Optimizing Performance with Zero Allocation in Golang
Learn how to optimize your Go applications by minimizing memory allocations. Discover techniques like object reuse, buffered channels, and byte slices for improved performance and reduced memory usage. Boost the efficiency and scalability of your code with these zero allocation tips.
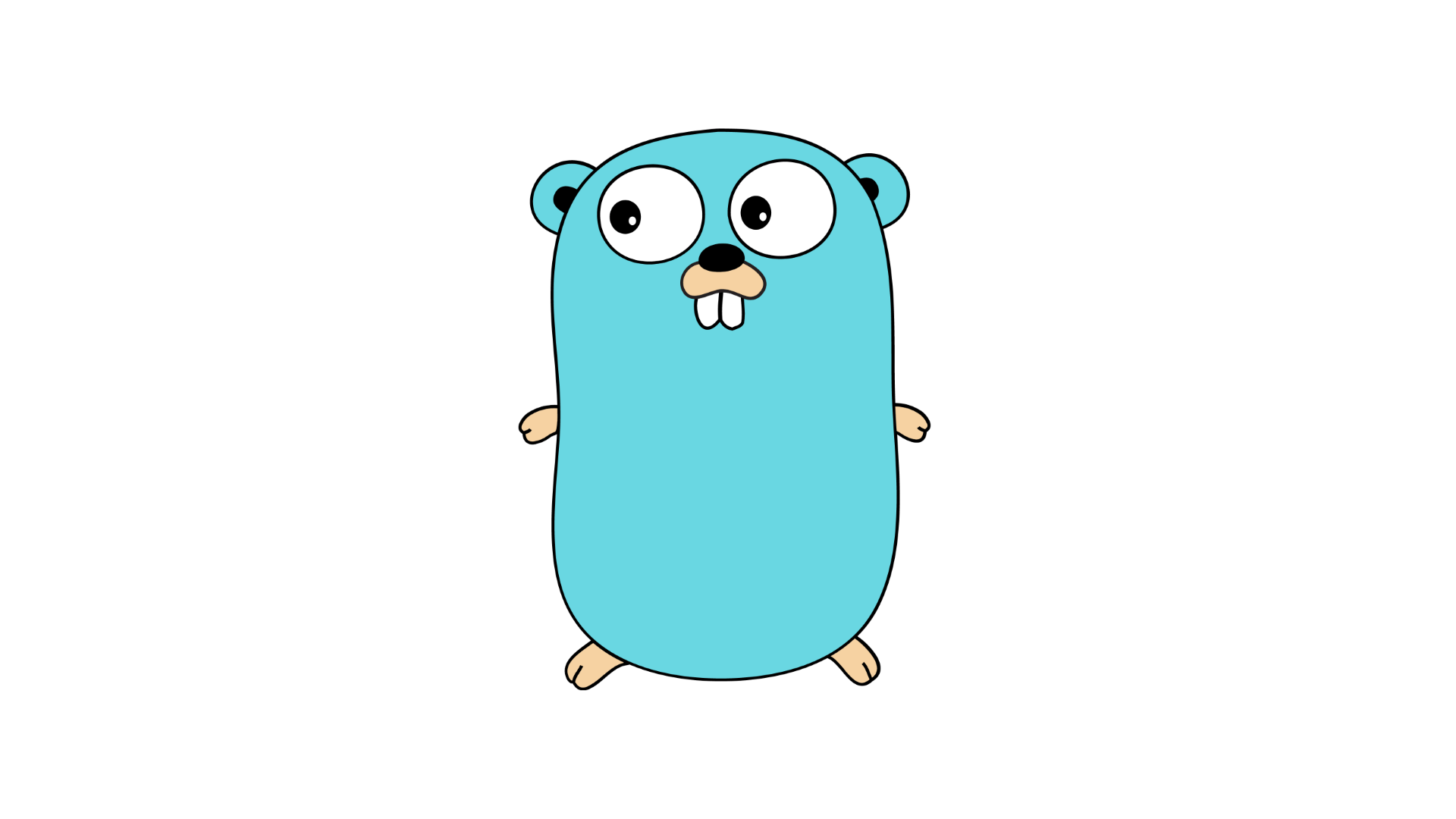
Introduction
Optimizing performance is a crucial aspect of any application development, and Go (Golang) provides several features and techniques to achieve that. One such technique is zero allocation, which refers to the practice of minimizing or eliminating memory allocations during runtime. By reducing allocations, you can improve the overall performance and efficiency of your Go applications.
