Golang Logging: A Guide to Effective Log Management and Analysis
Logging is crucial in software development for debugging, error tracking, performance monitoring, and security. Learn how to set up and utilize logging effectively in your Go applications for efficient log management and analysis.
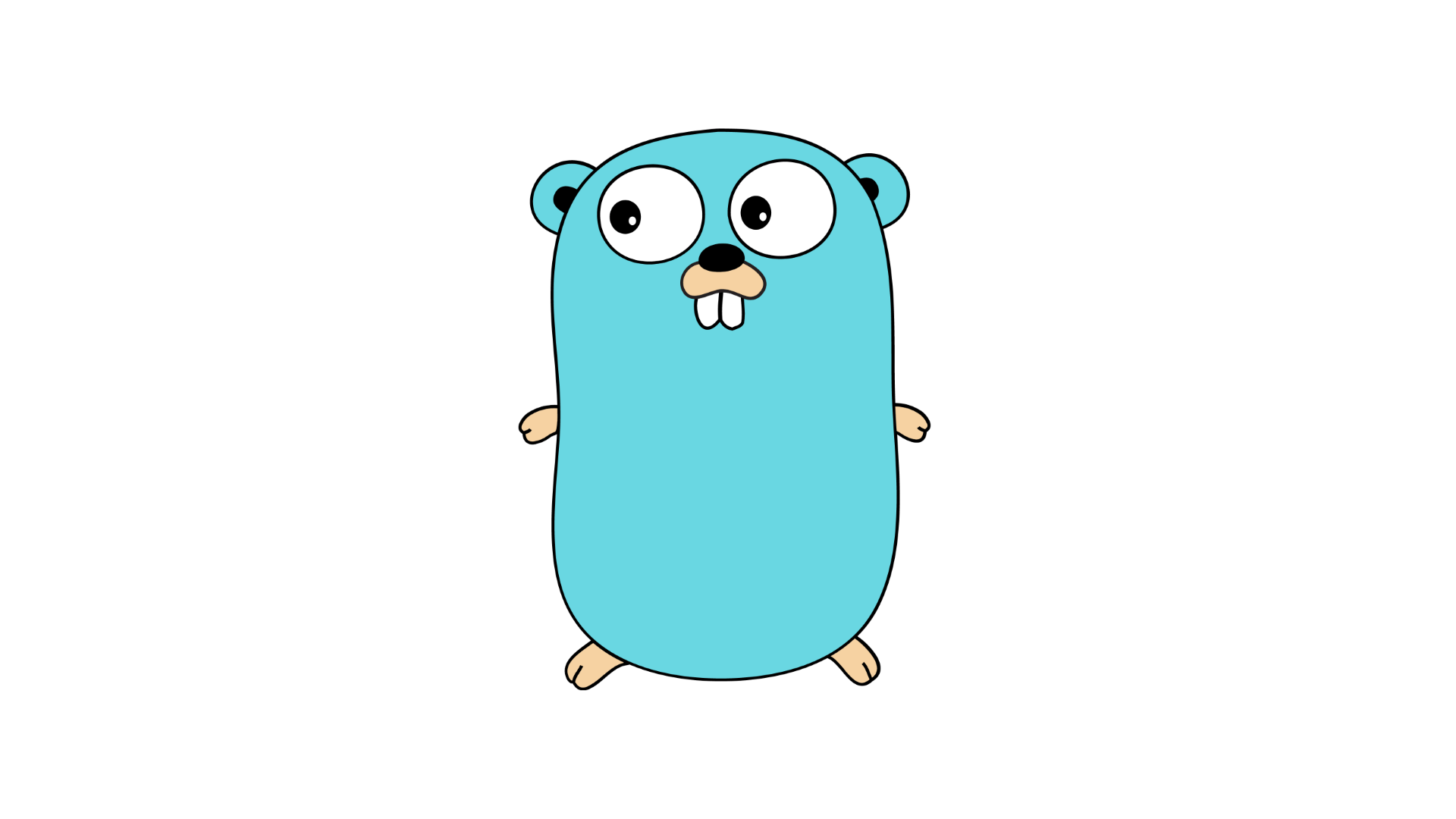
Introduction
Logging is an essential aspect of software development, allowing developers to track and analyze the behavior of applications. In Go (Golang), effective log management and analysis are crucial for identifying issues, troubleshooting problems, and monitoring application performance. This comprehensive guide will walk you through the process of setting up and utilizing logging in your Go applications, ensuring you have the tools and knowledge to effectively manage and analyze logs.
