Optimizing gRPC for Low Latency and High Throughput
Learn how to optimize gRPC for low latency and high throughput. Explore best practices for using protocol buffers, enabling compression, leveraging streaming, tuning RPC settings, using load balancing, and monitoring performance. Achieve optimal performance in your gRPC applications.
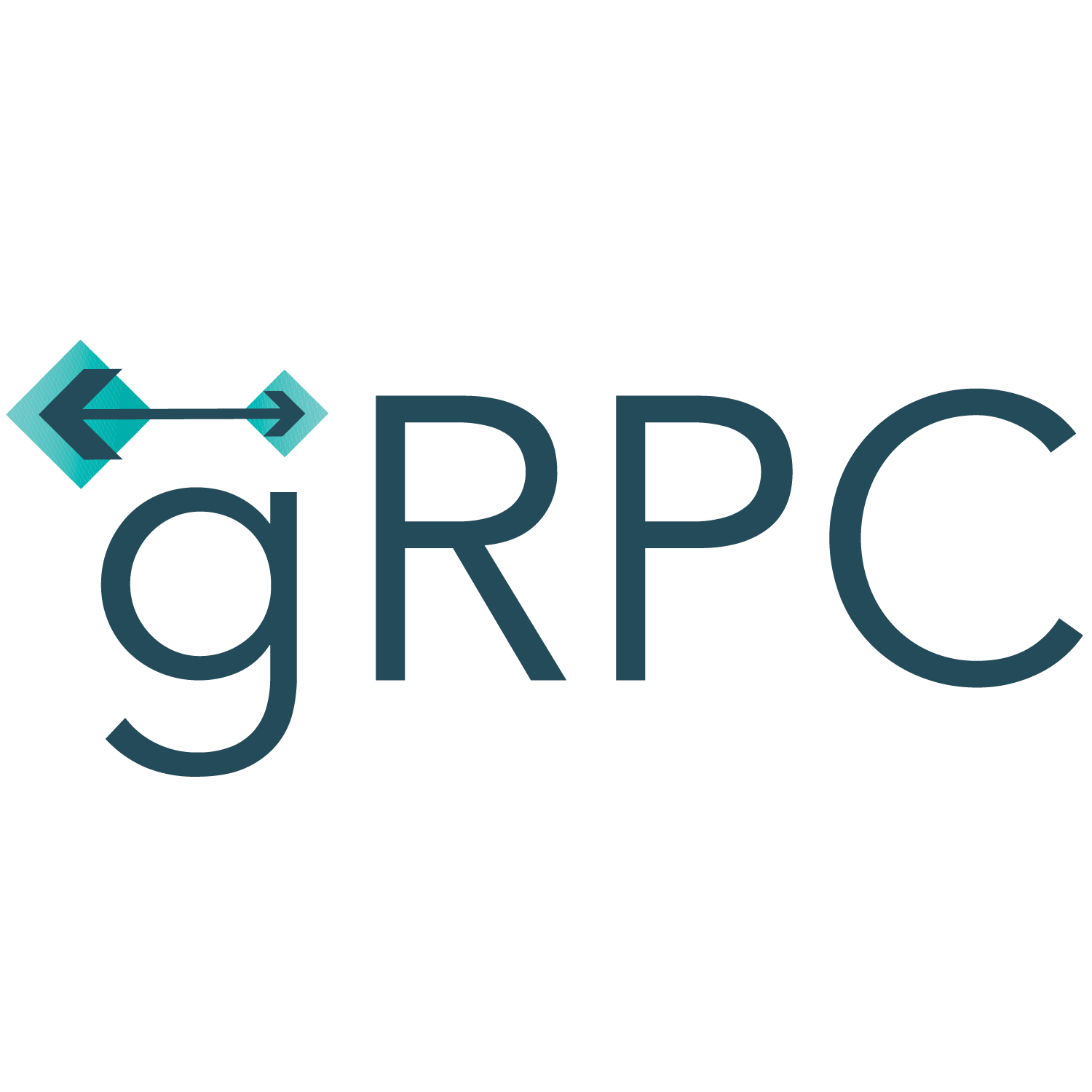
Introduction
gRPC is a high-performance, open-source framework that enables efficient communication between client and server applications. It is widely used in microservices architectures and distributed systems due to its support for various programming languages and platforms.
When building applications that require low latency and high throughput, it is crucial to optimize gRPC to ensure optimal performance. In this blog post, we will explore some best practices and techniques to optimize gRPC and achieve low latency and high throughput in your applications.
1. Use Protocol Buffers
Protocol Buffers, also known as "protobufs," are a language-agnostic binary serialization format used by gRPC. By using protobufs, you can reduce the payload size sent over the network, resulting in improved performance.
