> --python_out=<output_dir> <proto_files>
3. Implementing the Server
The next step is implementing the server logic. In the chosen programming language, you'll use the generated server stub to implement the methods defined in the service. These methods will handle the incoming requests and return the appropriate response.
In our example, the server-side code might look like this in Python:
from myservice_pb2 import HelloRequest, HelloResponse
from myservice_pb2_grpc import MyServiceServicer
class MyService(MyServiceServicer):
def SayHello(self, request, context):
return HelloResponse(message=f'Hello, {request.name}!')
server = grpc.server(ThreadPoolExecutor())
myservice_pb2_grpc.add_MyServiceServicer_to_server(MyService(), server)
server.add_insecure_port('[::]:50051')
server.start()
try:
while True:
time.sleep(86400)
except KeyboardInterrupt:
server.stop(0)
4. Calling the Service from the Client
Finally, you can call the service methods from the client application using the generated client stub. The client stub abstracts all the networking details and provides a simple API for invoking the remote methods.
Here's an example of a client calling the `SayHello` method, assuming we are using Python on the client side:
channel = grpc.insecure_channel('localhost:50051')
stub = myservice_pb2_grpc.MyServiceStub(channel)
response = stub.SayHello(myservice_pb2.HelloRequest(name='John'))
print('Server response:', response.message)
Integrating gRPC with Your Project
To integrate gRPC with your project, you'll need to:
- Install the necessary gRPC libraries for your programming language, such as the protobuf compiler for generating code.
- Create the protobuf files for defining your service and message types.
- Generate the client and server stubs using the protobuf compiler.
- Implement the server methods and handle incoming requests.
- Use the client stub to call the remote methods from your client application.
Make sure to follow the official gRPC documentation for your chosen programming language and platform to get started.
Conclusion
gRPC is a powerful tool that enables efficient and reliable cross-platform communication between client and server applications. By leveraging the advantages of gRPC, you can achieve seamless interoperability across different programming languages and platforms, making it easier to develop scalable and distributed systems. So why wait? Start using gRPC in your projects and unlock the full potential of cross-platform communication today!
Thank you for reading this blog post. Stay tuned for more informative articles on gRPC and other exciting topics in the world of software development!