Optimizing Performance in Golang: Tips and Tricks
Discover powerful tips and tricks to optimize the performance of your Golang applications. Boost concurrency, minimize memory usage, leverage compiled code, and more. Supercharge your code today!
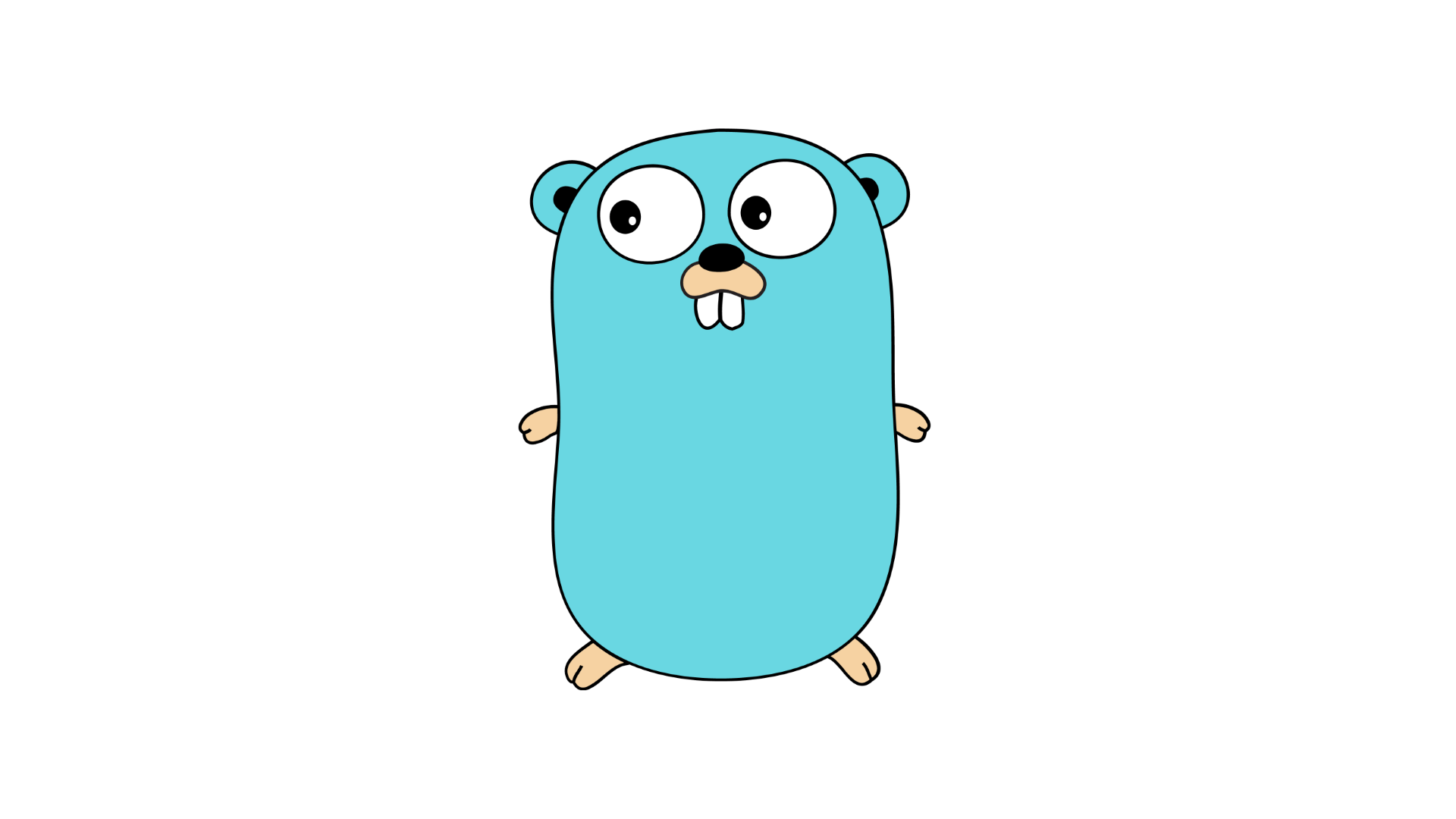
Optimizing Performance in Golang: Tips and Tricks
If you're new to Go (Golang) development, you may be wondering how to optimize the performance of your applications. In this guide, we'll explore some useful tips and tricks that can help you improve the performance of your Golang projects.
Introduction
Golang is known for its simplicity and efficiency, but there are always opportunities to optimize the performance of your code. By following best practices and leveraging some advanced techniques, you can significantly enhance the speed and efficiency of your Golang applications.
Use Concurrency
One of the key advantages of Go is its built-in support for concurrency. By utilizing Goroutines and channels, you can easily create concurrent code that can execute tasks in parallel. This can drastically improve the performance of your code by utilizing multiple CPU cores effectively.
Optimize Memory Usage
Golang has its own garbage collector that manages memory allocation and deallocation automatically. However, it's still important to be mindful of your memory usage to avoid unnecessary allocations and garbage collection pauses. Consider using sync.Pool for object reusability and minimizing memory allocations.
Use Compiled Code
Golang is a compiled language, and the Go compiler generates highly optimized machine code. To leverage the full performance potential of Go, it's important to compile your code and avoid interpreted execution. Use the 'go build' command to compile your code into an executable binary.
Profile Your Code
Go provides built-in tools for profiling your code to identify performance bottlenecks. Use the 'go test -bench' command to run benchmarks and measure the performance of your code. Additionally, the 'pprof' package can be used to generate detailed profiling reports for CPU and memory usage.
Use Buffers for I/O Operations
Performing I/O operations in small chunks can be relatively slow due to the overhead of system calls. To optimize I/O performance, use buffered readers and writers to read and write data in larger chunks. This can significantly reduce the number of system calls and improve overall performance.
Avoid Unnecessary Goroutine Creation
While Goroutines are lightweight, creating too many of them can still have an impact on performance. Avoid creating Goroutines unnecessarily and consider using worker pools or other patterns to limit the number of concurrent Goroutines and optimize resource utilization.
Use Efficient Data Structures
Choosing the right data structures can have a significant impact on performance. Use built-in data structures like maps, slices, and arrays when appropriate, as they are highly optimized in Go. Avoid unnecessary conversions and allocations to ensure optimal performance.
Avoid GC Pressures
The Go garbage collector (GC) can cause occasional pauses in your application. To minimize the impact of the GC on performance, avoid creating too many short-lived objects and unnecessary allocations. Use tools like the 'gcflags' command-line option to fine-tune the GC behavior.
Conclusion
Optimizing the performance of your Golang applications is crucial for delivering fast and efficient software. By following these tips and tricks, you can significantly boost the speed and efficiency of your code. Experiment with different optimization techniques and monitor the performance to find the best approach for your specific use cases.
Remember, performance optimization is an ongoing process, and it's important to continuously measure, analyze, and improve your code to ensure optimal performance in the long run.