RabbitMQ Exchange Types: Understanding Direct, Fanout, Topic, and Headers Exchanges
In this blog post, we explore the different types of RabbitMQ exchanges: Direct, Fanout, Topic, and Headers. Learn how to use them efficiently to design messaging architectures for your applications.
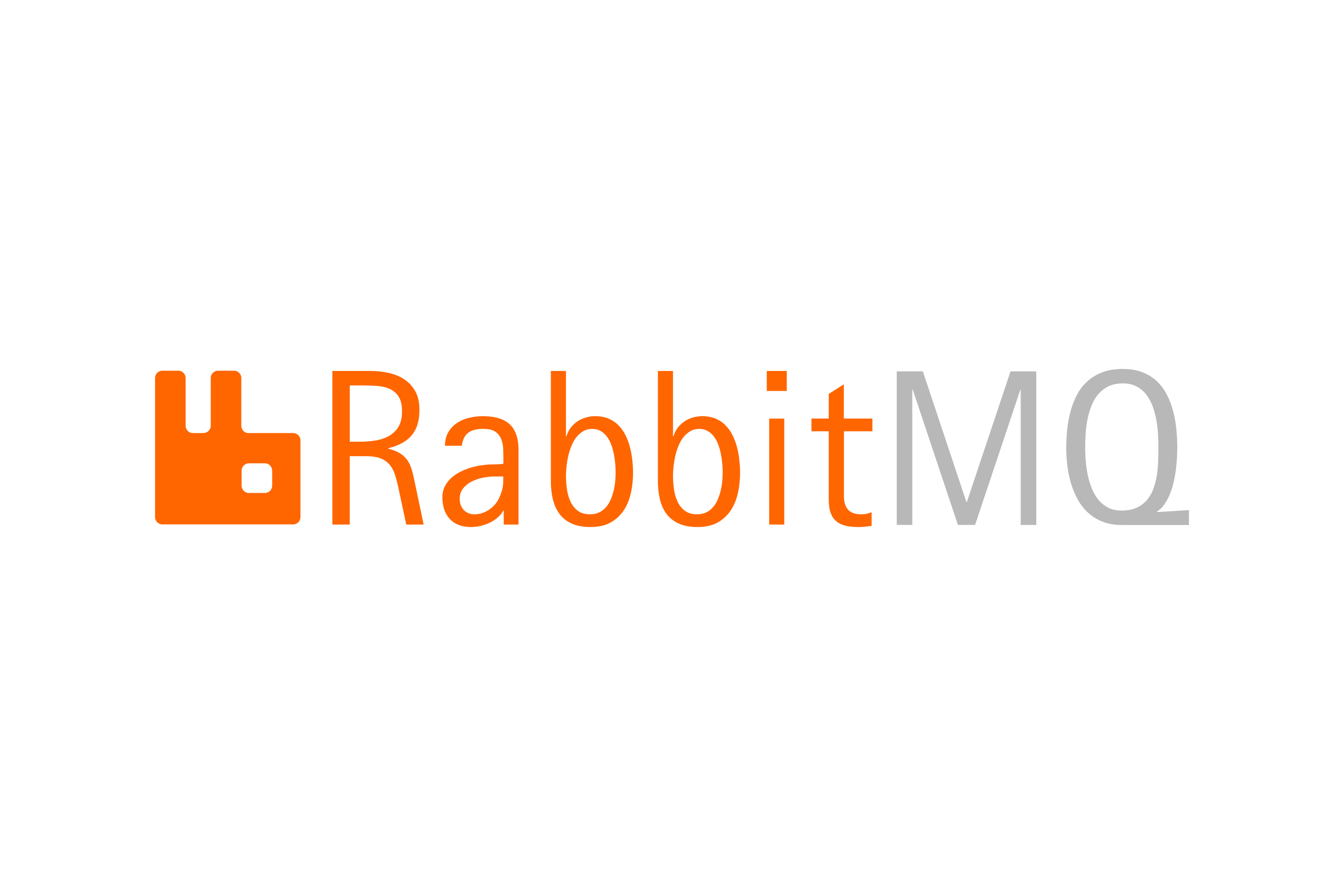
Introduction
RabbitMQ is a powerful message broker that allows applications to communicate with each other by sending and receiving messages. One of the key concepts in RabbitMQ is exchanges, which act as intermediaries that receive messages from producers and route them to queues based on certain criteria.
