Go by Example: Pointers
Understanding pointers is crucial for any Go developer. Pointers enable fine-grained control over memory and data structures, allowing you to modify variables indirectly. Learn the concepts, usage, and best practices of pointers in Go programming.
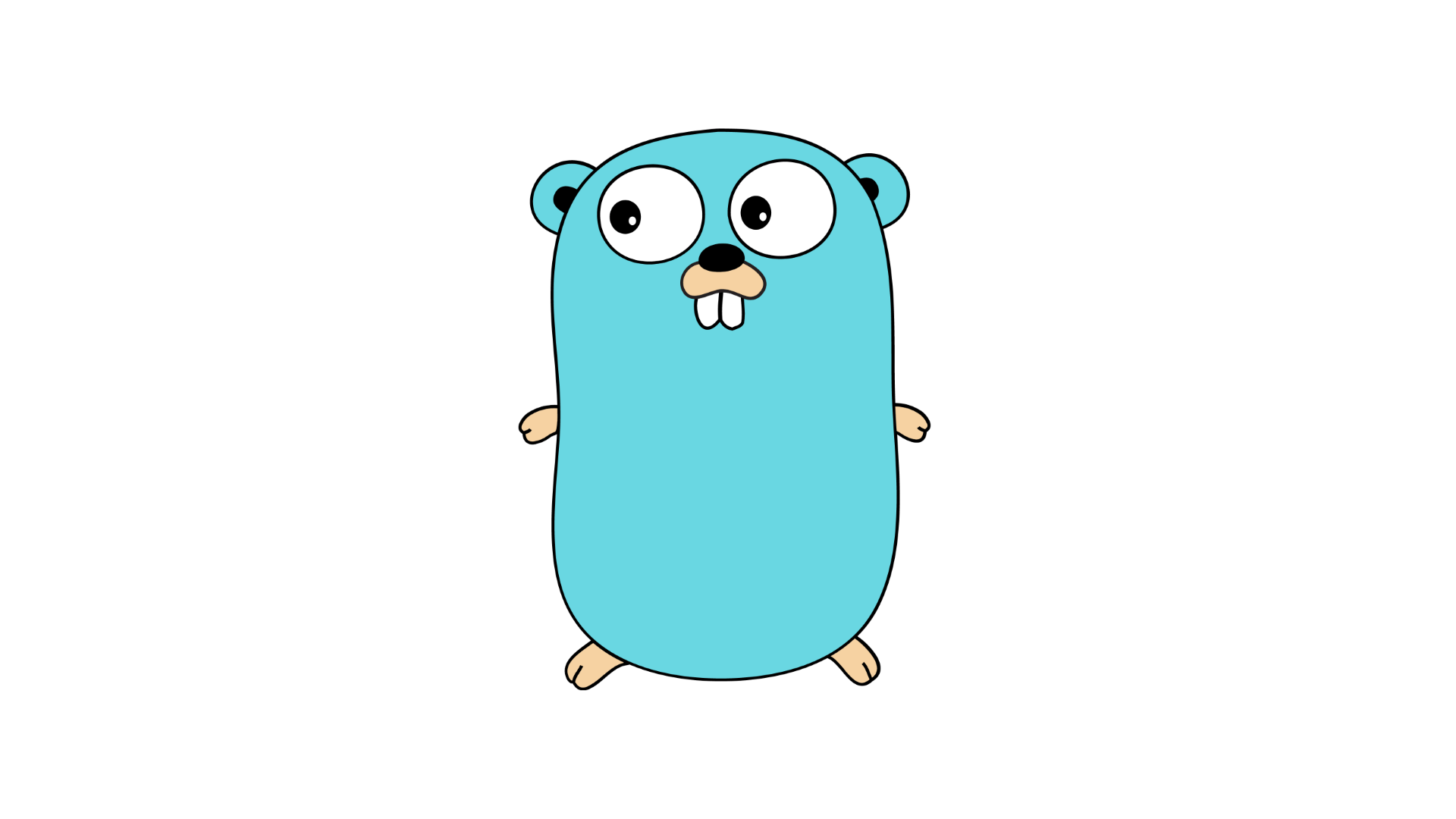
Introduction
Understanding pointers is crucial for any Go developer. Pointers allow you to reference and manipulate data indirectly, giving you fine-grained control over memory and data structures. In this Go by Example tutorial, we'll dive deep into pointers, exploring their concepts, usage, and best practices. By the end of this tutorial, you'll be proficient in working with pointers and harnessing their power in Go programming.
What are Pointers?
In Go, a pointer is a variable that points to the memory address of another variable. Pointers enable you to access and modify the value of the variable indirectly. Instead of working with a variable itself, you work with a memory location that holds the variable's value.
