RabbitMQ Message Acknowledgements: Ensuring Reliable Message Delivery
Learn how RabbitMQ message acknowledgements ensure reliable message delivery in distributed systems, handling failures, requeuing messages, and controlling message flow.
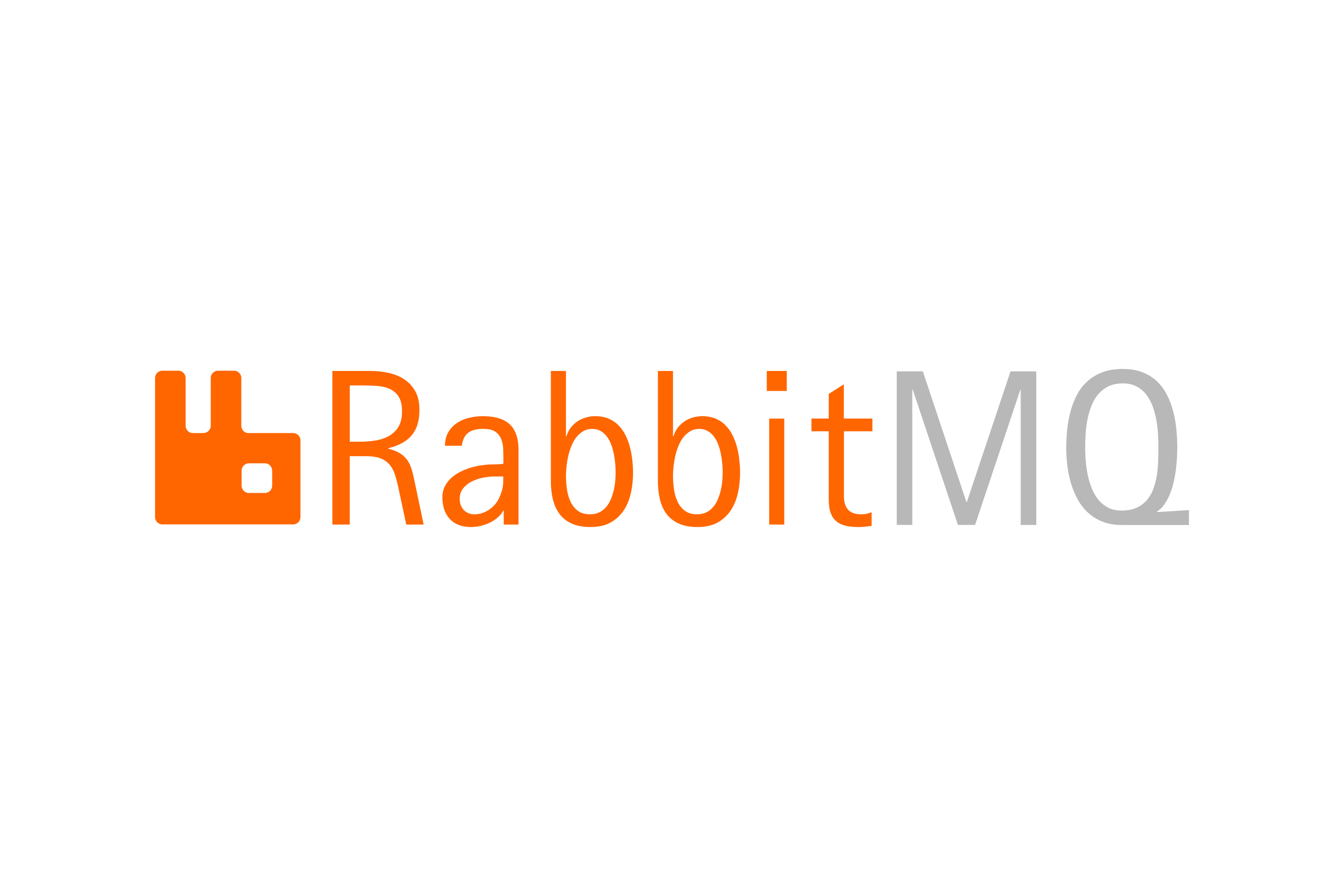
Introduction
When it comes to building distributed systems, reliable message delivery is of paramount importance. In such systems, it's crucial to ensure that messages are safely delivered from producers to consumers. That's where RabbitMQ message acknowledgements come into play. In this article, we'll explore the concept of message acknowledgements in RabbitMQ and how they help ensure reliable message delivery.
What are Message Acknowledgements?
In RabbitMQ, message acknowledgements are a mechanism that allows consumers to inform the broker about the successful processing of messages. When a consumer receives a message from the broker, it can either explicitly acknowledge the message or reject it. Message acknowledgements provide a way to handle the different failure scenarios that may occur during message processing.
Basic Message Acknowledgements
The basic form of message acknowledgement in RabbitMQ is called manual acknowledgements. In this mode, a consumer manually sends an acknowledgement to the broker once it has finished processing a message. The acknowledgement can be positive or negative, depending on the outcome of the processing.
// Create a new channel and declare a queue
Channel channel = connection.createChannel();
channel.queueDeclare("my-queue", true, false, false, null);
// Start consuming messages from the queue
channel.basicConsume("my-queue", false, "my-consumer-tag",
new DefaultConsumer(channel) {
// Handle incoming messages
public void handleDelivery(
String consumerTag,
Envelope envelope,
AMQP.BasicProperties properties,
byte[] body
) throws IOException {
// Process the message
processMessage(body);
// Send positive acknowledgement
channel.basicAck(envelope.getDeliveryTag(), false);
}
}
);
In the code snippet above, we create a new channel and declare a queue called "my-queue." Then, we start consuming messages from the queue using the basicConsume
method. Inside the handleDelivery
method, we process the incoming message and send a positive acknowledgement using the basicAck
method, passing the delivery tag and the false
parameter to indicate that we are not acknowledging multiple messages at once.
