How to Model Complex Business Domains using Golang and Domain-Driven Design
Learn how to model complex business domains using Go and Domain-Driven Design (DDD). Understand key concepts, design principles, and best practices for effective and maintainable software development.
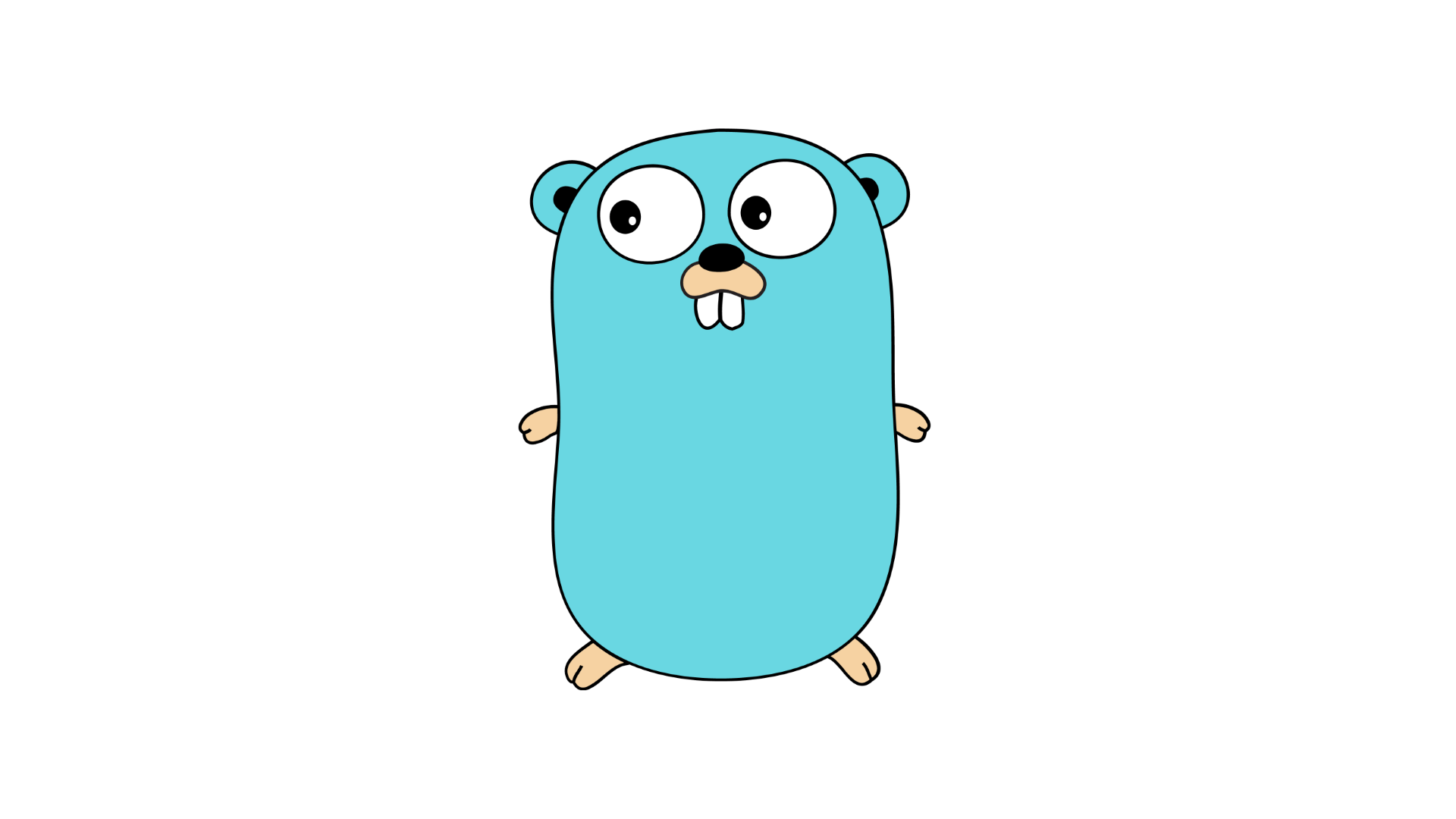
Introduction
When developing software applications, it's essential to have a clear understanding of the business domain. Modeling complex business domains can be a challenging task, but with the right approach, it becomes much more manageable. In this tutorial, we'll explore how to model complex business domains using Go (Golang) and Domain-Driven Design (DDD). We'll cover the key concepts and techniques that will help you effectively analyze and represent your business requirements in code. Let's get started!
What is Domain-Driven Design?
Domain-Driven Design (DDD) is an approach to software development that focuses on building applications based on a deep understanding of the business domain. It promotes collaboration between domain experts and developers to create an effective and maintainable software system.
