How Does Zero Allocation Work in Golang?
Understanding memory allocation and management is crucial for writing efficient code. In this post, we explore zero allocation in Golang and how it improves performance by minimizing memory usage and overhead.
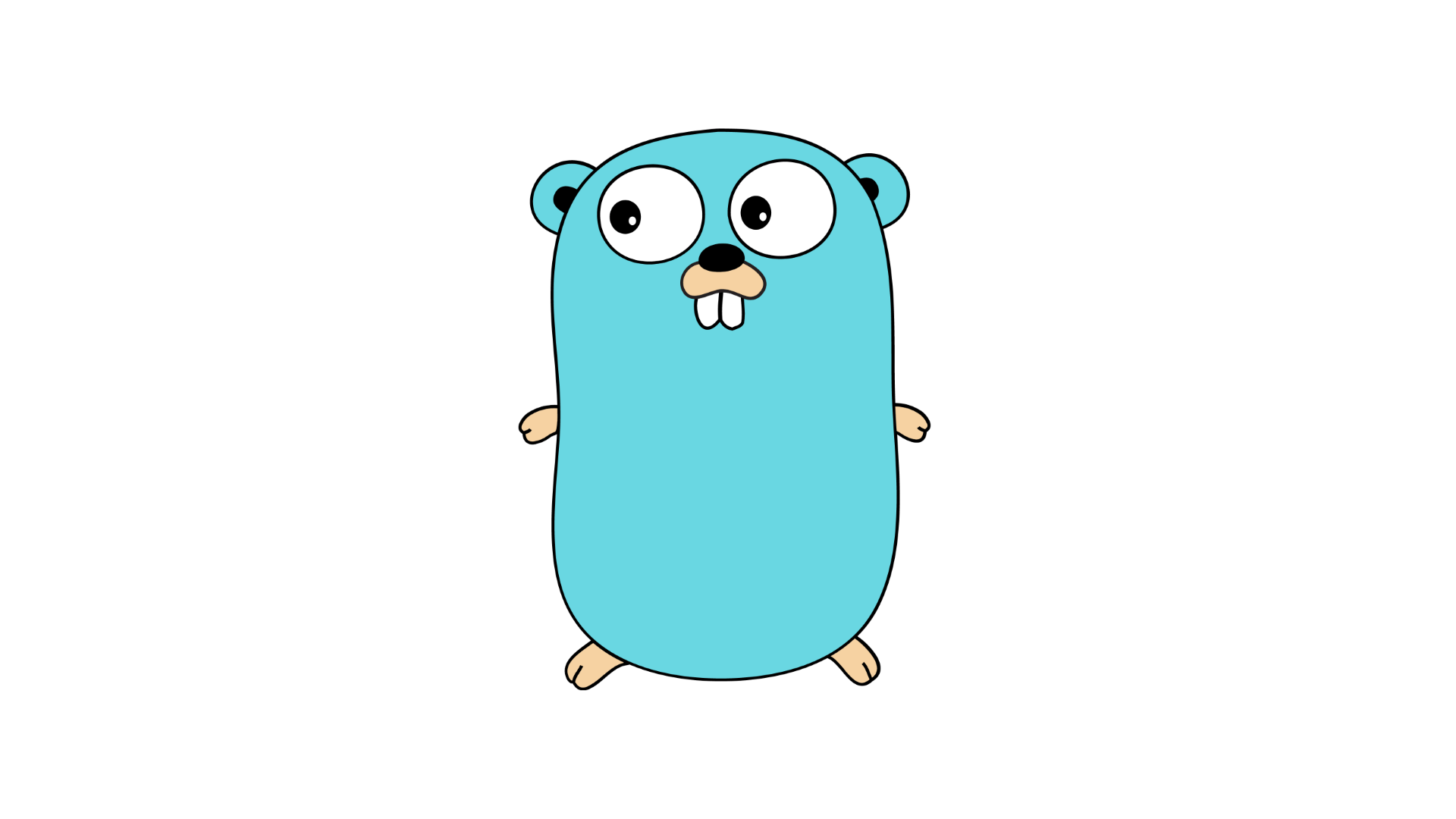
Introduction
Understanding memory allocation and management is crucial for writing efficient and performant code. In the world of Go (Golang), a language known for its speed and scalability, the concept of zero allocation plays a significant role in optimizing memory usage. In this blog post, we'll dive into the intriguing world of zero allocation in Golang and explore how it works.
