Redis Lua Scripting: Extending Redis with Custom Functionality
Redis Lua scripting allows you to extend Redis with custom scripts, reducing round-trips, and improving performance. Learn the basics, advantages, and best practices to get started with Redis Lua scripting.
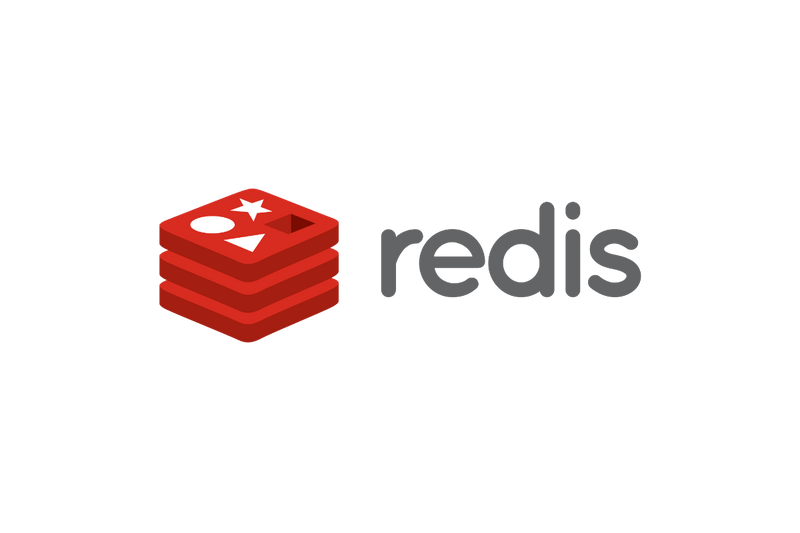
Introduction
Redis is a popular open-source in-memory data structure store that is known for its speed, versatility, and simplicity. It provides a variety of data structures and functionalities, making it a powerful tool for developers. One of the lesser-known features of Redis is its support for Lua scripting.
Redis Lua scripting allows you to extend Redis by writing custom scripts in Lua programming language. This feature gives you the flexibility to execute complex operations on the server side, reduce round-trips between the client and server, and optimize performance.
In this blog post, we will explore Redis Lua scripting and learn how to extend Redis with custom functionality. We will cover the basics of Lua scripting, the advantages of using Lua scripts in Redis, and provide practical examples to help you get started.
What is Lua Scripting?
Lua is a lightweight and efficient scripting language that is embedded in many applications and systems, including Redis. It was designed to be simple yet powerful, with a small footprint and a fast interpreter.
Redis Lua scripting allows you to write Lua scripts that can be executed within Redis. These scripts can access and manipulate Redis data structures, perform complex operations, and even define custom functions that can be used in subsequent script invocations.
Advantages of Using Lua Scripts in Redis
There are several advantages to using Lua scripts in Redis:
1. Reduce Round-Trips Between Client and Server
When you use Lua scripts in Redis, you can send a complete script to the server and execute it in a single round-trip. This reduces the number of round-trips between the client and server, resulting in improved performance, especially for complex operations that require multiple commands.
2. Atomicity and Transaction Support
Lua scripts in Redis offer atomic execution, meaning that the entire script is executed as a single unit. This ensures that Redis commands within the script are executed sequentially and without interruption, providing atomicity and consistency. Additionally, Lua scripts can be executed within a Redis transaction, allowing you to group multiple operations into a single transaction.
3. Reusability of Scripts
With Redis Lua scripting, you can define custom functions within your scripts. These functions can be reused across different scripts and even within the same script, making it easy to abstract complex operations into reusable components. This promotes code reusability, simplifies script maintenance, and improves development efficiency.
4. Better Performance
Since Redis Lua scripting allows you to perform complex operations on the server side, you can leverage the native Lua language features and libraries to optimize performance. By moving computation-intensive tasks to the server side, you can reduce the load on the client side and improve overall application performance.