In this blog post, we'll dive into the world of RabbitMQ exchanges and explore the different types available: Direct, Fanout, Topic, and Headers exchanges. By understanding the characteristics and use cases of each exchange type, you'll be able to design and implement efficient messaging architectures in your applications.
Direct Exchange
The direct exchange is the simplest type of exchange in RabbitMQ. It routes messages to queues based on a routing key. When a producer sends a message with a specific routing key, the direct exchange delivers it to the queue(s) with a matching binding key.
Here's an example of how the direct exchange works:
import pika
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
channel.exchange_declare(exchange='direct_exchange', exchange_type='direct')
# Create the queue
channel.queue_declare(queue='queue1')
channel.queue_declare(queue='queue2')
# Bind queue1 to the direct exchange with a binding key of 'key1'
channel.queue_bind(exchange='direct_exchange', queue='queue1', routing_key='key1')
# Bind queue2 to the direct exchange with a binding key of 'key2'
channel.queue_bind(exchange='direct_exchange', queue='queue2', routing_key='key2')
# Publish a message with the routing key 'key1'
channel.basic_publish(exchange='direct_exchange', routing_key='key1', body='Hello, queue1!')
# Publish a message with the routing key 'key2'
channel.basic_publish(exchange='direct_exchange', routing_key='key2', body='Hello, queue2!')
connection.close()
In this example, we declare a direct exchange named 'direct_exchange'. We also create two queues named 'queue1' and 'queue2'. We then bind 'queue1' to the exchange with a binding key of 'key1', and bind 'queue2' to the exchange with a binding key of 'key2'.
Finally, we publish two messages with different routing keys. The message with the routing key 'key1' will be delivered to 'queue1', while the message with the routing key 'key2' will be delivered to 'queue2'.
The direct exchange is useful when you want to deliver messages to specific queues based on a routing key. It provides a simple and efficient way to implement point-to-point communication between producers and consumers.
Fanout Exchange
The fanout exchange is the easiest exchange type to understand. It simply broadcasts all the messages it receives to all the queues bound to it. In other words, the fanout exchange ignores routing keys and delivers messages to all connected queues.
Here's an example of how the fanout exchange works:
import pika
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
channel.exchange_declare(exchange='fanout_exchange', exchange_type='fanout')
# Create the queues
channel.queue_declare(queue='queue1')
channel.queue_declare(queue='queue2')
# Bind queue1 and queue2 to the fanout exchange
channel.queue_bind(exchange='fanout_exchange', queue='queue1')
channel.queue_bind(exchange='fanout_exchange', queue='queue2')
# Publish a message to the fanout exchange
channel.basic_publish(exchange='fanout_exchange', routing_key='', body='Hello, everyone!')
connection.close()
In this example, we declare a fanout exchange named 'fanout_exchange'. We create two queues named 'queue1' and 'queue2', and bind both of them to the fanout exchange without specifying a routing key.
When we publish a message to the fanout exchange, it broadcasts the message to all the connected queues. In this case, both 'queue1' and 'queue2' will receive the message.
The fanout exchange is useful when you want to distribute messages to multiple queues without any filtering. It provides a simple way to implement publish-subscribe-type communication, where multiple consumers receive the same messages.
Topic Exchange
The topic exchange is more flexible than the direct and fanout exchanges. It routes messages to queues based on a pattern specified by the binding keys.
Binding keys for topic exchanges are formed using words separated by dots (e.g., "stock.usd.nasdaq"). Each word can be a dot-separated hierarchy, and you can use wildcard characters to match multiple words or parts of a word.