What is Memory Allocation?
Before we explore zero allocation, let's first understand the concept of memory allocation. In any programming language, memory allocation refers to the process of assigning memory space for variables, data structures, or objects. When you declare a variable or create an object, it needs memory to store its value or state.
In most languages, memory is typically allocated dynamically by the operating system or the programming language's runtime. This process involves requesting memory from the operating system, which then assigns a specific block of memory to the program. Once the program is done using that memory, it can release it back to the operating system for reuse.
What is Zero Allocation?
Zero allocation, as the name suggests, refers to the practice of avoiding memory allocation for certain operations or data structures. In languages like Go, minimizing memory allocation is a fundamental design principle that aims to improve performance, reduce garbage collection overhead, and minimize memory fragmentation.
By reducing memory allocations, Go programs can achieve better efficiency and responsiveness. The Go language provides several mechanisms and techniques to achieve zero allocation or minimize memory allocation as much as possible.
Zero Allocation in Go Slice and Array Operations
Go's slice and array operations provide efficient mechanisms for working with collections of elements. One key advantage of using slices and arrays in Go is their ability to operate without requiring any additional memory allocation in certain scenarios.
Let's take a look at two common operations where zero allocation is achieved:
Appending Elements to a Slice
In many programming languages, when you append an element to an array or a list, the underlying data structure often needs to be resized, involving memory allocation and copying of data. However, Go's slice implementation takes a different approach.
When you append an element to a slice in Go, the runtime checks if the underlying array has enough capacity to accommodate the new element. If there is sufficient capacity, it appends the new element to the slice within the existing array, without allocating new memory.
If the underlying array doesn't have enough capacity, the runtime allocates a new array, copies the existing elements, and appends the new element. However, this reallocation and copying process is done in a smart and efficient way that minimizes memory allocation and overhead.
As a result, Go's slice operations, including appending elements, often achieve zero allocation if the underlying array has enough capacity.
Range Iteration over Slice or Array
Iterating over a slice or an array is a common operation in many programs. In languages like C or Java, this operation typically involves using an index or a pointer to access each element.
However, Go provides a more convenient and efficient way to iterate over a slice or an array using the range
keyword. The range
keyword allows you to loop over the elements of a slice or an array directly, without the need for manual indices or pointers.
Here's an example of iterating over a slice using the range
keyword:
package main
import "fmt"
func main(){
nums := []int{1, 2, 3, 4, 5}
for index, value := range nums {
fmt.Println(index, value)
}
}
When you use the range
keyword, Go allocates memory internally for the index and the value. However, this memory allocation is hidden from the user and doesn't impact the overall performance significantly. The allocation for the index and value is optimized and reused across iterations, resulting in minimal memory allocation.
Sync.Pool for Object Reuse
Go provides the sync.Pool
package, which allows you to reuse allocated objects instead of creating new ones. The sync.Pool
is a built-in mechanism to store and retrieve temporary objects for efficient memory management.
The sync.Pool
package uses a pool of objects, pre-allocated and ready for use. When your program needs an object, it can request one from the pool. If the pool has a free object available, the pool returns it. Otherwise, it creates a new object and returns it.
Here's an example of using the sync.Pool
package to minimize object allocation:
package main
import (
"fmt"
"sync"
)
type Object struct {
value string
}
func main() {
pool := sync.Pool{
New: func() interface{} {
return &Object{value: "default value"}
},
}
obj1 := pool.Get().(*Object)
fmt.Println(obj1.value)
obj1.value = "new value"
pool.Put(obj1)
obj2 := pool.Get().(*Object)
fmt.Println(obj2.value)
pool.Put(obj2)
}
In this example, instead of creating a new object every time, we use the sync.Pool
to store and re-use the objects. This avoids unnecessary memory allocations and improves performance by reusing objects.
Conclusion
Zero allocation is a critical aspect of writing performant and efficient code in Go. By minimizing memory allocations, you can optimize performance, reduce garbage collection overhead, and enhance the overall responsiveness of your applications.
In this blog post, we explored how zero allocation works in Golang, focusing on slice and array operations, as well as the sync.Pool
package for object reuse. Understanding these concepts and applying them in your code can help you write more efficient and memory-friendly Go programs.
Keep exploring and experimenting with zero allocation techniques in Go, and you'll continue to improve your code's performance and efficiency.