Deep Dive into Zero Allocation in Golang: Best Practices and Tips
Learn how to minimize memory allocations and optimize the performance of your Go applications. Explore best practices and tips for zero allocation in Go.
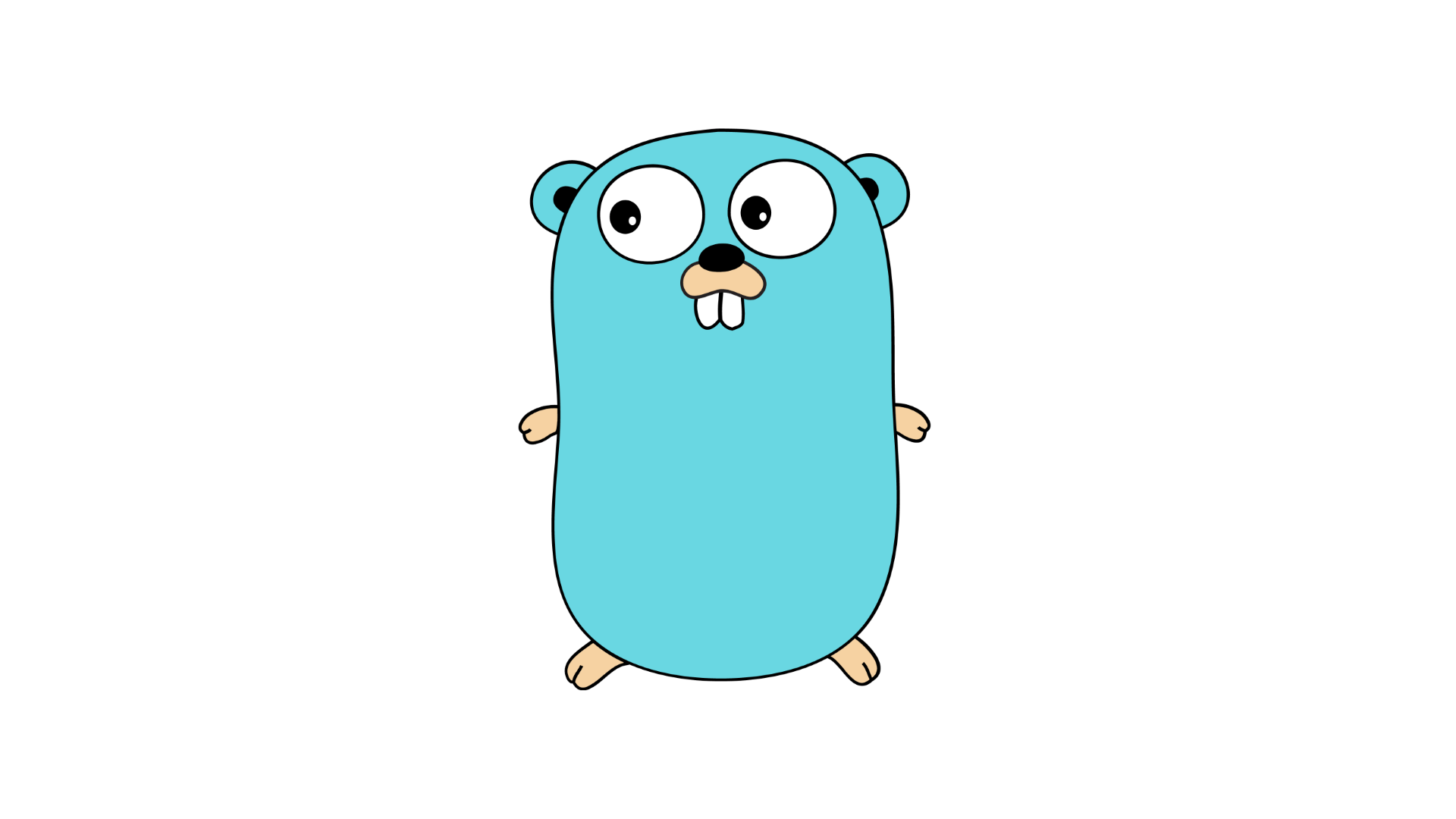
Introduction
As a Go developer, understanding the concept of zero allocation is crucial for writing high-performance, memory-efficient code. In this deep dive into zero allocation in Go, we will explore the best practices and tips to minimize memory allocations and optimize the performance of your Go applications. By following these techniques, you can reduce garbage collection overhead and improve the overall response time of your applications. Let's dive in!
What is Zero Allocation?
In Go, zero allocation refers to the practice of minimizing memory allocations performed by your application. When a program allocates memory, it increases the pressure on the garbage collector to free up memory that is no longer in use. Minimizing these allocations can lead to improved performance and reduced memory consumption.
1. Use the sync.Pool
The sync.Pool package in Go provides a mechanism to reuse allocated objects instead of creating new ones. It is especially useful for scenarios where you frequently allocate and deallocate objects, such as in concurrent programming. By reusing objects from the sync.Pool, you can significantly reduce memory allocations and improve the overall performance of your application.
