Go by Example: Closures
Learn how to use closures in Go to create functions that have access to variables defined in their parent scope. Closures provide flexibility and can be used for abstraction, capturing state, and function factories.
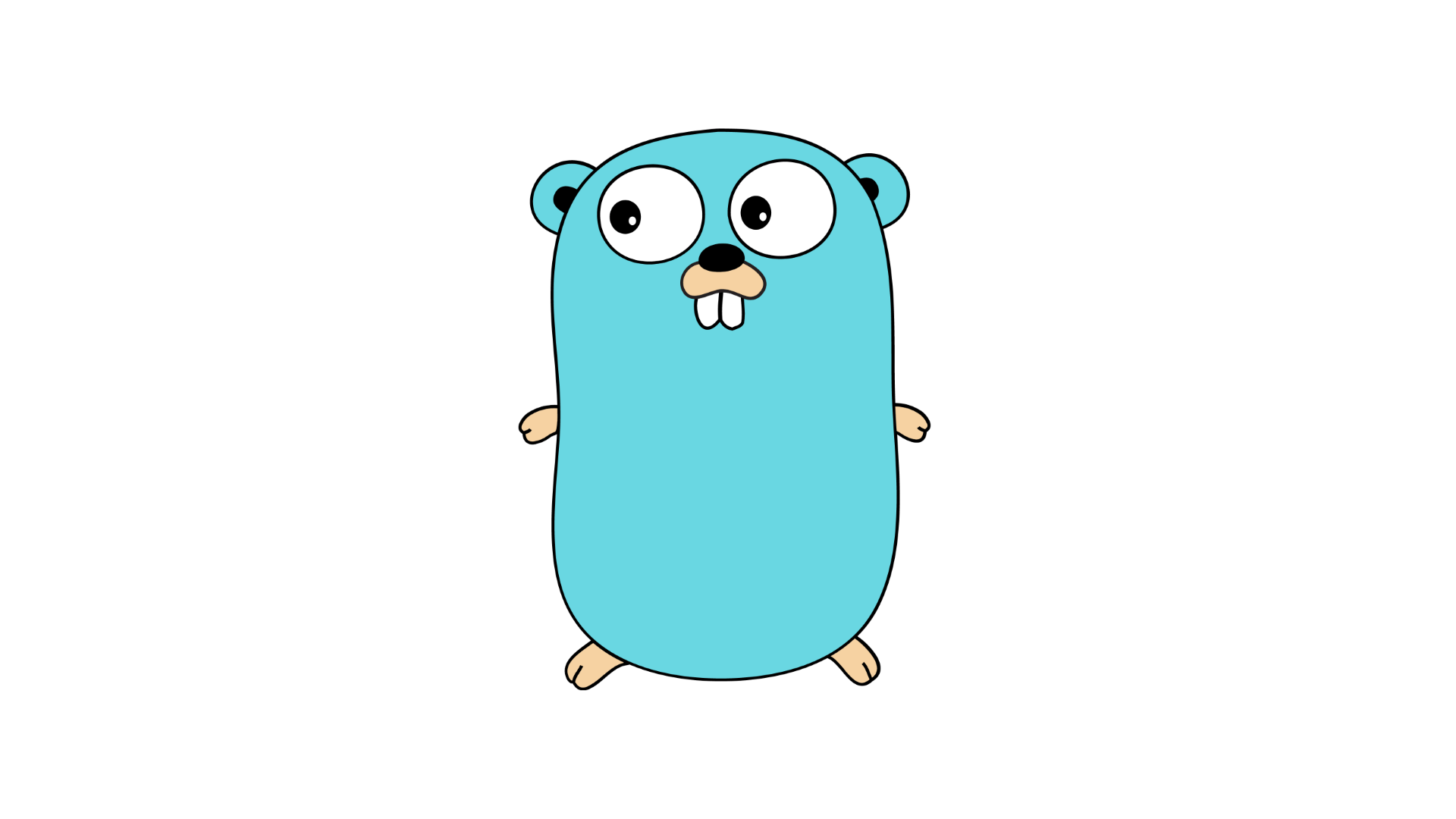
Introduction
Welcome to the Go by Example series! In this edition, we are going to explore the concept of closures in Go. Closures are a powerful feature of Go that allow you to create functions that have access to variables defined in their parent scope. By understanding closures, you'll be able to write more flexible and expressive code. So let's dive in and learn how to use closures in Go!
Understanding Closures
Before we delve into the code, let's understand what closures are and why they are useful.
A closure is a function value that references variables from outside its body. In simpler terms, a closure is a function bundled together with its surrounding state (the variables that it has access to). This allows the function to remember and access those variables even when it is called outside of its original scope.
Closures are often used to create anonymous functions (functions without a name) that have access to variables defined outside of the function body. This makes closures incredibly powerful as they allow you to create functions that are aware of their execution context.
Go Closures Example
Let's dive into some code examples to understand how closures work in Go.
package main
import "fmt"
func createCounter() func() int {
count := 0
return func() int {
count++
return count
}
}
func main() {
counter := createCounter()
fmt.Println(counter()) // Output: 1
fmt.Println(counter()) // Output: 2
fmt.Println(counter()) // Output: 3
}
In this example, we define a function createCounter
that returns another function. The returned function is a closure that has access to the count
variable defined in the createCounter
function.
Inside the closure, we increment the count
variable and return its value. Each time the closure is called, it remembers the state of the count
variable due to closure's ability to remember the variables from the outer scope.
When we run the main
function, we create a new closure using the createCounter
function and assign it to the counter
variable. We can then call the closure like a regular function and see that it returns the incremented count each time it is called.
Practical Uses of Closures
Closures can be used in a variety of scenarios to enhance the flexibility and power of your code. Let's explore a few practical use cases:
1. Providing Abstraction
Closures can be used to abstract away complex logic and provide a more simplified interface. By encapsulating the internal state and implementation details within a closure, you can expose a cleaner and more intuitive API to the user.
2. Capturing State
Closures are especially useful when dealing with asynchronous programming or callback functions. They allow you to capture and preserve the current state of your program, making it easier to handle callbacks and retain the context in which they were created.
3. Function Factories
Closures can also be used to create factories for generating functions with customized behavior. By defining a closure that accepts parameters, you can easily generate and return functions with specific configurations.
Conclusion
Closures are a powerful feature in Go that allow you to create functions with access to variables from their parent scope. They provide a flexible and expressive way to write code that is aware of its execution context. Understanding closures will help you write more efficient and elegant code in Go.
I hope this tutorial helped you understand the concept of closures in Go. Stay tuned for the next installment of the Go by Example series!