Angular Services and Dependency Injection: Simplifying Code Organization
In this blog post, we explore Angular services and dependency injection. Learn how services encapsulate reusable logic and how DI simplifies code organization in Angular applications.
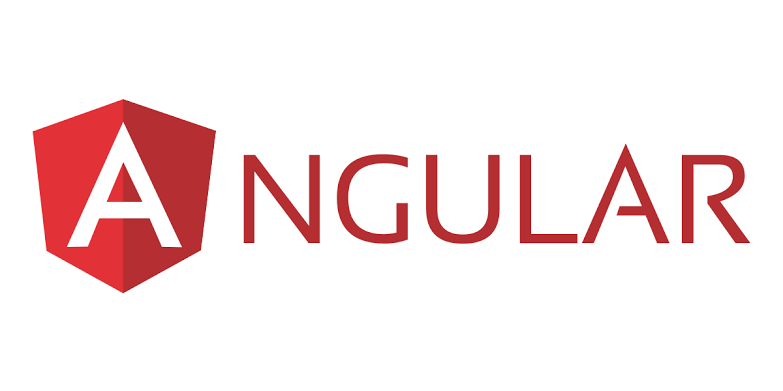
Introduction
When it comes to building Angular applications, code organization is crucial for maintainability and scalability. One of the key aspects of code organization in Angular is the use of services and dependency injection. These powerful concepts allow you to modularize your code, separate concerns, and improve the overall structure of your application.
In this blog post, we will explore the world of Angular services and dependency injection. We'll start by understanding what services are and why they are important. Then, we'll dive into the concept of dependency injection and how it simplifies code organization in Angular applications. By the end of this post, you'll have a solid understanding of how to use services and dependency injection effectively to build better Angular applications.
What are Angular Services?
In Angular, services are the building blocks of your application. They are classes that provide a specific functionality or a set of related functionalities that can be shared across different parts of your application.
Services are used to encapsulate reusable logic, such as data access, API calls, or business logic. By encapsulating this logic in services, you can create independent and modular components that can be easily reused and tested.
An Angular service is typically a singleton, meaning that there is only one instance of the service throughout the application. This ensures that the state and data in the service are shared across different components in a consistent manner.
Why are Angular Services Important?
Angular services are important for several reasons:
- Code Reusability: By encapsulating reusable logic in services, you can easily reuse the same functionality across multiple components.
- Separation of Concerns: Services allow you to separate the business logic from the presentation logic in your components, improving the overall maintainability of your code.
- Dependency Injection: Services play a crucial role in dependency injection, a powerful concept that simplifies code organization and promotes modular development.
Dependency Injection in Angular
Dependency injection (DI) is a design pattern that allows you to inject dependencies into a class instead of creating them within the class itself. In Angular, DI is a built-in feature that simplifies code organization and promotes loose coupling between different parts of your application.
Angular's dependency injection system is based on a hierarchical injector tree. At the root of this tree is the AppModule
, which is responsible for configuring the dependencies for the entire application. Each component or service in your application can have its own dependencies, and Angular's DI system takes care of resolving and injecting these dependencies when needed.
Let's take a closer look at how dependency injection works in Angular:
- Provider: A provider is a configuration object that tells Angular how to create an instance of a dependency. The
AppModule
is responsible for configuring providers for the entire application. - Injector: An injector is responsible for creating instances of dependencies and managing their lifecycle. Angular's DI system maintains a hierarchical tree of injectors, allowing dependencies to be resolved and injected at different levels of the application.
- Inject: The
@Inject
decorator is used to specify a dependency for a class. By using the@Inject
decorator, you inform Angular that the class requires a specific dependency to be injected.
Angular's DI system takes care of creating instances of dependencies and injecting them into the appropriate classes at runtime. This removes the need for manual instantiation of dependencies within your components and services, making your code cleaner and more maintainable.
Using Services and Dependency Injection in Angular Applications
Now that we understand the importance of services and dependency injection in Angular, let's see how to use them in practice.
Creating an Angular Service
To create an Angular service, you can use the @Injectable()
decorator provided by Angular. The @Injectable()
decorator marks the class as a service and allows it to be injected as a dependency in other classes.
Here's an example of a simple Angular service:
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class DataService {
private data: string[] = [];
constructor() { }
addData(item: string) {
this.data.push(item);
}
getData(): string[] {
return this.data;
}
}
Here, we define a service called DataService
that has a private array called data
and two methods: addData()
and getData()
. The addData()
method adds an item to the data
array, and the getData()
method returns the data
array.
Note that we use the @Injectable()
decorator to mark the DataService
class as a service. Additionally, we provide the service at the root level by specifying { providedIn: 'root' }
. This makes the service available to the entire application.
Injecting a Service into a Component
Once you have created a service, you can inject it into a component by adding it as a dependency in the component's constructor:
import { Component } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-my-component',
templateUrl: './my-component.component.html',
styleUrls: ['./my-component.component.css']
})
export class MyComponent {
constructor(private dataService: DataService) { }
}
In this example, we import the DataService
service and include it as a dependency in the MyComponent
component. We use the private
keyword to specify that the dataService
property is private to the component.
Conclusion
Services and dependency injection are essential tools for organizing and structuring your code in Angular applications. By using services, you can encapsulate reusable logic and separate concerns, making your code more maintainable and scalable. Dependency injection simplifies code organization and promotes loose coupling between different parts of your application.
In this blog post, we explored the concepts of services and dependency injection in Angular. We discussed why services are important and how they can be used to encapsulate reusable logic. We also explored the concept of dependency injection and its role in simplifying code organization. By using services and dependency injection effectively, you can build better Angular applications that are easier to develop, test, and maintain.
Thank you for reading! I hope this blog post has been helpful in understanding Angular services and dependency injection. Stay tuned for more articles on Angular development!