Testing and Debugging in Golang: Tools and Techniques
Master the tools and techniques for testing and debugging in Go (Golang). Learn unit testing, print statements, GDB, Delve, profiling, and logs to ensure reliable code.
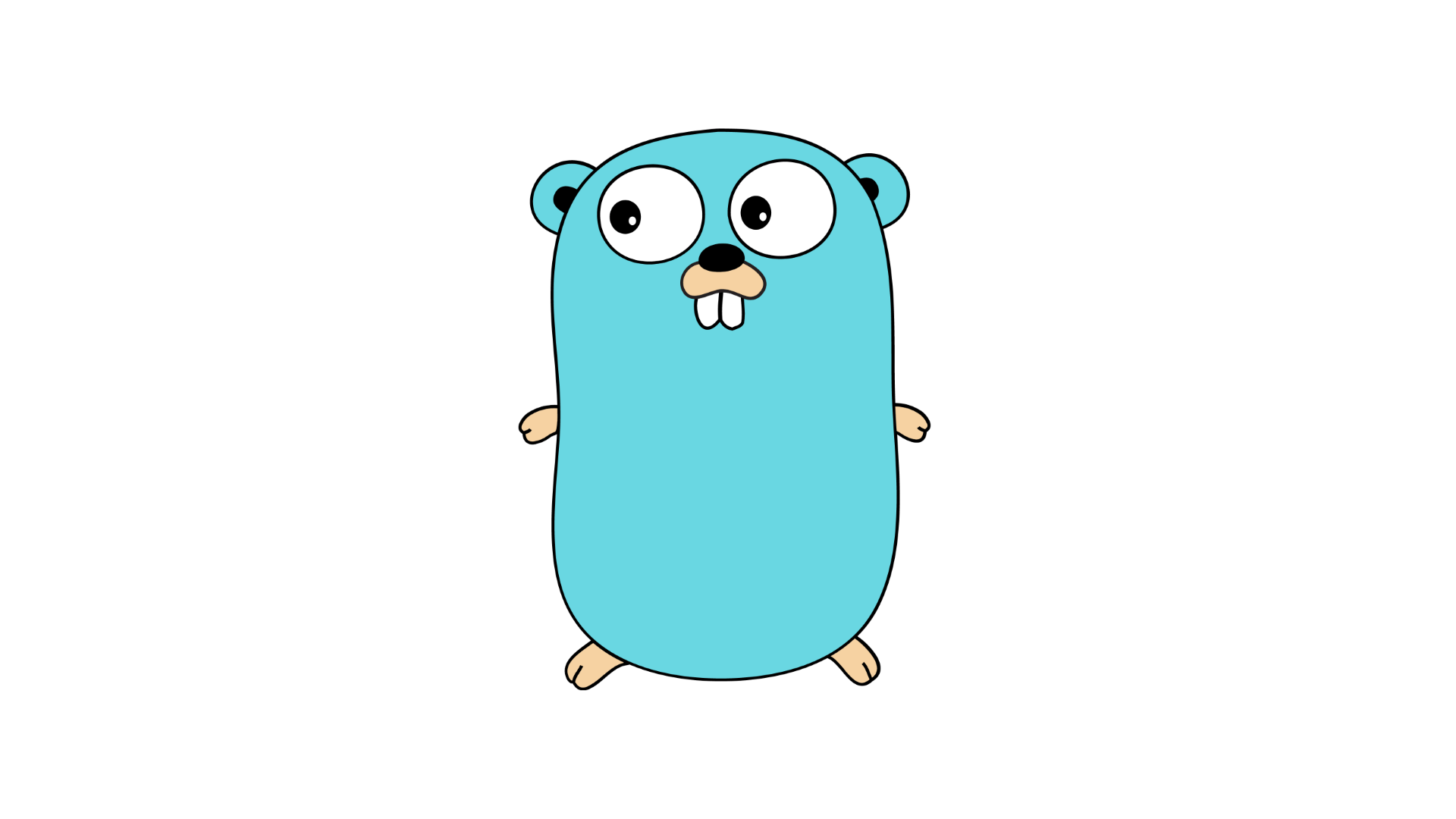
Introduction
Testing and debugging are crucial aspects of software development in any programming language, and Go (Golang) is no exception. In this section, we will explore the tools and techniques available in Go for testing and debugging your code. By mastering these tools and techniques, you'll be able to catch bugs early, ensure the reliability of your code, and optimize your development process.
Unit Testing in Go
Unit testing is an essential practice in software development as it allows you to verify the correctness of individual units, or functions, within your code. Go provides a built-in testing package, "testing," which makes unit testing straightforward and efficient. With the help of this package, you can write tests for your functions, execute them, and view the results.
Writing Unit Tests
To write unit tests in Go, create a separate file with "_test" as the suffix in the file name. For example, if you have a file named "math.go" that contains your math-related functions, you will write the corresponding unit tests in a file named "math_test.go."
In the test file, import the "testing" package and create functions whose names start with "Test." For instance, if you have a function named "Add" in your "math.go" file, you can write a test function named "TestAdd" in your test file.
Within the test function, use the "t" parameter of type "testing.T" to call the various testing functions provided by Go. These functions allow you to check if the results of your functions match the expected values using assertions such as "t.Run," "t.Errorf," and "t.Fail."
Running Unit Tests
To run your unit tests in Go, navigate to the root directory of your project in the terminal and execute the command:
go test
This command will automatically search for files ending in "_test.go" and run the corresponding tests. You will see the test results, including any failures or errors, in the terminal output.
Debugging in Go
Debugging allows you to identify and resolve issues in your code by examining its behavior at runtime. Go provides various tools and techniques to help you debug your applications effectively.
Print Statements
One of the simplest and most effective debugging techniques in Go is using print statements. By strategically placing print statements in your code, you can output variables' values or log messages to the console at specific points in your program's execution. This approach allows you to observe the flow of your program and identify any unexpected behavior.
GDB
GDB, the GNU Debugger, is a powerful command-line tool that enables you to debug Go applications at a lower level. You can use GDB to set breakpoints, step through code execution, inspect variables, and analyze stack traces. By running your program with GDB, you have more control and visibility into its inner workings. To use GDB with Go, you need the Delve debugger, which is discussed in the next section.
Delve
Delve is a popular debugger and development tool for Go. It provides a more user-friendly and feature-rich debugging experience compared to GDB. Delve allows you to set breakpoints, examine variables and memory, step through code, and more. It integrates well with popular code editors like Visual Studio Code, making it ideal for debugging Go applications in modern development environments.
Profiling
Profiling is the process of analyzing the performance of your code to identify bottlenecks and optimize its execution. Go provides built-in profiling tools that allow you to measure the CPU, memory, and block profiling of your applications. By profiling your code, you can pinpoint areas that consume excessive resources and make necessary optimizations, resulting in faster and more efficient programs.
Writing Logs
Logging is another useful technique for debugging your Go code. By logging relevant information, such as variable values, function calls, and error messages, you can track and analyze the behavior of your application over time. Go provides a built-in "log" package that makes logging straightforward and configurable.
Conclusion
Testing and debugging are vital for developing robust and reliable Go applications. By leveraging the testing package, writing effective unit tests becomes a breeze. Moreover, using tools like print statements, GDB, Delve, and profiling, you can locate and fix bugs efficiently. Don't forget the importance of logging, as it allows you to have visibility into your application's behavior. With these tools and techniques at your disposal, you will be well equipped to ensure the quality and stability of your Go code.
In the next section, we will explore advanced testing strategies and best practices to take your Go testing skills to the next level. Stay tuned!