Protobufs provide a compact binary format that is more efficient than JSON and XML. Additionally, they support features like schema evolution, allowing you to add or remove fields without breaking compatibility with existing clients or servers.
To use protobufs with gRPC, define your service and message definitions in a .proto
file. Then, use the protoc
compiler to generate code for your chosen programming language. This code will provide strongly-typed APIs and serialization/deserialization functions for your gRPC services.
2. Enable Compression
gRPC supports various compression algorithms, such as gzip and zlib, to reduce the size of data transmitted over the network. Enabling compression can significantly improve performance, especially when dealing with large payloads.
To enable compression in gRPC, you need to configure the server and client to use compression algorithms supported by gRPC. Both the server and client should specify the desired compression algorithm in their configuration.
For example, in Go, you can enable gzip compression on the server side using the following code:
import "google.golang.org/grpc/encoding"
encoding.RegisterCompressor(func() encoding.Compressor {
return encoding.Gzip
})
Similarly, on the client side, you can enable compression using the following code:
import "google.golang.org/grpc"
conn, _ := grpc.Dial(
address,
grpc.WithDefaultCallOptions(
grpc.UseCompressor(encoding.Gzip),
),
)
3. Use Streaming
gRPC supports two types of streaming: Unary and Streaming. Unary RPCs are like traditional remote procedure calls, where the client sends a request and the server sends back a response. Streaming RPCs, on the other hand, allow the client and server to send a stream of messages back and forth.
Using streaming can improve performance and throughput in scenarios where the client or server needs to send or receive multiple messages. Instead of making multiple round trips, streaming allows you to send or receive data in batches, reducing latency and minimizing network overhead.
To use streaming in gRPC, define your service methods as either Unary
or Streaming
. For example, a unary method would have the signature rpc MyMethod(MyRequest) returns (MyResponse);
, while a streaming method would have the signature rpc MyMethod(stream MyRequest) returns (stream MyResponse);
.
4. Tune RPC Settings
gRPC provides various settings that you can tune to optimize performance for your use case. These settings control aspects such as message size limits, connection pooling, and flow control.
For example, you can configure the maximum message size allowed to be sent or received by modifying the grpc.MaxCallRecvMsgSize
and grpc.MaxCallSendMsgSize
options. Setting appropriate limits can prevent resource exhaustion and improve overall performance.
Additionally, you can adjust the size of the channel pools for better resource utilization. By default, gRPC maintains a pool of idle connections to reuse for subsequent requests. Modifying the grpc.MaxConnAge
and grpc.MaxConcurrentStreams
options can help control the size of the connection pool and optimize connection reuse.
5. Use Load Balancing
In distributed systems, load balancing is essential for achieving high availability and distributing incoming requests across multiple servers. gRPC provides built-in support for load balancing by allowing you to specify multiple backend servers for a gRPC service.
By using load balancing, you can distribute the workload among multiple servers, enabling horizontal scaling and reducing the chances of a single server becoming a performance bottleneck.
gRPC supports various load balancing strategies, including round-robin, weighted round-robin, and least connection. You can choose the appropriate load balancing strategy based on your application's requirements.
To configure load balancing in gRPC, use a grpc.Balancer
implementation that supports your desired load balancing strategy. Then, pass the grpc.WithBalancer()
option when creating a gRPC connection (client) to specify the load balancing strategy for that connection.
6. Monitor Performance
Monitoring the performance of your gRPC applications is crucial to identify bottlenecks and optimize your system. By collecting and analyzing performance metrics, you can gain insights into the behavior of your applications and make informed optimizations.
gRPC provides built-in support for metrics and monitoring, allowing you to collect and expose performance statistics to monitoring systems like Prometheus or Grafana. You can instrument your gRPC services to track metrics such as request latency, error rates, and throughput.
Choose a metrics library that integrates well with gRPC, such as OpenTelemetry, and configure it to collect relevant performance data. Then, use a monitoring system to visualize and analyze the collected metrics, enabling you to identify performance bottlenecks and make informed decisions for optimizations.
Conclusion
Optimizing gRPC for low latency and high throughput is crucial for building performant applications. By following the best practices and techniques discussed in this blog post, you can achieve better performance and scalability in your gRPC applications.
Remember to use protocol buffers to reduce payload size, enable compression to minimize network overhead, leverage streaming to improve throughput, tune RPC settings for your use case, use load balancing for scalability, and monitor performance metrics to identify bottlenecks.
By continuously monitoring and optimizing your gRPC applications, you'll ensure that they perform optimally and provide efficient communication between client and server components.
Happy optimizing!