Kafka Streams: Processing Real-Time Data with Apache Kafka
Discover how Kafka Streams enables real-time data processing using a scalable, fault-tolerant, and developer-friendly platform. Analyze clickstream data, calculate metrics, and seamlessly integrate with Kafka for real-time analytics.
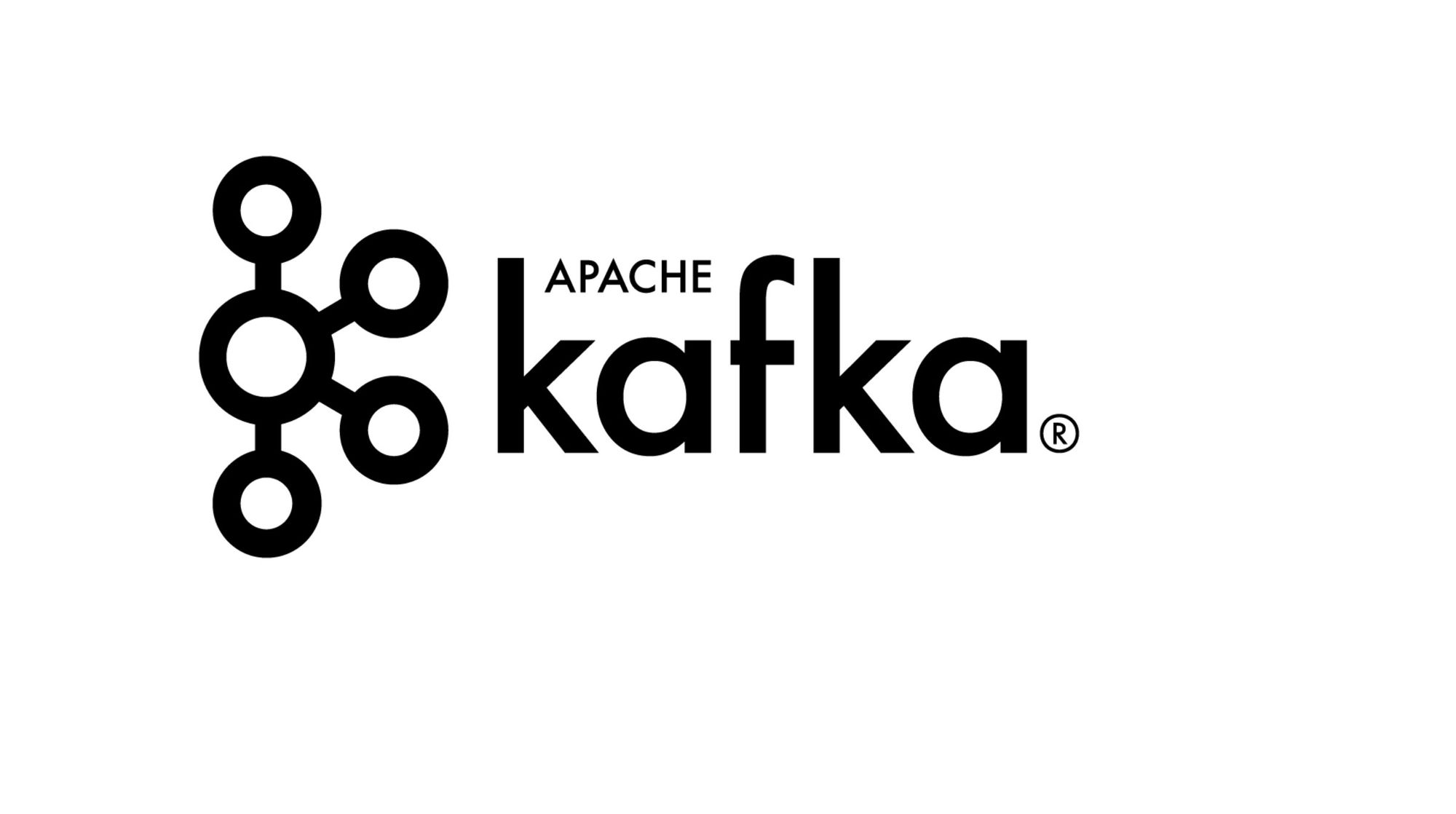
Introduction
Welcome to the world of Kafka Streams! In this blog post, we will explore how you can process real-time data using Apache Kafka and its powerful stream processing library, Kafka Streams. With Kafka Streams, you can build highly scalable and fault-tolerant stream processing applications that can seamlessly integrate with your existing Kafka infrastructure. Whether you are dealing with high-throughput data streams or real-time analytics, Kafka Streams provides a robust platform to harness the power of real-time data processing.
What is Kafka Streams?