DDD encourages modeling the business domain using domain concepts, entities, aggregates, value objects, services, and repositories. These concepts help to capture the essence of the business domain and represent it in a clear and expressive manner.
The Importance of Modeling Complex Business Domains
Modeling complex business domains is crucial for several reasons:
- Accurate representation: By modeling the business domain, you can accurately represent the various rules, relationships, and constraints of the domain.
- Effective communication: A well-modeled domain provides a common language that both technical and non-technical stakeholders can understand, facilitating effective communication.
- Maintainability: A well-designed domain model makes the codebase easier to understand, refactor, and maintain as it reflects the structure and behavior of the business domain.
- Flexibility: A well-modeled domain allows for easier adaptation to changing business requirements, as the changes can be made at the domain level.
Key Concepts of Domain-Driven Design
Before we dive into modeling complex business domains using Go and DDD, let's briefly understand the key concepts of DDD:
1. Domain
The domain represents the subject matter or the problem space of the software system. It consists of the business rules, processes, and concepts that are unique to the specific problem domain.
2. Domain Model
A domain model is a representation of the real-world concepts and relationships in the business domain. It captures the essential business logic and behaviors of the domain and serves as a bridge between the business stakeholders and the technical implementation.
3. Aggregates
Aggregates are cohesive clusters of related objects that are treated as a single unit during data changes. They ensure consistency and encapsulation of business rules within the domain model.
4. Value Objects
Value objects represent concepts that have no identity beyond their attributes. They are immutable objects that are used to encapsulate and operate on a specific set of values.
5. Entities
Entities represent objects that have unique identities and go through different life-cycle states. They define the behavior and attributes that are relevant to the business domain.
6. Services
Services encapsulate operations or business logic that doesn't naturally belong to any specific entity or value object. They represent behaviors or operations that are essential to the business domain but don't have an identity of their own.
7. Repositories
Repositories are responsible for persisting and retrieving aggregates from the underlying data store. They provide a clean abstraction for data access and encapsulate the details of data storage and retrieval.
Modeling a Complex Business Domain in Go
Now that we have a solid understanding of DDD concepts, let's explore how to model a complex business domain using Go:
1. Understand the Business Domain
The first step in modeling a complex business domain is to gain a deep understanding of the domain itself. Collaborate with domain experts, stakeholders, and users to gather requirements and define the core concepts, rules, and processes that drive the domain.
2. Identify Aggregates, Value Objects, and Entities
Once you have a clear understanding of the business domain, identify the aggregates, value objects, and entities within the domain. Aggregates are the root objects that encapsulate other related objects, while value objects and entities represent concepts within the domain.
3. Design the Domain Model
Design the domain model by creating Go structs that represent the aggregates, value objects, and entities identified in the previous step. Define the attributes, behaviors, and relationships of each domain concept.
4. Encapsulate Business Logic in Aggregates and Entities
Encapsulate the business logic within the aggregates and entities. Define methods and functions that enforce the domain rules and constraints. Use data validation techniques to ensure the integrity and consistency of the domain model.
5. Implement Services for Cross-Cutting Operations
Implement services to encapsulate cross-cutting operations or business logic that doesn't naturally belong to any specific entity or value object. Use interfaces to define service contracts, allowing for flexibility and testability.
6. Create Repositories for Data Persistence
Create repositories to handle the persistence and retrieval of aggregates from the underlying data store. Implement methods for saving, updating, and querying aggregates, while abstracting away the details of the data storage technology.
Applying DDD with Go Best Practices
Here are some best practices to consider when applying DDD with Go:
1. Use Idiomatic Go Code
Write code that follows the Go idioms and best practices. Use interfaces, structs, and functions to represent the domain concepts, and leverage Go's strong typing to ensure type safety in your domain model.
2. Keep the Domain Model Pure
Avoid introducing external dependencies or technical concerns into the domain model. Keep it focused on representing the business domain and keep infrastructure-related code, such as persistence or UI concerns, separate from the domain code.
3. Use Unit Tests for Domain Logic
Write comprehensive unit tests for the domain logic to ensure it behaves correctly. Test the business rules, constraints, and behaviors of the aggregates, value objects, and entities. Use test doubles, such as mocks, to isolate the domain code from external dependencies.
4. Apply Simplified Data Access Patterns
Use simple data access patterns like repositories to handle persistence and retrieval of aggregates. Keep the repositories focused on the domain model and avoid introducing complex query mechanisms or leaky abstractions.
Conclusion
Modeling complex business domains using Go and Domain-Driven Design is essential for building effective and maintainable software systems. By understanding and applying the key concepts of DDD, you can accurately represent the business requirements, improve communication with stakeholders, and maintain flexibility in your codebase. Keep practicing and refining your skills in modeling complex domains, and you'll be well on your way to becoming a proficient domain-driven developer with Go!
Let's embrace the power of DDD and Go to build robust and elegant software solutions. Happy coding!