Go uses an asterisk (*) to represent a pointer type. For example, *int
signifies a pointer to an integer, while *string
represents a pointer to a string.
Declaring and Initializing Pointers
To declare a pointer variable in Go, use the asterisk (*) followed by the variable type. Here's an example:
var p *int
This declares a pointer variable p
of type *int
. However, this pointer variable doesn't point to anything yet. To initialize it and make it point to a specific variable, you need to use the address-of operator (&).
var num int = 42
var p *int = &num
In the above code snippet, we declare an integer variable num
with a value of 42. Then, we declare a pointer variable p
of type *int
and initialize it with the address of the num
variable using &num
. Now, p
points to the memory location of num
.
Dereferencing a Pointer
Dereferencing a pointer means accessing the value stored at the memory address pointed to by the pointer. In Go, you can dereference a pointer using the asterisk (*) operator.
var p *int = &num
fmt.Println(*p) // Output: 42
In the above code snippet, *p
dereferences the pointer p
, giving us the value stored at the memory address it points to. Therefore, the output is 42.
Passing Pointers to Functions
One of the primary benefits of pointers is that they enable you to pass variables by reference to functions, rather than by value. This allows you to modify the original variable within the function scope. To pass a pointer to a function in Go, you need to define the function parameter with a pointer type.
func increment(p *int) {
*p++
}
func main() {
var num int = 10
fmt.Println(num) // Output: 10
increment(&num)
fmt.Println(num) // Output: 11
}
In the above code snippet, the increment
function takes a pointer to an integer p
. Inside the function, we dereference the pointer p
using *p
and increment the value it points to. When we call the increment
function with &num
, it modifies the original num
variable.
Nil Pointers
In Go, a nil pointer represents a pointer variable that doesn't point to any memory address. By default, when you declare a pointer without initializing it, it is nil.
var p *int
fmt.Println(p) // Output: nil
In the above code snippet, p
is declared but not initialized. Therefore, it is a nil pointer. When you print a nil pointer, it displays nil
as the output.
Pointers to Structures
Go supports pointers to structures, allowing you to create complex data structures and manipulate them efficiently. When you create a pointer to a structure, you can access and modify the structure fields using the arrow (->) operator.
type Person struct {
Name string
Age int
}
func main() {
var p *Person = &Person{"Alice", 25}
fmt.Println(p.Name) // Output: Alice
fmt.Println(p.Age) // Output; 25
p.Age = 30
fmt.Println(p.Age) // Output: 30
}
In the above code snippet, we define a Person
structure with two fields: Name
and Age
. We then declare a pointer variable p
of type *Person
and initialize it with the address of a new Person
structure. Using the arrow (->
) operator, we can access and modify the structure fields through the pointer.
Pointer to Pointer
In Go, you can have a pointer that points to another pointer. This is known as a pointer to pointer. It is useful in scenarios where you need to change the value of a pointer variable inside a function. To declare a pointer to a pointer, use two consecutive asterisks (**) followed by the variable type.
func main() {
var num int = 42
var p *int = &num
var pp **int = &p
fmt.Println(**pp) // Output: 42
}
In the above code snippet, we have a pointer variable p
that points to the num
variable. Then, we declare a pointer to a pointer variable pp
that points to the p
variable. By dereferencing **pp
, we get the value stored at the memory address that p
points to, which is 42.
Using the new() Function to Create Pointers
In addition to the address-of operator (&
), Go provides the new()
function to allocate memory for a variable and return a pointer to it. The allocated memory is set to the zero value of the variable's type.
func main() {
var p *int = new(int)
fmt.Println(*p) // Output: 0
*p = 42
fmt.Println(*p) // Output: 42
}
In the above code snippet, new(int)
allocates memory for an integer and returns a pointer to it. We assign this pointer to the variable p
and dereference it to access and modify the value stored at the memory address.
Tips and Best Practices
Here are some essential tips and best practices for working with pointers in Go:
- Always initialize a pointer before using it to avoid nil pointer errors.
- Don't pass a pointer to a variable that will go out of scope, as the pointer will become invalid.
- Avoid creating unnecessarily complex structures with excessive pointer indirections, as it can make code harder to understand and maintain.
- Use pointers when you need to modify the original variable, and pass variables by value when you only need to read them.
Conclusion
Pointers are powerful tools in Go programming, offering control, flexibility, and efficiency. In this Go by Example tutorial, we covered the basics of pointers, including their usage, initialization, dereferencing, passing to functions, and advanced concepts like pointers to structures and pointer to pointer. By applying the tips and best practices, you can leverage pointers to optimize your Go programs and write more efficient and maintainable code.
Stay tuned for more Go by Example tutorials, where we'll explore other fundamental concepts and advanced topics in Go programming. Happy coding!