Understanding Golang's Memory Management: Pointers and Garbage Collection
Get a deeper understanding of memory management in Go, including pointers and garbage collection. Learn how Go handles memory allocation and deallocation automatically.
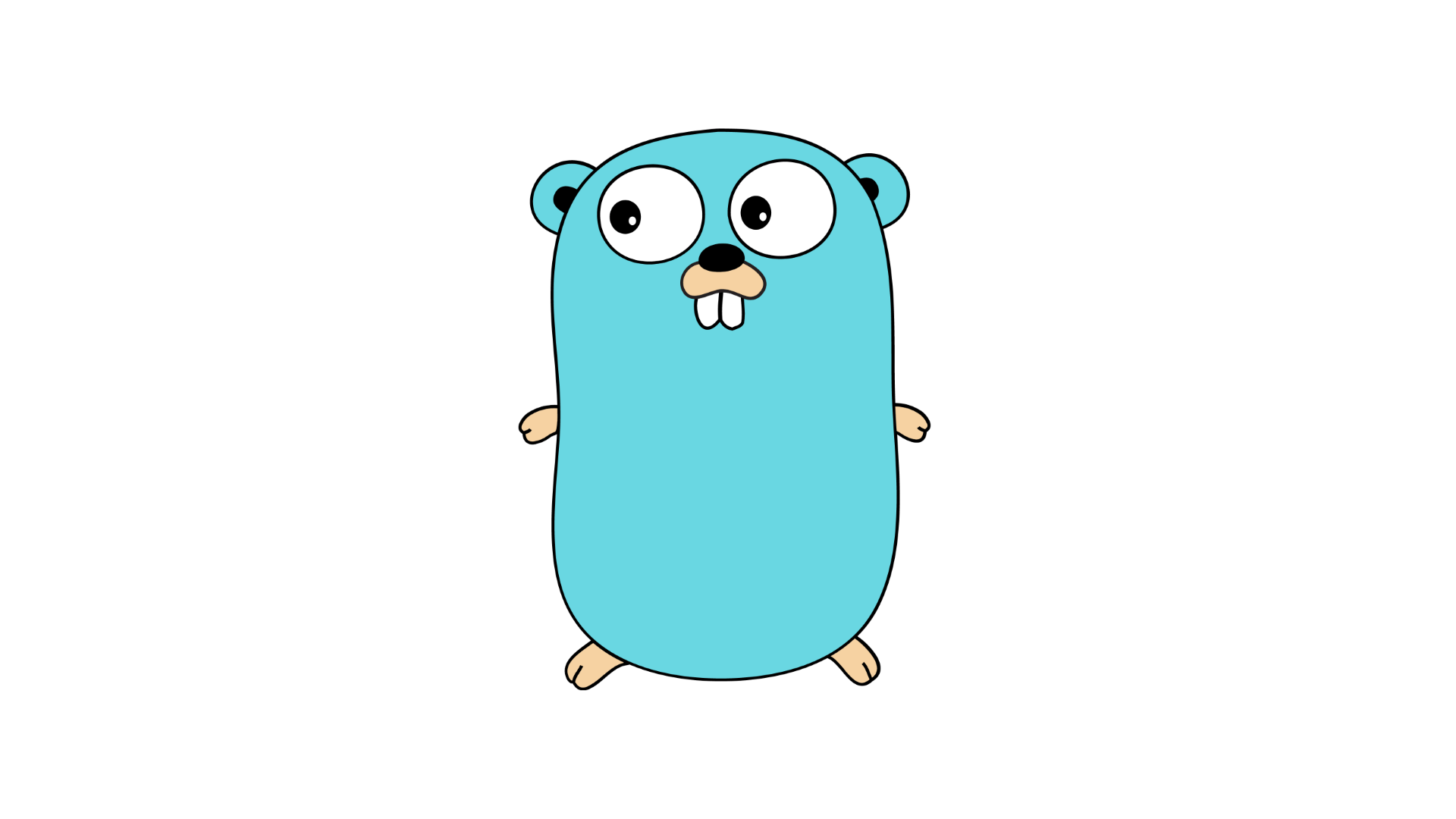
Introduction
Golang, or Go, is a popular programming language known for its simplicity, efficiency, and strong support for concurrency. One of the key aspects of Go that sets it apart from other programming languages is its memory management system. In this article, we will explore Go's memory management, focusing specifically on pointers and garbage collection.
Pointers in Go
In Go, a pointer is a variable that stores the memory address of another variable. Unlike some languages that allow direct manipulation of memory addresses, Go uses pointers in a safer and controlled manner.
Pointers in Go are defined using the asterisk (*) symbol followed by the type of the variable being pointed to. For example, to declare a pointer to an integer variable, we would write:
var ptr *int
When we have a variable and we want to obtain its memory address, we can use the ampersand (&) symbol. Here's an example:
var num int = 42
var ptr *int = &num
In this example, ptr
is a pointer to the variable num
, and it holds the memory address of num
.
Garbage Collection in Go
Garbage collection is a vital process in any programming language that dynamically allocates memory. It helps reclaim memory that is no longer in use, preventing memory leaks and ensuring efficient memory usage.
Go has a built-in garbage collector that automatically manages memory for us. It uses a technique called mark and sweep, which works as follows:
- Every time memory is allocated, the garbage collector marks it as in-use.
- Periodically, the garbage collector sweeps through the memory and identifies memory blocks that are not marked. These unmarked blocks are then considered garbage and are freed.
The garbage collector in Go handles memory management in the background, without requiring explicit intervention from the developer. This automatic memory management mechanism greatly simplifies memory management and eliminates the possibility of memory leaks and dangling pointers.
Understanding Pointers and Garbage Collection in Go
Now that we have a clear understanding of pointers and garbage collection in Go, let's explore how they work together.
When a pointer points to an object, the garbage collector recognizes that the object is still in use and should not be garbage collected. It ensures that the memory allocated to the object remains intact as long as there are active references to it through pointers.
However, if a pointer no longer references an object, the garbage collector detects that the object is no longer being used and marks it as available for garbage collection. The next time the garbage collector runs, it will sweep through the memory and free the memory occupied by the unreferenced object.
Go's garbage collector handles the complexities of managing memory with pointers, allowing developers to focus on writing code without worrying about memory leaks or the intricacies of manual memory management.
Conclusion
Understanding memory management in Go is crucial for writing efficient and reliable code. Pointers and garbage collection are key components of Go's memory management system that ensure safe, automatic, and efficient memory allocation and deallocation.
By grasping the concepts of pointers and garbage collection in Go, you will be better equipped to write high-performance and scalable applications. So, keep practicing and exploring the power of memory management in Go!