What are Allocations?
In Go, an allocation occurs when memory is dynamically assigned to a variable or data structure. Allocations can happen for various operations, such as creating new objects, appending to slices, or concatenating strings. While allocations are necessary for many operations, excessive allocations can negatively impact performance and introduce unnecessary garbage collection overhead.
Benefits of Zero Allocation
Minimizing or eliminating allocations can have several benefits:
- Improved Performance: By reducing allocations, you can decrease the amount of work the garbage collector needs to perform, which in turn leads to faster execution times.
- Reduced Memory Pressure: Fewer allocations mean less memory is used, reducing the memory pressure on your application and potentially allowing it to handle larger workloads.
- Better Scalability: Applications that minimize allocations are more scalable, as they can handle a larger number of concurrent requests without running out of memory.
Techniques for Zero Allocation
Now let's dive into some techniques you can use to minimize or eliminate allocations in your Go code:
1. Use sync.Pool for Object Reuse
The sync.Pool
package provides a way to reuse objects instead of creating new ones. By recycling objects for future use, you can minimize memory allocations. Here's an example:
package main
import (
"fmt"
"sync"
)
type MyObject struct {
// fields
}
func main() {
pool := sync.Pool{
New: func() interface{} {
return &MyObject{}
},
}
obj := pool.Get().(*MyObject)
// use obj
fmt.Println(obj)
pool.Put(obj)
}
2. Use Buffered Channels for Goroutines
When using goroutines for concurrent execution, using buffered channels instead of unbuffered channels can reduce the number of allocations. Buffered channels allocate a fixed-sized buffer, which eliminates the need for dynamic memory allocation during message passing. Here's an example:
package main
import "fmt"
func worker(input chan string, output chan int) {
for {
data := <-input
// process data
result := len(data)
output <- result
}
}
func main() {
input := make(chan string)
output := make(chan int)
go worker(input, output)
for i := 0; i < 10; i++ {
input <- "data"
}
for i := 0; i < 10; i++ {
result := <-output
fmt.Println("Result:", result)
}
}
3. Use Byte Slice Instead of Strings for Concatenation
String concatenation using the +
operator can create new strings, resulting in allocations. Using a []byte
slice and the append
function can help reduce allocations. Once the concatenation is complete, you can convert the []byte
slice to a string using string(mySlice)
. Example:
package main
import "fmt"
func concatenateStrings(strs ...string) string {
var result []byte
for _, str := range strs {
result = append(result, []byte(str)...)
}
return string(result)
}
func main() {
result := concatenateStrings("Hello", " ", "World")
fmt.Println("Result:", result)
}
4. Use Struct Tags for Memory Alignment
Go structs are aligned in memory based on field order. By using struct tags to control the memory alignment, you can minimize or eliminate padding bytes, reducing memory consumption. Here's an example:
package main
import (
"fmt"
"unsafe"
)
type MyStruct struct {
Field1 int
Field2 int
}
type MyAlignedStruct struct {
Field1 int `json:"field1"`
Field2 int `json:"field2"`
}
func main() {
size1 := unsafe.Sizeof(MyStruct{}) // Size without alignment
size2 := unsafe.Sizeof(MyAlignedStruct{}) // Size with alignment
fmt.Println("Size without alignment:", size1)
fmt.Println("Size with alignment:", size2)
}
Conclusion
Optimizing performance with zero allocation in Go is important for achieving efficient and scalable applications. By applying techniques such as object reuse, buffered channels, byte slices, and memory alignment, you can minimize memory allocations and improve the overall performance of your Go applications. Remember to profile your code to identify potential bottlenecks and prioritize optimization efforts.
Happy coding!