Getting Started with Redis Lua Scripting
Now that we understand the benefits of Redis Lua scripting, let's dive into the practical aspects of using Lua scripts in Redis. Here are the steps to get started:
1. Install Redis
If you haven't already, you need to install Redis on your local machine or server. You can download Redis from the official website or use a package manager to install it. Make sure you have the latest stable version of Redis installed.
2. Enable Lua Scripting in Redis
By default, Lua scripting is enabled in Redis. However, you can double-check by opening the Redis configuration file (redis.conf) and ensuring that the lua-enabled
configuration option is set to true
.
3. Connect to Redis Server
Next, connect to the Redis server using a Redis client. You can use the Redis CLI (Command Line Interface) or any other Redis client libraries available in your programming language of choice.
4. Write and Execute Lua Scripts
Once you have connected to the Redis server, you can start writing and executing Lua scripts. A Lua script in Redis is enclosed within the EVAL
command, which takes the script as a parameter along with any necessary arguments.
Here's an example of a simple Lua script that increments a counter:
local counter = tonumber(redis.call('GET', 'counter') or '0')
counter = counter + 1
redis.call('SET', 'counter', counter)
return counter
In this example, the Lua script retrieves the current value of the 'counter' key using the Redis GET
command. If the key does not exist, it defaults to 0. It then increments the counter by one and updates the value using the Redis SET
command. Finally, the script returns the new value of the counter.
To execute this script, use the EVAL
command in the Redis CLI:
EVAL "local counter = tonumber(redis.call('GET', 'counter') or '0')\ncounter = counter + 1\nredis.call('SET', 'counter', counter)\nreturn counter" 0
The EVAL
command takes the Lua script as the first parameter and the number of arguments as the second parameter. In this example, the script does not require any arguments, so the second parameter is set to 0.
After executing the script, you should see the new value of the counter printed in the Redis CLI.
5. Explore Complex Operations and Custom Functions
Redis Lua scripting allows you to perform complex operations and define custom functions. You can leverage the full power of Lua language features and libraries to implement advanced functionality within your scripts.
For example, you can use Lua tables and iterators to process Redis hashes or sets, perform mathematical computations using Lua's math library, or even call external Lua libraries to extend the functionality of Redis.
Best Practices and Tips
To make the most of Redis Lua scripting, here are some best practices and tips to keep in mind:
1. Keep Scripts Small and Modular
Since Lua scripts are executed as a single unit, it's important to keep them small and modular. Splitting complex operations into smaller, reusable scripts makes them easier to maintain and debug.
2. Use Redis Scripts Caching
Redis provides a built-in script caching mechanism that can improve performance for repeated script invocations. By storing the script's SHA1 hash and reusing it instead of sending the entire script on each invocation, you can reduce network overhead and improve execution speed.
3. Handle Errors Gracefully
Lua scripts in Redis can throw runtime errors that need to be handled gracefully. You can use pcall
or xpcall
functions to catch and handle errors within your scripts. Additionally, make sure to validate input parameters and handle possible edge cases in your scripts to prevent unexpected behavior.
4. Test and Benchmark Scripts
Before deploying Lua scripts in a production environment, it's important to thoroughly test and benchmark them. This ensures that the scripts function correctly and perform well under different load conditions. You can use Redis's built-in benchmarking tools or external tools like redis-benchmark
to measure the performance of your scripts.
Conclusion
Redis Lua scripting is a powerful feature that allows you to extend Redis with custom functionality. By using Lua scripts, you can reduce round-trips between the client and server, achieve atomicity and transaction support, promote code reusability, and improve performance. Whether you need to perform complex operations or implement advanced functionality, Redis Lua scripting is a valuable tool.
By following the steps outlined in this blog post, you can get started with Redis Lua scripting and explore the endless possibilities it offers. Remember to keep best practices and tips in mind, test your scripts thoroughly, and have fun experimenting with Redis Lua scripting.
Now, it's time to level up your Redis skills and take advantage of Lua scripting in Redis. Happy scripting!