Why Logging Matters
Logging is not just a fancy feature to have in your application; it is an essential component of software development for several reasons:
- Debugging and Troubleshooting: Logs provide valuable information about the execution flow, variable values, and error messages, making it easier to identify and fix issues.
- Error Tracking: Logs help developers track errors and exceptions, providing valuable insights into the root cause of failures and enabling timely bug fixes.
- Performance Monitoring: By analyzing logs, developers can identify bottlenecks, optimize code, and improve the overall performance of the application.
- Security: Logs play a critical role in security monitoring and incident management, allowing you to detect and respond to security breaches or unusual activities.
Setting Up Logging in Go
Go provides a built-in package called log
in the standard library for basic logging functionality. However, for more advanced logging features and flexibility, many developers prefer using third-party logging libraries. Let's explore some popular logging libraries for Go:
1. Logrus
Logrus is a popular logging library that offers powerful features such as leveled logging, structured logging, and custom formatting. It is widely used in the Go community and provides an easy-to-use API.
To use Logrus, start by installing it using the following command:
$ go get github.com/sirupsen/logrus
Here's an example of how to use Logrus in your Go application:
package main
import (
"github.com/sirupsen/logrus"
)
func main() {
logrus.SetFormatter(&logrus.JSONFormatter{})
logrus.SetLevel(logrus.InfoLevel)
logrus.Info("This is an informational message")
logrus.Warn("This is a warning message")
logrus.Error("This is an error message")
}
In this example, we set the log level to InfoLevel
, which means that only log entries with a severity level of Info
and above will be recorded. The log entries are formatted using the JSON formatter for easy parsing and analysis.
2. Zap
Zap is a high-performance logging library developed by Uber. It focuses on both speed and usability and provides several advanced features, including structured logging, a fast and zero-allocation logger, and automatic production optimization.
Install Zap using the following command:
$ go get -u go.uber.org/zap
Here's an example of how to use Zap in your Go application:
package main
import (
"go.uber.org/zap"
)
func main() {
logger, _ := zap.NewProduction()
defer logger.Sync()
logger.Info("This is an informational message")
logger.Warn("This is a warning message")
logger.Error("This is an error message")
}
In this example, we create a production-level logger instance using NewProduction()
. The logger's Sync()
method ensures all buffered logs are written before the program exits.
3. Seelog
Seelog is a powerful and flexible logging library that allows you to configure logging through an XML or JSON configuration file. It supports various features such as asynchronous logging, rolling log files, log message filtering, and more.
Install Seelog using the following command:
$ go get github.com/cihub/seelog
Here's an example of how to use Seelog in your Go application:
package main
import (
"github.com/cihub/seelog"
)
func main() {
defer seelog.Flush()
logger, _ := seelog.LoggerFromConfigAsFile("seelog.xml")
seelog.ReplaceLogger(logger)
seelog.Info("This is an informational message")
seelog.Warn("This is a warning message")
seelog.Error("This is an error message")
}
In this example, we configure the logger using an XML file (seelog.xml
). The logger is replaced with the configured logger using ReplaceLogger()
. The Flush()
method ensures all buffered logs are written before the program exits.
Log Formatting and Contextual Logging
Customizing log formats and adding context to log entries can significantly enhance log readability and analysis. Let's explore how to format logs and add contextual information using the Logrus library:
Log Formatters
Logrus provides several formatters to customize log output. These include the TextFormatter
, JSONFormatter
, and LogstashFormatter
. You can choose the formatter that best suits your needs.
Here's an example of how to use different log formatters with Logrus:
package main
import (
"github.com/sirupsen/logrus"
)
func main() {
logrus.SetFormatter(&logrus.JSONFormatter{})
logrus.Info("This log entry uses the JSON formatter")
logrus.SetFormatter(&logrus.TextFormatter{})
logrus.Info("This log entry uses the text formatter")
logrus.SetFormatter(&logrus.LogstashFormatter{})
logrus.Info("This log entry uses the Logstash formatter")
}
In this example, we set the formatter to JSONFormatter
, TextFormatter
, and LogstashFormatter
to generate log entries in different formats.
Adding Contextual Information
Adding contextual information to log entries can provide valuable insights into the execution context and help in troubleshooting. Logrus allows you to add fields to log entries to provide additional information.
package main
import (
"github.com/sirupsen/logrus"
)
func main() {
logger := logrus.WithFields(logrus.Fields{
"app": "my-app",
"env": "production",
"node": "server-1",
})
logger.Info("Server started successfully")
}
In this example, we create a logger with additional fields using the WithFields()
method. The fields are passed as a map to the method. The logger will include these fields in all log entries.