Here is an example of using the sync.Pool to manage objects:
package main
import (
"fmt"
"sync"
)
type Person struct {
Name string
}
var pool = sync.Pool{
New: func() interface{} {
return &Person{}
},
}
func main() {
p := pool.Get().(*Person)
defer pool.Put(p)
p.Name = "John Doe"
fmt.Println(p.Name)
}
In this example, we define a Person struct and a sync.Pool that manages instances of Person. We use the Get() and Put() methods of the sync.Pool to get and return instances from the pool. By reusing instances from the pool, we minimize memory allocations and improve the performance of our application.
2. Use Byte Slices Instead of Strings
In Go, strings are immutable, which means that every time you perform an operation on a string (such as concatenation or substring extraction), a new string is allocated in memory. This can lead to unnecessary memory allocations and increased garbage collection overhead.
One way to mitigate this is by using byte slices instead of strings, especially in performance-critical sections of your code. Byte slices are mutable, meaning you can modify them without allocating new memory.
Here's an example that demonstrates the use of byte slices:
package main
import (
"fmt"
)
func main() {
str := "Hello, World!"
bytes := []byte(str)
bytes[7] = 'G'
fmt.Println(string(bytes))
}
In this example, we convert the string "Hello, World!" to a byte slice using the []byte() conversion. We then modify the element at index 7 to 'G'. Since byte slices are mutable, no memory allocation is required.
3. Avoid Concatenating Strings Using Sprintf or Operators
When concatenating strings in Go using the fmt.Sprintf() function or the + operator, a new string is created, resulting in memory allocation. This can be a performance bottleneck, especially when concatenating strings in a loop or in frequently executed code paths.
A more efficient approach is to use the bytes.Buffer type from the bytes package. The bytes.Buffer type provides a buffer that can be used to efficiently build and manipulate strings without unnecessary memory allocations.
Here is an example that demonstrates the use of bytes.Buffer:
package main
import (
"fmt"
"bytes"
)
func main() {
var buffer bytes.Buffer
for i := 0; i < 1000; i++ {
buffer.WriteString("Hello, ")
buffer.WriteString("World!")
}
fmt.Println(buffer.String())
}
In this example, we create a bytes.Buffer and use the WriteString() method to efficiently build the final string. By using a buffer, we minimize memory allocations and improve the performance of our application.
4. Use Optimized Data Structures
The choice of data structure can have a significant impact on memory allocation and performance. Using optimized data structures can help minimize memory allocations and improve the efficiency of your code.
For example, if you frequently perform lookups or insertions in a collection, using a map instead of a slice can be more efficient. Maps in Go are implemented as hash tables, which provide fast lookup and insertion operations.
Here is an example that demonstrates the use of a map:
package main
import (
"fmt"
)
func main() {
data := map[int]string{
1: "one",
2: "two",
3: "three",
}
value, exists := data[2]
if exists {
fmt.Println(value)
}
}
In this example, we use a map to store key-value pairs. Maps provide fast lookup operations, reducing the need for memory allocations and improving the performance of our application.
5. Use Declare-Only Variables for Empty Interfaces
Empty interfaces (interface{}) are a powerful construct in Go that allows you to work with values of any type. However, empty interfaces can result in memory allocations if not used carefully.
One way to minimize memory allocations when working with empty interfaces is to use declare-only variables. Declare-only variables are variables without an explicit initial value, which means that memory is not allocated for the value until it is assigned.
Here is an example that demonstrates the use of declare-only variables:
package main
import (
"fmt"
)
func main() {
var data interface{}
// Use data
data = 42
// Use data
data = "Hello, World!"
// Use data
fmt.Println(data)
}
In this example, we declare a variable data
of type interface{}
without an initial value. The memory for the value is only allocated when we assign a value to data
. By using declare-only variables, we can avoid unnecessary memory allocations when working with empty interfaces.
6. Use Pointers Instead of Values
In Go, passing values between functions can result in memory copies, which can lead to unnecessary memory allocations. To avoid this, you can use pointers instead of values. Pointers allow you to work with the memory address of a variable instead of its value.
Here is an example that demonstrates the use of pointers:
package main
import (
"fmt"
)
func modifyValue(value *int) {
*value = 42
}
func main() {
var value int
modifyValue(&value)
fmt.Println(value)
}
In this example, we define a function modifyValue
that takes a pointer to an int
. By passing &value
to the modifyValue
function, we modify the value of value
directly through the pointer. This allows us to avoid unnecessary memory allocations when passing values between functions.
Wrapping Up
Congratulations on completing this deep dive into zero allocation in Go! By following the best practices and tips outlined in this article, you can minimize memory allocations and optimize the performance of your Go applications.
Remember to use the sync.Pool to reuse allocated objects, prefer byte slices over strings, avoid concatenating strings using Sprintf or operators, use optimized data structures, use declare-only variables for empty interfaces, and use pointers instead of values when passing variables between functions.
By incorporating these techniques into your development workflow, you'll write more efficient and performant Go code. Happy coding!