Simplifying Microservices Communication with gRPC Gateway
Discover how gRPC Gateway simplifies microservice communication by generating a reverse proxy server that translates RESTful JSON API requests into Protocol Buffers. Leverage the benefits of gRPC with a RESTful interface. Build scalable and efficient microservices architectures.
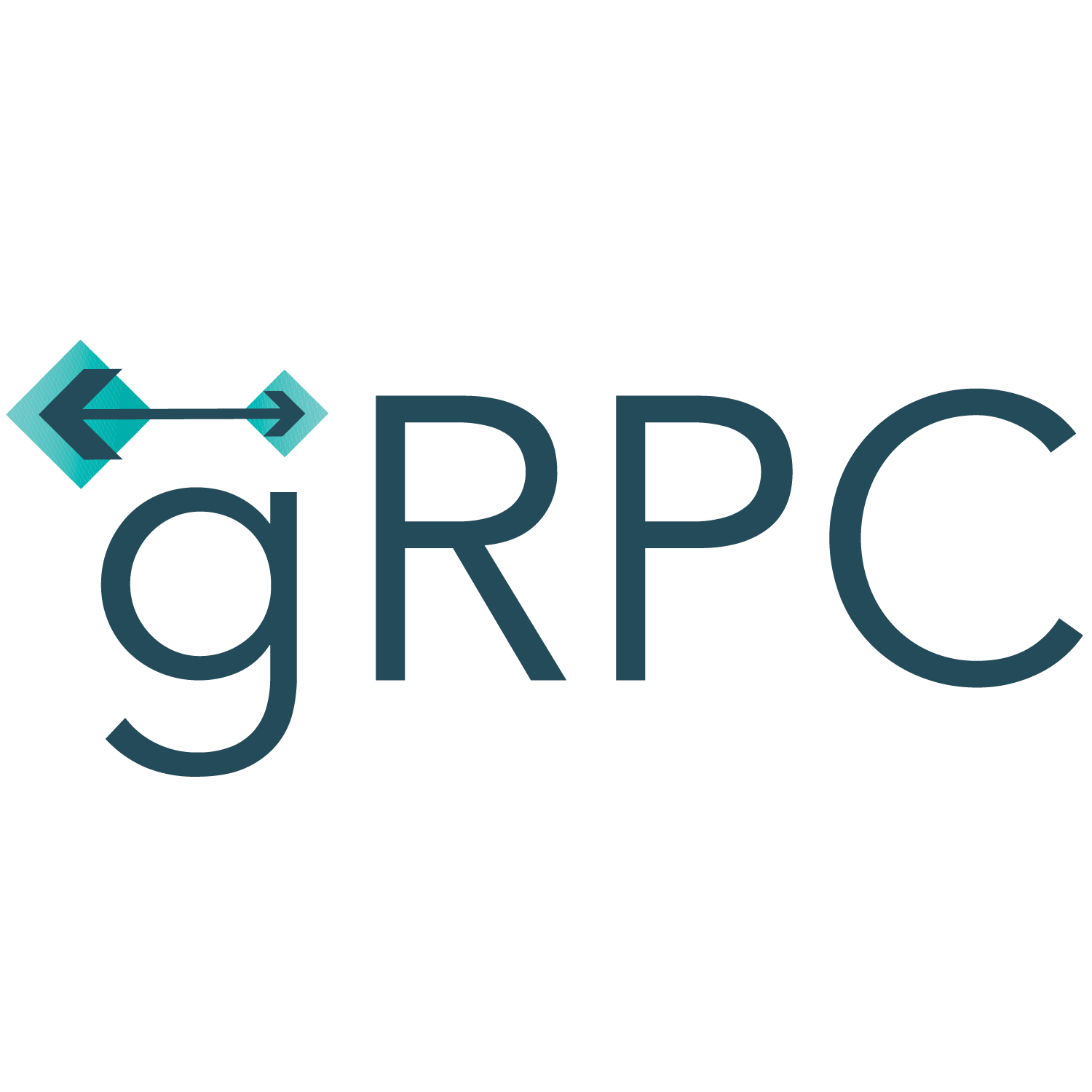
Introduction
Microservices architecture has gained significant popularity in recent years due to its ability to build large and complex applications using small and independent services. One of the critical challenges in a microservices architecture is orchestrating the communication between these services. Traditionally, services communicate with each other using RESTful APIs, but this approach can become cumbersome and inefficient as the number of services increases.
