Redis Data Types: Exploring Strings, Lists, Sets, and Hashes
Redis is an open-source, in-memory data structure store that is known for its speed and versatility. It supports strings, lists, sets, and hashes, making it a powerful tool for various applications. Explore the different data types in Redis and learn how to use them effectively in your projects.
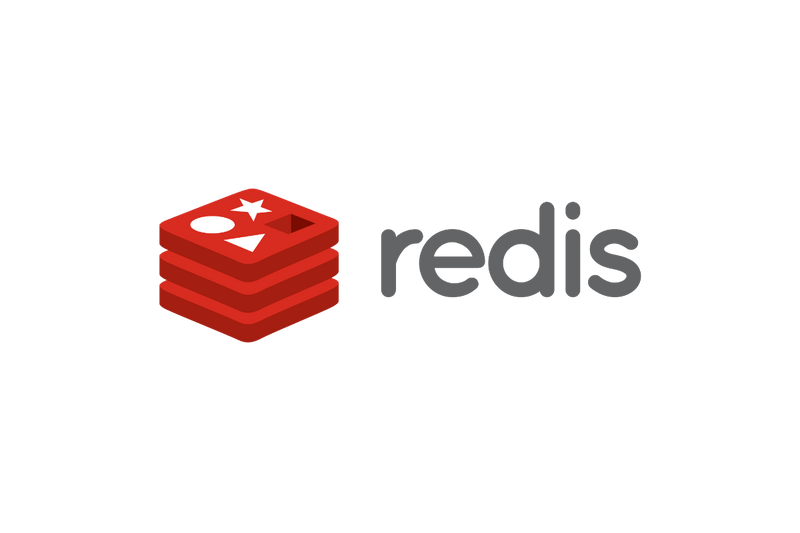
Introduction
Redis is an open-source, in-memory data structure store that is known for its speed, versatility, and ease of use. It supports a wide range of data types and provides powerful operations on these data types. In this article, we will explore the different data types in Redis and learn how to use them effectively in your applications.
Strings
Strings are the most basic data type in Redis. They can store any kind of data, such as text, numbers, or binary data. Redis strings are binary safe, which means they can contain any sequence of bytes.
Here are some common operations you can perform on Redis strings:
SET
command: Set the value of a key to a string.GET
command: Get the value of a key.INCR
command: Increment the value of a key by 1.APPEND
command: Append a value to a key.
SET name "John"
GET name
INCR counter
APPEND message "Hello, World!"
Lists
Lists in Redis are a collection of ordered strings. Redis lists are implemented as doubly linked lists, which makes it easy to perform operations such as push, pop, or slice.
Here are some common operations you can perform on Redis lists:
LPUSH
command: Insert one or more values at the beginning of a list.RPUSH
command: Insert one or more values at the end of a list.LPOP
command: Remove and get the first element of a list.RPOP
command: Remove and get the last element of a list.LLEN
command: Get the length of a list.
LPUSH colors "red" "blue" "green"
RPUSH colors "yellow"
LPOP colors
RPOP colors
LLEN colors
Sets
Sets in Redis are an unordered collection of unique strings. Redis sets are ideal for storing tags, user IDs, or any other data that needs to have unique values.
Here are some common operations you can perform on Redis sets:
SADD
command: Add one or more members to a set.SMEMBERS
command: Get all members of a set.SISMEMBER
command: Check if a member exists in a set.SREM
command: Remove one or more members from a set.
SADD fruits "apple" "banana" "orange"
SMEMBERS fruits
SISMEMBER fruits "apple"
SREM fruits "banana"
Hashes
Hashes in Redis are key-value pairs where the keys and values are strings. Redis hashes are great for representing objects or complex data structures.
Here are some common operations you can perform on Redis hashes:
HSET
command: Set the value of a field in a hash.HGET
command: Get the value of a field in a hash.HDEL
command: Delete one or more fields from a hash.HKEYS
command: Get all keys in a hash.
HSET user:id1 name "John" email "john@example.com"
HGET user:id1 name
HDEL user:id1 email
HKEYS user:id1
Conclusion
Redis provides a rich set of data types that are powerful and easy to use. By understanding the different data types - strings, lists, sets, and hashes - and their respective operations, you can make the most of Redis in your applications.
Remember, Redis is not just a key-value store, it's a versatile data structure store that can handle a wide range of use cases. So, don't hesitate to dive deeper into Redis and explore its full potential.
Happy coding!