Securing gRPC Communication with SSL/TLS Certificates
Learn how to secure gRPC communication with SSL/TLS certificates. Encrypt your data, ensure integrity, and authenticate clients for secure client-server interactions.
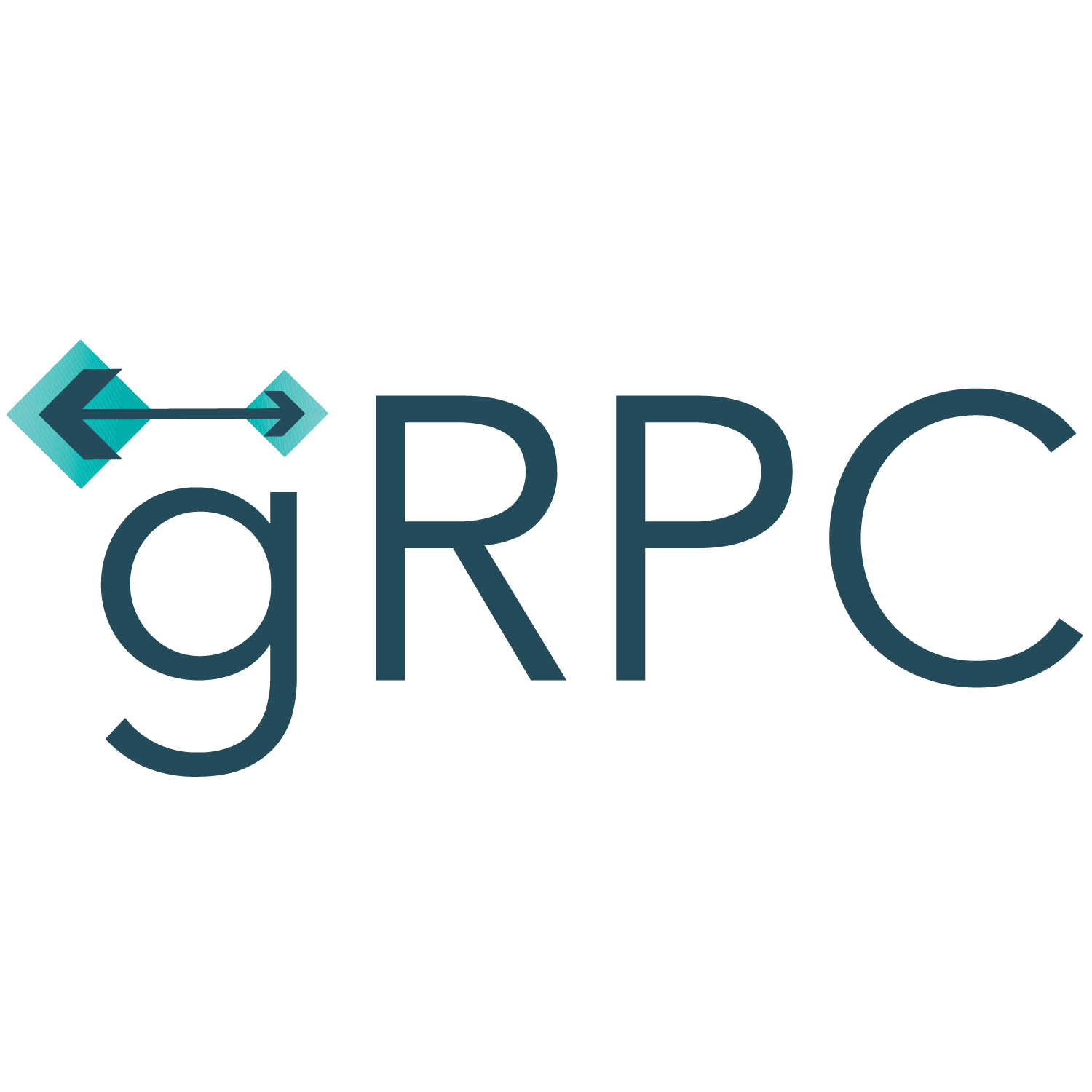
Securing gRPC Communication with SSL/TLS Certificates
gRPC is a modern, high-performance, open-source framework for building scalable and efficient distributed systems. It provides a robust and extensible RPC (Remote Procedure Call) mechanism that allows services to communicate with each other, often across different languages and platforms. While gRPC offers powerful features out of the box, it's crucial to ensure secure communication between client and server. In this article, we'll explore how to secure gRPC communication with SSL/TLS certificates.
What is SSL/TLS?
SSL (Secure Sockets Layer) and TLS (Transport Layer Security) are cryptographic protocols that provide secure data transmission over the internet. They establish an encrypted connection between clients and servers, ensuring privacy, data integrity, and authentication. SSL/TLS certificates play a vital role in this process by verifying the identity of the communicating parties.
Why SSL/TLS for gRPC?
Securing gRPC communication with SSL/TLS certificates brings several benefits:
- Data Encryption: SSL/TLS encrypts the data transmitted between the client and server, ensuring that it cannot be intercepted or read by unauthorized entities.
