Testing Techniques for Domain-Driven Design in Golang Applications
"Testing DDD in Golang is crucial for ensuring correctness and maintainability. Learn unit testing for aggregates and entities, integration testing for bounded contexts, and database testing with go-sqlmock."
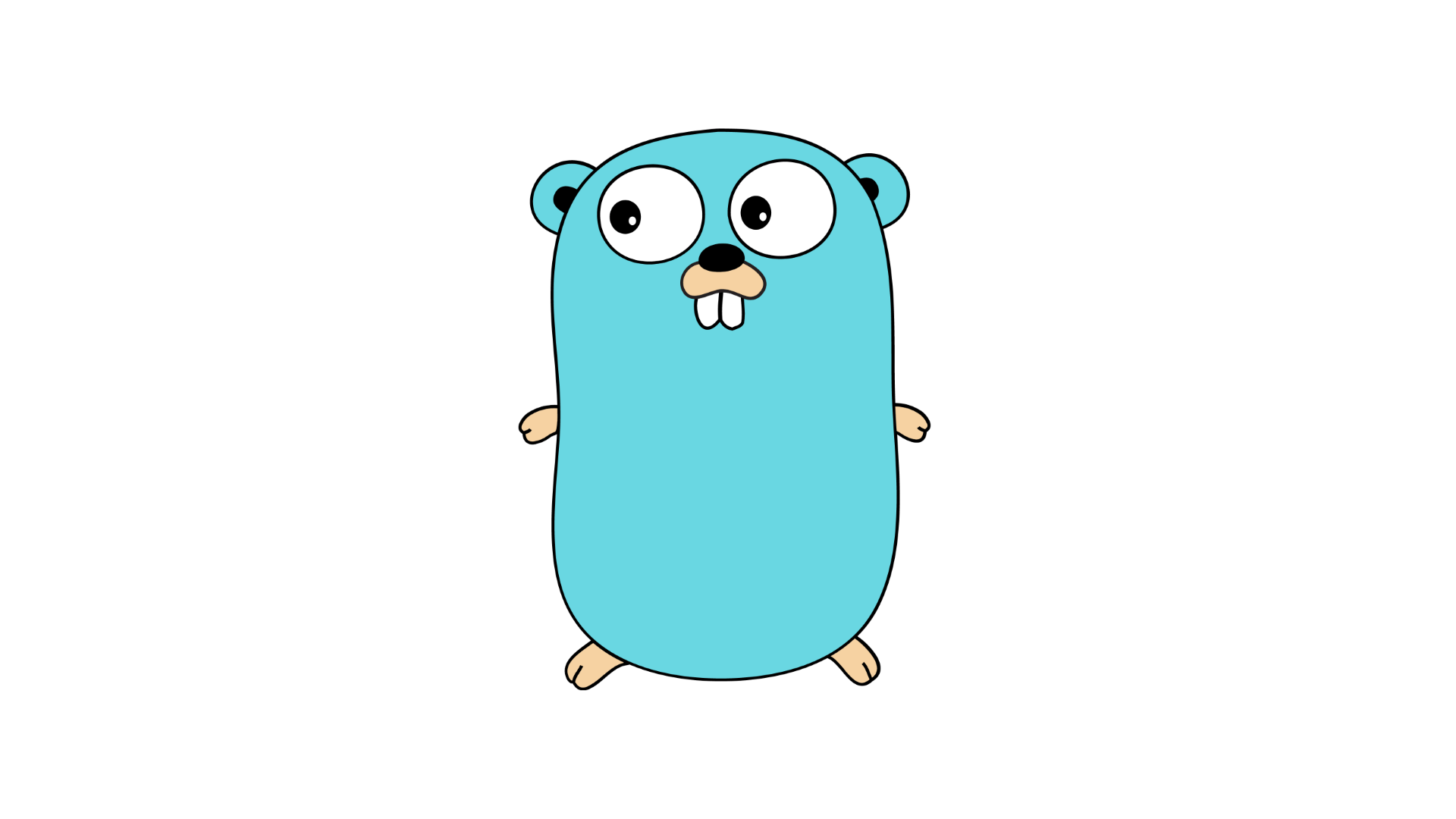
Introduction
Domain-Driven Design (DDD) is a powerful methodology for building complex applications with clean and maintainable code. As a developer, it's crucial to ensure that your DDD-based Go (Golang) applications are thoroughly tested to verify their correctness and robustness. In this blog post, we'll explore various testing techniques that can be applied to Golang applications following the principles of Domain-Driven Design.