Kafka Streams is a stream processing library provided by Apache Kafka. It enables developers to build real-time applications and microservices that can process and analyze streams of data in a scalable and fault-tolerant manner. Kafka Streams leverages the distributed nature of Kafka and provides a high-level API to define stream processing operations such as filtering, mapping, aggregating, and joining, among others.
Why Use Kafka Streams?
Kafka Streams offers several advantages that make it a popular choice for real-time data processing:
- Scalability: Kafka Streams provides built-in support for distributed processing, allowing you to scale your stream processing applications horizontally as the data volume or complexity increases.
- High fault-tolerance: Kafka Streams takes advantage of Kafka's fault-tolerant design, ensuring that your stream processing applications are resilient to failures and can seamlessly recover from any errors.
- Integration with Kafka: Kafka Streams seamlessly integrates with the Kafka ecosystem, enabling you to easily consume and produce data from Kafka topics. It supports exactly-once processing semantics, ensuring that your application state is not inconsistent, even in the presence of failures.
- Developer-friendly API: Kafka Streams provides a high-level, functional programming-like API, making it easy for developers to define and execute stream processing operations without dealing with the complexity of distributed systems.
Real-Time Data Processing with Kafka Streams
To get started with Kafka Streams, you need to have a working Kafka installation and a basic understanding of Kafka's core concepts, such as topics, partitions, and producers/consumers. Once you have set up your Kafka cluster, you can start building your stream processing application using Kafka Streams.
Let's walk through a simple example to demonstrate the power of Kafka Streams in real-time data processing.
Example Scenario
Suppose you are building a real-time analytics application that processes clickstream data generated by a website. The clickstream data consists of events such as page views, clicks, and purchases. You want to analyze this data in real-time to calculate metrics such as total page views, unique visitors, and average time spent on the website.
Building the Stream Processing Topology
In Kafka Streams, a stream processing application is defined as a directed graph of processing stages, where each stage represents a stream processor that transforms the input data and produces an output stream. The graph is called a "topology" and is defined using the Kafka Streams DSL (Domain-Specific Language).
Let's define the stream processing topology for our clickstream analytics application:
StreamsBuilder builder = new StreamsBuilder();
// Step 1: Create a source stream from the 'clickstream' topic
KStream<String, ClickEvent> clickstream = builder.stream("clickstream");
// Step 2: Perform filtering to exclude irrelevant events
KStream<String, ClickEvent> filteredStream = clickstream
.filter((key, event) -> event.getEventType().equals("page_view"));
// Step 3: Perform aggregations to calculate metrics
KTable<String, Long> pageViews = filteredStream
.groupBy((key, event) -> event.getPageId())
.count();
KTable<String, Long> uniqueVisitors = filteredStream
.groupBy((key, event) -> event.getUserId())
.count();
// Step 4: Create an output stream to publish the calculated metrics
pageViews.toStream().to("page_views");
uniqueVisitors.toStream().to("unique_visitors");
// Step 5: Build and start the Kafka Streams application
KafkaStreams streams = new KafkaStreams(builder.build(), config);
streams.start();
Let's understand what's happening in each step:
- We create a source stream from the "clickstream" topic using the `stream` method. Each record in the stream represents a click event.
- We filter the stream to exclude irrelevant events such as clicks on ads or non-page view events.
- We perform two aggregations using the `groupBy` and `count` operations. The first aggregation groups the events by page ID and counts the number of page views for each page. The second aggregation groups the events by user ID and counts the number of unique visitors.
- We create two KTables, `pageViews` and `uniqueVisitors`, which represent the calculated metrics. We then publish these KTables as output streams to the "page_views" and "unique_visitors" topics, respectively.
- We build the Kafka Streams application using `builder.build()` and start it using `streams.start()`.
Consuming the Calculated Metrics
Once the stream processing application is running, you can consume the calculated metrics from the output topics and perform further analysis or visualization. For example, you can use a separate Kafka consumer to consume the "page_views" topic and store the metrics in a database for reporting purposes.
Monitoring and Scaling
Kafka Streams provides built-in support for monitoring and scaling your stream processing applications. You can use tools such as Kafka's built-in metrics, Prometheus, or Grafana to monitor the performance and health of your Kafka Streams application. Additionally, you can scale your application by adding more instances or partitions to the underlying Kafka topics.
Conclusion
With Kafka Streams, processing real-time data has never been easier. Its scalable and fault-tolerant architecture, integration with Kafka, and developer-friendly API make it an ideal choice for building real-time applications and microservices. Whether you are building real-time analytics, monitoring, or fraud detection systems, Kafka Streams provides a powerful platform to process and analyze streams of data in real-time. So why wait? Start exploring Kafka Streams and unlock the potential of real-time data processing with Apache Kafka!