In this blog post, we will explore a powerful open-source tool called gRPC Gateway that simplifies microservices communication. gRPC Gateway allows you to generate a reverse proxy server that translates a RESTful JSON API into Protocol Buffers, the language-agnostic binary serialization format used by gRPC. This allows you to leverage the benefits of gRPC, such as strong typing, bi-directional streaming, and efficient serialization, while still providing a RESTful interface for your clients.
Prerequisites
Before we dive into using gRPC Gateway, make sure you have the following:
- A basic understanding of microservices architecture
- A working knowledge of gRPC and Protocol Buffers
- Go installed on your machine
Setting Up Your Environment
The first step is to set up your development environment. Follow these steps to install the necessary tools:
- Install Protocol Buffers: Download and install the Protocol Buffers compiler from the official website based on your operating system.
- Install gRPC: Open your terminal and run the following command to install gRPC:
go get google.golang.org/grpc
- Install gRPC Gateway: Run the following command to install gRPC Gateway:
go get github.com/grpc-ecosystem/grpc-gateway/v2/protoc-gen-grpc-gateway
go get github.com/grpc-ecosystem/grpc-gateway/v2/protoc-gen-openapiv2
Defining Your API
The next step is to define your gRPC service and generate the necessary protobuf files. To make things easier, let's assume you already have a gRPC service defined. If not, you can refer to the official gRPC documentation to learn how to define your service.
Once you have defined your gRPC service, you need to generate the protobuf files using the protoc
compiler. Run the following command to generate the necessary files:
protoc --proto_path=<path/to/your/protos> --go_out=<output/path> --go-grpc_out=<output/path> --grpc-gateway_out=<output/path> your_service.proto
This command will generate the necessary Go code and protobuf files for your gRPC service, as well as the necessary files for gRPC Gateway.
Generating the Reverse Proxy
Now that you have your protobuf files and the necessary Go code, it's time to generate the reverse proxy server using gRPC Gateway. Follow these steps:
- Create a new file called
gateway.go
in your project directory:
package main
import (
"context"
"flag"
"log"
"net/http"
"github.com/grpc-ecosystem/grpc-gateway/v2/runtime"
"google.golang.org/grpc"
"your_project/your_package"
)
var (
grpcServerEndpoint = flag.String("grpc-server-endpoint", "localhost:50051", "gRPC server endpoint")
)
func run() error {
ctx := context.Background()
ctx, cancel := context.WithCancel(ctx)
defer cancel()
mux := runtime.NewServeMux()
opts := []grpc.DialOption{grpc.WithInsecure()}
err := your_package.RegisterYourServiceHandlerFromEndpoint(ctx, mux, *grpcServerEndpoint, opts)
if err != nil {
return err
}
return http.ListenAndServe(":8080", mux)
}
func main() {
flag.Parse()
if err := run(); err != nil {
log.Fatal(err)
}
}
- Build and run the gateway: Use the following command to build and run the gateway:
go build gateway.go
./gateway
Now, you have a working reverse proxy server that translates RESTful JSON API requests into Protocol Buffers and forwards them to your gRPC service.
Testing the Gateway
Let's test the gateway using a REST client tool like cURL or Postman. You can use the following command to make a POST request to the gateway:
curl -X POST -H "Content-Type: application/json" -d '{"your_field": "your_value"}' http://localhost:8080/api/your_service
The gateway will translate the request into a gRPC request and forward it to your gRPC service. You can monitor the requests and responses in your gateway server logs.
Adding Authentication and Authorization
gRPC Gateway also allows you to add authentication and authorization to your microservices. You can leverage existing tools and libraries such as OAuth2 for authentication and JWT (JSON Web Tokens) for authorization.
To add authentication and authorization, you need to modify your gateway code to handle the authentication and include authorization headers in the gRPC requests.
Wrapping Up
Congratulations! You have successfully simplified microservices communication using gRPC Gateway. Now you can build scalable and efficient microservices architectures without sacrificing the simplicity and ease-of-use of RESTful JSON APIs. Leveraging the power of gRPC, you can achieve high-performance and secure communication between your services.
Stay tuned for more blog posts where we explore advanced concepts and techniques for building microservices architectures with Go and gRPC!