Go by Example: Values
Learn about values in Go and how to declare, initialize, and work with them. Explore examples of changing variables, comparing values, and concatenating strings.
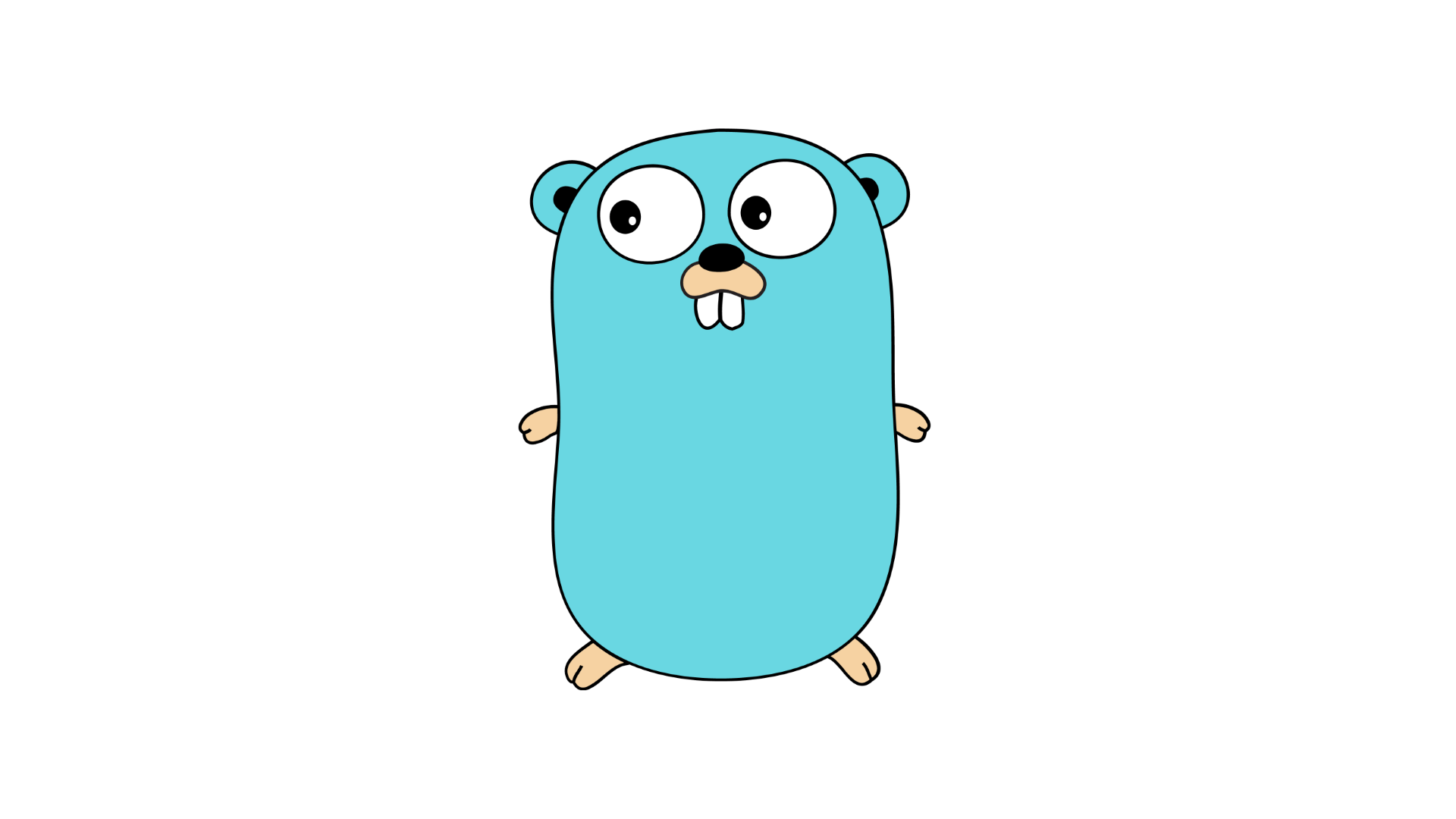
Introduction
Welcome to the first part of our Go by Example series! In this series, we will explore various aspects of the Go programming language by providing examples that demonstrate the language features and concepts. In this article, we will dive into the topic of values in Go.
What are Values?
In Go, a value is a fundamental piece of data that can be stored and manipulated by a program. Values can be of different types, such as numbers, strings, booleans, and more. Understanding how values work in Go is essential for writing efficient and effective code.
Declaring and Initializing Values
In Go, you can declare and initialize values using several different techniques. Let's take a look at some of the common ways to declare and initialize values:
1. var
Keyword
The var
keyword is used to declare variables in Go. You can declare a variable and assign a value to it in a single line using the following syntax:
var name string = "John"
In the above example, we have declared a variable named name
of type string
and assigned the value "John" to it.
You can also declare multiple variables in a single line:
var a, b, c int = 1, 2, 3
In this case, we have declared three variables a
, b
, and c
, all of type int
, and assigned the values 1, 2, and 3, respectively.
2. Short Variable Declaration
Go also provides a shorthand syntax for declaring and initializing variables called short variable declaration. You can use this syntax to declare a variable and assign a value to it without explicitly specifying its type:
name := "John"
In this example, we have declared a variable name
and assigned the value "John" to it. Go automatically determines the type of the variable based on the value assigned to it.
You can also use this syntax to declare and initialize multiple variables in a single line:
a, b, c := 1, 2, "three"
In this case, we have declared three variables a
, b
, and c
, and assigned the values 1, 2, and "three" to them, respectively. Go infers the types of a
and b
as int
, and the type of c
as string
.
Working with Values
Once you have declared and initialized values in Go, you can perform various operations on them. Let's explore some common operations:
1. Changing the Value of a Variable
In Go, you can change the value of a variable by reassigning a new value to it. For example:
var age int = 25
age = 30
In this example, we have declared a variable age
and assigned it the value 25. We then change the value of age
to 30 by reassigning a new value to it. After this assignment, the value of age
will be 30.
2. Comparing Values
In Go, you can compare values using comparison operators such as ==
, !=
, <
, <=
, >
, and >=
. These operators return a boolean value (true
or false
) based on the comparison result. For example:
var a = 10
var b = 20
fmt.Println(a > b) // prints false
In this case, we compare the values of a
and b
using the >
operator. Since a
is not greater than b
, the comparison result is false
.
3. Concatenating Strings
In Go, you can concatenate strings using the +
operator. For example:
var firstName = "John"
var lastName = "Doe"
var fullName = firstName + " " + lastName
fmt.Println(fullName) // prints "John Doe"
In this example, we declare two variables firstName
and lastName
and concatenate their values to create the fullName
variable using the +
operator. The resulting value is "John Doe".
Conclusion
Values are an essential concept in the Go programming language. In this article, we explored how to declare and initialize values, and perform various operations on them. Understanding these concepts will help you write efficient and effective Go code. Stay tuned for the next part of our Go by Example series, where we will explore more exciting aspects of the Go programming language!