Logging Levels
Logging levels allow developers to control the verbosity of the log output. Different log levels can be used to differentiate between log entries of different importance.
The common log levels used in most logging libraries include:
- Trace/Debug: Used to log detailed information for debugging purposes. These log entries are typically disabled in production environments.
- Info: Used to log informational messages about the application's operation.
- Warning: Used to log warning messages that may indicate potential issues.
- Error: Used to log error messages and exceptions.
- Fatal: Used to log critical errors that may cause the application to terminate.
Here's an example of how to use different log levels with Logrus:
package main
import (
"github.com/sirupsen/logrus"
)
func main() {
logrus.SetLevel(logrus.DebugLevel)
logrus.Trace("This is a trace level message")
logrus.Debug("This is a debug level message")
logrus.Info("This is an info level message")
logrus.Warn("This is a warning level message")
logrus.Error("This is an error level message")
logrus.Fatal("This is a fatal level message")
}
In this example, we set the log level to DebugLevel
using the SetLevel()
method. The logger will now record log entries with a severity level of Debug
and above.
Log Rotation and Retention
As your application generates more log entries, it's essential to implement log rotation and retention strategies to prevent logs from consuming excessive disk space and ensure efficient log analysis. Let's explore some approaches:
Log Rotation
Log rotation involves periodically creating a new log file and archiving the older logs. This approach helps prevent a single log file from growing indefinitely and making it difficult to manage or analyze logs. Various libraries, such as Lumberjack and file-rotatelogs, provide log rotation functionality in Go.
Here's an example of how to use Lumberjack for log rotation:
package main
import (
"github.com/sirupsen/logrus"
"gopkg.in/natefinch/lumberjack.v2"
)
func main() {
logrus.SetOutput(&lumberjack.Logger{
Filename: "app.log",
MaxSize: 100, // MB
MaxBackups: 3,
MaxAge: 30, // days
})
logrus.Info("This log entry will be rotated based on the configuration")
}
In this example, we set the output of the logger to a lumberjack.Logger
instance. The logger will create a new log file when the current file exceeds the specified maximum size (MaxSize
), keep a maximum number of backup log files (MaxBackups
), and retain log files for a specified number of days (MaxAge
).
Log Retention
Log retention involves defining a policy to delete or archive logs after a certain period. This helps manage the overall disk space usage and ensures that logs are available for analysis within a reasonable time frame. You can implement log retention policies by using a batch job or cron job that periodically removes or archives old log files based on the defined retention policy.
Analyzing Logs
Analyzing logs is an integral part of log management. It allows you to gain insights into your application's behavior, identify trends, and detect any anomalies or errors. Here are a few tips for effective log analysis:
- Centralized Logging: Consider using a centralized logging solution, such as Elastic Stack, Splunk, or Logz.io, which enables you to collect and analyze logs from multiple sources in a centralized dashboard.
- Log Filtering: Apply filters to narrow down log entries based on specific criteria, such as log level, time range, or specific keywords.
- Log Parsing and Extraction: Use regular expressions or log parsing tools to extract structured information from log entries, such as HTTP request details, error codes, or user IDs.
- Log Visualization: Utilize visualization techniques such as charts, graphs, or heatmaps to understand log patterns and identify anomalies visually.
Best Practices for Effective Logging
To ensure that your logs serve their intended purpose effectively, follow these best practices:
- Use Descriptive Log Messages: Log messages should be clear and concise, providing meaningful information about the events being logged.
- Include Relevant Context: Add contextual information to log entries, such as timestamps, request IDs, or user IDs, to aid in analysis and troubleshooting.
- Log Error Stack Traces: When logging error messages, include the stack trace to facilitate quick identification and debugging of issues.
- Apply Log Levels Appropriately: Use log levels judiciously to control log verbosity and prevent unnecessary information overload.
- Regularly Review and Update Log Configurations: Periodically review your log configurations to ensure they align with evolving application requirements and logging best practices.
Conclusion
Effective log management and analysis are instrumental in building robust and performant Go applications. By setting up logging, choosing the right logging library, and adhering to best practices, you can ensure that your logs serve as a valuable resource for troubleshooting, error tracking, and performance monitoring. Invest time in understanding your application's logging needs and leverage the power of logging libraries and analysis tools to gain valuable insights from your logs.