Acknowledging Multiple Messages
Aside from acknowledging a single message at a time, RabbitMQ also provides a mechanism for acknowledging multiple messages in bulk. This can significantly improve the performance of message acknowledgements.
// Create a new channel and declare a queue
Channel channel = connection.createChannel();
channel.queueDeclare("my-queue", true, false, false, null);
// Start consuming messages from the queue
channel.basicConsume("my-queue", false, "my-consumer-tag",
new DefaultConsumer(channel) {
// Keep track of the received delivery tags
List<Long> deliveryTags = new ArrayList<>();
// Handle incoming messages
public void handleDelivery(
String consumerTag,
Envelope envelope,
AMQP.BasicProperties properties,
byte[] body
) throws IOException {
// Process the message
processMessage(body);
// Keep track of the delivery tag
deliveryTags.add(envelope.getDeliveryTag());
// Acknowledge every N messages
if (deliveryTags.size() % 10 == 0) {
channel.basicAck(
deliveryTags.get(deliveryTags.size() - 1), // Last delivered message
true // Acknowledge multiple messages
);
}
}
}
);
In the code snippet above, we introduce a deliveryTags
list to keep track of the received delivery tags. For every incoming message, we process it and add its delivery tag to the list. Then, we check if the number of messages received is a multiple of 10. If so, we send a positive acknowledgement for the last delivered message using the basicAck
method with the true
parameter to acknowledge multiple messages.
Negative Acknowledgements and Requeuing
In addition to positive acknowledgements, RabbitMQ also supports negative acknowledgements, also known as message rejection. When a consumer determines that a message cannot be processed successfully, it can send a negative acknowledgement to the broker, asking it to requeue the message for redelivery or handle it according to a dead letter exchange policy.
// Create a new channel and declare a queue
Channel channel = connection.createChannel();
channel.queueDeclare("my-queue", true, false, false, null);
// Start consuming messages from the queue
channel.basicConsume("my-queue", false, "my-consumer-tag",
new DefaultConsumer(channel) {
// Handle incoming messages
public void handleDelivery(
String consumerTag,
Envelope envelope,
AMQP.BasicProperties properties,
byte[] body
) throws IOException {
// Process the message
boolean success = processMessage(body);
// Send acknowledgment based on the processing result
if (success) {
channel.basicAck(envelope.getDeliveryTag(), false);
} else {
channel.basicNack(
envelope.getDeliveryTag(),
false, // Do not requeue the message
false // Do not ignore unacknowledged messages
);
}
}
}
);
In the code snippet above, after processing the message, we introduce a boolean variable called success
to indicate whether the processing was successful or not. If the processing is successful, we send a positive acknowledgement using the basicAck
method. If the processing fails, we send a negative acknowledgement using the basicNack
method, passing the delivery tag, false
to indicate that we do not want the message to be requeued, and false
to indicate that we do not want to ignore unacknowledged messages.
Pre-fetch Count
In RabbitMQ, the pre-fetch count determines the number of messages that can be sent to a consumer before receiving any acknowledgements. By default, the pre-fetch count is set to 1, meaning that RabbitMQ will only send one message at a time before waiting for an acknowledgment.
// Create a new channel and set the pre-fetch count
Channel channel = connection.createChannel();
channel.basicQos(10); // Allow 10 unacknowledged messages at a time
// Start consuming messages from the queue
channel.basicConsume("my-queue", false, "my-consumer-tag",
new DefaultConsumer(channel) {
// Handle incoming messages
public void handleDelivery(
String consumerTag,
Envelope envelope,
AMQP.BasicProperties properties,
byte[] body
) throws IOException {
// Process the message
processMessage(body);
// Send positive acknowledgement
channel.basicAck(envelope.getDeliveryTag(), false);
}
}
);
In the code snippet above, we set the pre-fetch count to 10 using the basicQos
method. This means that RabbitMQ will send up to 10 unacknowledged messages to the consumer at a time. Once the consumer acknowledges a message, RabbitMQ will send the next message.
Acknowledgements and Durability
It's important to note that message acknowledgements are separate from message durability in RabbitMQ. Acknowledgements ensure that a message is successfully processed by a consumer, while durability ensures that a message is not lost in case of broker failures or restarts.
If you want to ensure both reliable message delivery and message durability, you need to combine message acknowledgements with the appropriate durability settings. This includes declaratively marking queues, exchanges, and messages as durable, and configuring appropriate persistence options.
Reliable Message Delivery with RabbitMQ Acknowledgements
RabbitMQ message acknowledgements play a vital role in ensuring reliable message delivery. By properly implementing and utilizing message acknowledgements, you can handle message processing failures, requeue messages when needed, and control the flow of messages between producers and consumers. This results in a robust and fault-tolerant distributed system.
Conclusion
In this article, we explored the concept of RabbitMQ message acknowledgements and how they help ensure reliable message delivery in distributed systems. We covered basic message acknowledgements, acknowledging multiple messages, negative acknowledgements and requeuing, pre-fetch count, and the relationship between acknowledgements and message durability.
By incorporating message acknowledgements into your RabbitMQ workflows, you can build more reliable and resilient distributed systems where message delivery is guaranteed.
Happy messaging!