Why Testing DDD in Golang?
Testing DDD in Golang has numerous benefits. It helps in:
- Ensuring that business logic is correctly implemented
- Validating interactions between different modules and bounded contexts
- Identifying and fixing errors early in the development process
- Maintaining high code quality and reducing technical debt
Testing Techniques for DDD in Golang
1. Unit Testing for Aggregates and Entities
Aggregates and entities form the core building blocks of a DDD-based application. Unit testing is essential to ensure their functionality and behavior.
In Golang, you can use the built-in testing package (testing
) along with go test
to write unit tests for your aggregates and entities. Let's consider an example of testing an aggregate:
package main
import (
"testing"
)
func TestOrder_Accept(t *testing.T) {
order := NewOrder() // Initialize Order entity
order.Accept() // Invoke Accept method
if !order.IsAccepted() {
t.Errorf("Order was not accepted")
}
}
This unit test ensures that the Accept
method of the Order
aggregate updates its IsAccepted
flag correctly. Similarly, you can write unit tests for other methods and properties of your aggregates and entities.
2. Integration Testing for Bounded Contexts
Bounded contexts represent different modules or components of your DDD application. Integration testing is crucial to validate interactions and data flow between these bounded contexts.
Golang provides excellent support for integration testing through its standard library and the flexibility to use external testing libraries. Let's see an example of integration testing using Golang's httptest
package:
func TestAPI_withData(t *testing.T) {
// Set up a test server
server := httptest.NewServer(api.Handlers())
defer server.Close()
// Send a request to the test server
resp, err := http.Get(server.URL + "/api/books")
if err != nil {
t.Fatalf("Failed to send request: %v", err)
}
defer resp.Body.Close()
// Assert the response status code
if resp.StatusCode != http.StatusOK {
t.Errorf("Expected status %d; got %d", http.StatusOK, resp.StatusCode)
}
// Parse and assert the response body
var books []models.Book
if err := json.NewDecoder(resp.Body).Decode(&books); err != nil {
t.Fatalf("Failed to parse response: %v", err)
}
if len(books) != 3 {
t.Errorf("Expected 3 books; got %d", len(books))
}
}
This integration test ensures that the API route "/api/books" returns the expected data. You can extend this example to cover other endpoints and test different interactions between your bounded contexts.
3. Database Testing with go-sqlmock
When working with databases in DDD applications, it's vital to test the interactions between your code and database without relying on an actual database connection. The go-sqlmock
library provides powerful capabilities for writing database tests in Golang.
Let's see an example of a database test that uses go-sqlmock
:
func TestRepository_GetByID(t *testing.T) {
db, mock := sqlmock.New()
defer db.Close()
repo := NewRepository(db)
id := "123"
rows := sqlmock.NewRows([]string{"id", "name"}).AddRow(id, "Book 1")
mock.ExpectQuery("SELECT (.+) FROM books").WithArgs(id).WillReturnRows(rows)
book, err := repo.GetByID(id)
if err != nil {
t.Errorf("Failed to get book by ID: %v", err)
}
if book.ID != id || book.Name != "Book 1" {
t.Errorf("Unexpected book data: %+v", book)
}
}
This test validates that the GetByID
method of a repository fetches the correct data from the database. By utilizing go-sqlmock
, you can simulate various database scenarios and ensure the correctness of your database interactions.
Conclusion
Testing your Domain-Driven Design applications in Golang is crucial to ensuring their correctness, robustness, and maintainability. By applying the testing techniques discussed in this blog post, you'll be able to validate your application's aggregates, entities, bounded contexts, and database interactions. Remember, comprehensive testing is key to building reliable and scalable DDD applications in Golang.