Here's an example of how the topic exchange works:
import pika
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
channel.exchange_declare(exchange='topic_exchange', exchange_type='topic')
# Create the queues
channel.queue_declare(queue='queue1')
channel.queue_declare(queue='queue2')
# Bind queue1 to the topic exchange with a binding key of 'stock.*'
channel.queue_bind(exchange='topic_exchange', queue='queue1', routing_key='stock.*')
# Bind queue2 to the topic exchange with a binding key of '*.usd.*'
channel.queue_bind(exchange='topic_exchange', queue='queue2', routing_key='*.usd.*')
# Publish a message with the routing key 'stock.usd.nasdaq'
channel.basic_publish(exchange='topic_exchange', routing_key='stock.usd.nasdaq', body='Hello, queue1!')
# Publish a message with the routing key 'market.eur.nasdaq'
channel.basic_publish(exchange='topic_exchange', routing_key='market.eur.nasdaq', body='Hello, queue2!')
connection.close()
In this example, we declare a topic exchange named 'topic_exchange'. We create two queues named 'queue1' and 'queue2'.
We then bind 'queue1' to the exchange with a binding key of 'stock.*'. This means that 'queue1' will receive messages with routing keys starting with 'stock' (e.g., 'stock.usd', 'stock.eur', etc.).
We also bind 'queue2' to the exchange with a binding key of '*.usd.*'. This means that 'queue2' will receive messages with routing keys containing 'usd' anywhere in the key and any number of words on either side (e.g., 'stock.usd.nasdaq', 'market.usd.ibm', etc.).
Finally, we publish two messages with different routing keys. The message with the routing key 'stock.usd.nasdaq' matches the binding key of 'queue1', so it will be delivered to 'queue1'. The message with the routing key 'market.eur.nasdaq' does not match any of the binding keys, so it will not be delivered to any queue.
The topic exchange is useful when you want to route messages based on multiple criteria or patterns. It provides a flexible and powerful way to implement sophisticated message routing in your applications.
Headers Exchange
The headers exchange uses message headers instead of routing keys to determine which queues to deliver messages to. It allows you to match based on multiple header values and datatypes.
Here's an example of how the headers exchange works:
import pika
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
channel.exchange_declare(exchange='headers_exchange', exchange_type='headers')
# Create the queue
channel.queue_declare(queue='queue1')
# Bind the queue to the headers exchange with header patterns
channel.queue_bind(exchange='headers_exchange', queue='queue1', arguments={'x-match': 'all', 'type': 'stock', 'price': 100})
# Publish a message with headers
channel.basic_publish(exchange='headers_exchange', routing_key='', body='Hello, queue1!', properties=pika.BasicProperties(headers={'type': 'stock', 'price': 100}))
connection.close()
In this example, we declare a headers exchange named 'headers_exchange'. We create a queue named 'queue1' and bind it to the exchange with header patterns.
The headers exchange requires a match of all the specified header patterns, which are passed as arguments when binding the queue. In this case, we specify that the 'type' header must be 'stock' and the 'price' header must be 100.
When we publish a message to the headers exchange, we include the corresponding headers in the message properties. If the headers in the message match the header patterns, the message will be delivered to the bound queue.
The headers exchange is useful when you want to route messages based on custom header values and conditions. It provides a versatile way to implement complex routing logic in your applications.
Conclusion
Congratulations on gaining a comprehensive understanding of the different types of RabbitMQ exchanges: Direct, Fanout, Topic, and Headers exchanges. You now have the knowledge to design and implement efficient messaging architectures in your applications.
Remember, the choice of exchange type depends on your specific use case and requirements. Direct exchanges are suitable for point-to-point communication, fanout exchanges for publish-subscribe-type communication, topic exchanges for routing based on patterns, and headers exchanges for matching based on custom header values and conditions.
Experiment with different exchange types and explore their capabilities to unleash the full potential of RabbitMQ in your applications. Happy messaging!