- Data Integrity: SSL/TLS guarantees that the data received is not tampered with during transmission, as it detects and rejects any alterations in the encrypted data.
- Authentication: SSL/TLS certificates verify the identities of the client and server, ensuring that only trusted and authorized parties can establish a connection.
- Trustworthiness: By using SSL/TLS certificates, you establish trust with your clients, assuring them that their data is transmitted securely.
Generating Self-Signed Certificates
Before we dive into securing gRPC communication, let's generate self-signed SSL/TLS certificates for our development environment. Self-signed certificates are useful for testing and local development, but for production use, it's recommended to acquire certificates from a trusted certificate authority (CA).
To generate a self-signed certificate, follow these steps:
- Open a terminal or command prompt.
- Navigate to a directory where you want to generate the certificates.
- Run the following command to generate a private key:
openssl genrsa -out server.key 2048
- Next, generate a certificate signing request (CSR) using the private key:
openssl req -new -key server.key -out server.csr
- You'll be prompted to provide information about yourself and your organization. Fill in the required information accordingly.
- Finally, generate the self-signed certificate using the CSR and private key:
openssl x509 -req -days 365 -in server.csr -signkey server.key -out server.crt
Now you have a self-signed SSL/TLS certificate (server.crt
) and a private key (server.key
) that you can use for securing gRPC communication in your development environment.
Configuring gRPC Server with SSL
Once you have your SSL/TLS certificate and private key, you can configure the gRPC server to use them for secure communication. Here's an example of how to configure a gRPC server with SSL:
package main
import (
"crypto/tls"
"crypto/x509"
"log"
"net"
"google.golang.org/grpc"
"google.golang.org/grpc/credentials"
)
func main() {
cert, err := tls.LoadX509KeyPair("server.crt", "server.key")
if err != nil {
log.Fatalf("failed to load certificates: %v", err)
}
certPool := x509.NewCertPool()
bs, err := ioutil.ReadFile("server.crt")
if err != nil {
log.Fatalf("failed to read CA certificate: %v", err)
}
ok := certPool.AppendCertsFromPEM(bs)
if !ok {
log.Fatalf("failed to append CA certificate")
}
tlsConfig := &tls.Config{
Certificates: []tls.Certificate{cert},
ClientAuth: tls.RequireAndVerifyClientCert,
ClientCAs: certPool,
}
lis, err := net.Listen("tcp", ":50051")
if err != nil {
log.Fatalf("failed to listen: %v", err)
}
s := grpc.NewServer(grpc.Creds(credentials.NewTLS(tlsConfig)))
// Register your gRPC service handlers here...
if err := s.Serve(lis); err != nil {
log.Fatalf("failed to serve: %v", err)
}
}
In this example, we load the SSL/TLS certificates and private key using tls.LoadX509KeyPair()
and create a tls.CertPool
to store the CA certificate. We then configure the tls.Config
with the loaded certificates and set the ClientAuth
property to tls.RequireAndVerifyClientCert
, which requires clients to present valid certificates.
The gRPC server is created with grpc.NewServer(grpc.Creds(credentials.NewTLS(tlsConfig)))
, ensuring that it uses the TLS credentials for secure communication.
Configuring gRPC Client with SSL
To configure a gRPC client to use SSL/TLS for secure communication, follow these steps:
- Load the server's SSL/TLS certificate:
cert, err := ioutil.ReadFile("server.crt")
if err != nil {
log.Fatalf("failed to read server certificate: %v", err)
}
- Configure a
tls.Config
with the loaded certificate:
certPool := x509.NewCertPool()
ok := certPool.AppendCertsFromPEM(cert)
if !ok {
log.Fatalf("failed to append server certificate")
}
tlsConfig := &tls.Config{
RootCAs: certPool,
}
- Create a
grpc.DialOption
with the TLS credentials:
opt := grpc.WithTransportCredentials(credentials.NewTLS(tlsConfig))
- Use the
grpc.Dial
function to establish a connection to the gRPC server:
conn, err := grpc.Dial("localhost:50051", opt)
if err != nil {
log.Fatalf("failed to connect: %v", err)
}
defer conn.Close()
The gRPC client loads the server's SSL/TLS certificate, creates a tls.Config
with the certificate, and uses grpc.WithTransportCredentials()
to configure the connection with TLS credentials. The grpc.Dial()
function establishes a connection to the gRPC server, ensuring secure communication.
Conclusion
Securing gRPC communication with SSL/TLS certificates is essential to protect sensitive data and ensure secure client-server interactions. By following the steps outlined in this article, you can generate self-signed SSL/TLS certificates, configure the gRPC server and client to use them, and establish secure communication channels.
Remember that self-signed certificates are suitable for development and testing purposes, but for production use, it's recommended to obtain certificates from a trusted CA.
With secure gRPC communication in place, you can confidently build and deploy robust distributed systems that harness the power of gRPC while maintaining privacy and data integrity.
Thank you for reading! Stay tuned for more articles on gRPC and secure communication.