The Importance of Zero Allocation in Golang: A Performance Perspective
"Inefficient memory allocation can lead to poor performance in Go. Learn why zero allocation matters and techniques to minimize allocations for high-performance code."
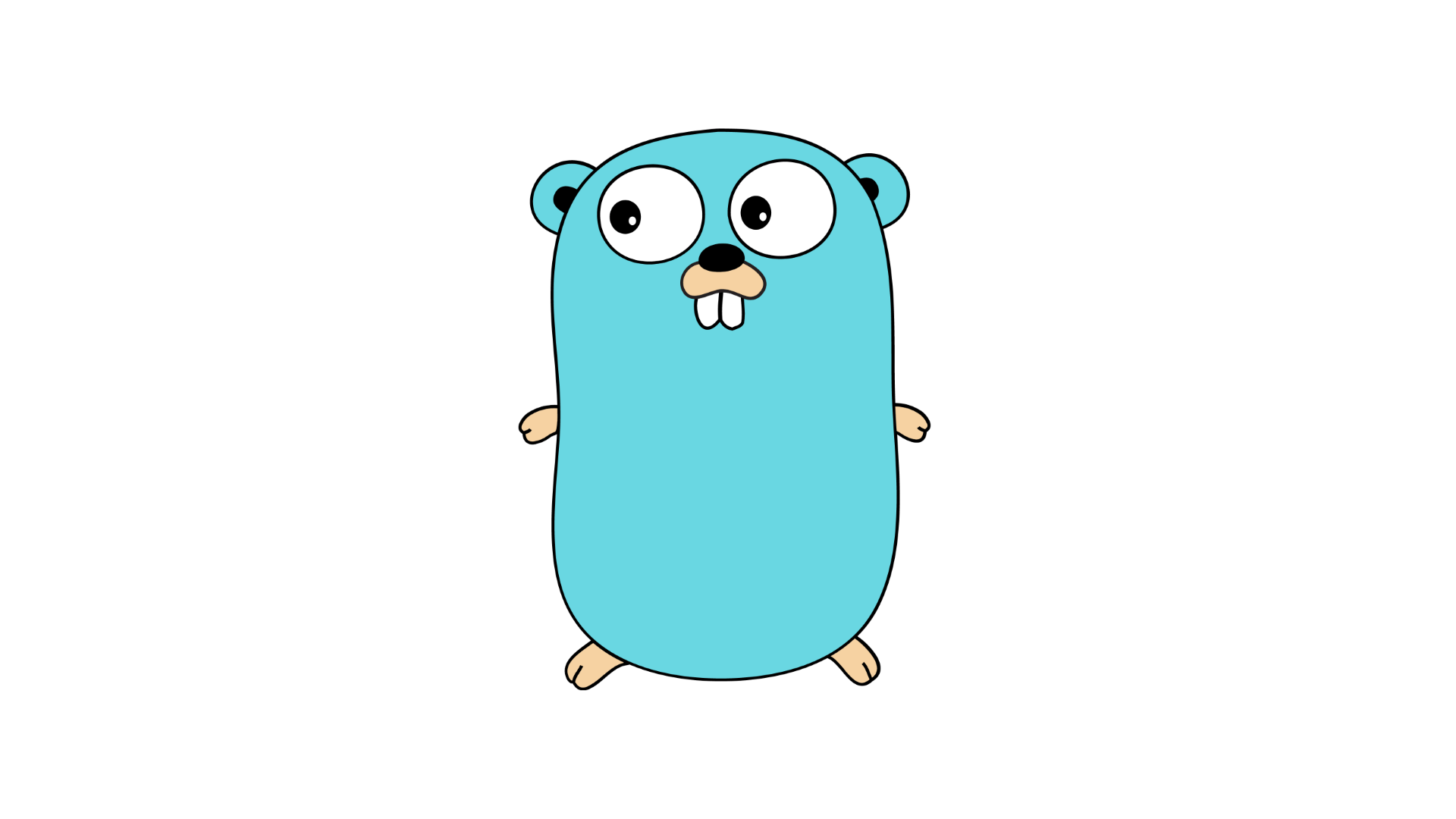
Introduction
When it comes to writing high-performance Go (Golang) code, understanding memory allocation and management is crucial. Inefficient memory allocation can lead to poor performance and inefficient resource utilization, which can hamper the overall speed and efficiency of your application.
In this article, we'll explore the importance of zero allocation in Go from a performance perspective. We'll discuss what zero allocation means, why it matters, and how you can optimize your code to minimize unnecessary allocations. Let's dive in!
What is Zero Allocation?
In Go, zero allocation refers to the process of minimizing or completely eliminating memory allocations during the execution of your program. When you allocate memory, you create new objects or data structures in memory to store the necessary information. However, memory allocation and deallocation can be expensive operations, especially when done frequently or in large quantities.
Zero allocation techniques aim to reduce or eliminate the need for allocating additional memory by reusing existing memory or using alternative data structures. By doing so, you can significantly improve the performance and efficiency of your Go code.
Why is Zero Allocation Important?
There are several reasons why zero allocation is important for optimizing Go code:
1. Reducing Garbage Collection Pressure
Garbage collection is the process of reclaiming memory that is no longer in use by your program. When your code allocates new memory frequently, it increases the workload for the garbage collector, which can lead to performance overhead and slowdowns. By minimizing memory allocations, you reduce the pressure on the garbage collector, resulting in improved performance and responsiveness of your application.
2. Improving Cache Locality
CPU caches are small but extremely fast memory units that store frequently accessed data. When you allocate memory frequently, it can cause cache thrashing, where the CPU constantly fetches data from the main memory to the cache, degrading performance. By minimizing allocations, you increase the chances of reusing existing data in the cache, which improves cache locality and speeds up your code execution.
3. Avoiding Garbage Collection Pauses
During garbage collection, Go halts the execution of your program to perform memory cleanup. These pauses can be disruptive to the performance and responsiveness of your application, especially when they occur frequently or for longer durations. By minimizing memory allocations, you reduce the frequency and duration of garbage collection pauses, leading to a smoother and more efficient application.
4. Reducing Memory Usage
By avoiding unnecessary allocations, you can reduce the overall memory footprint of your program. This is particularly important in resource-constrained environments where memory is limited. Minimizing memory usage improves efficiency and allows your application to run smoothly even with limited resources.
Techniques for Minimizing Memory Allocations
Now that we understand the importance of zero allocation in Go, let's explore some techniques for minimizing memory allocations:
1. Use sync.Pool for Object Reuse
The sync.Pool
package provides a way to reuse objects without the need for reallocation. By pooling frequently accessed objects, you can avoid unnecessary allocations and deallocations, improving performance and reducing garbage collection pressure.
import "sync"
type MyObject struct {
// Define your object fields here
}
func main() {
myPool := &sync.Pool{
New: func() interface{} {
return &MyObject{}
},
}
// Get an object from the pool
obj := myPool.Get().(*MyObject)
// Use the object
// Return the object to the pool
myPool.Put(obj)
}
2. Use Byte Slices Instead of Strings
Go strings are immutable, which means every time you modify a string, a new string is created in memory. By using byte slices instead, you can modify the content directly without incurring the overhead of creating new strings.
import "fmt"
func main() {
str := "Hello, World!"
// Convert the string to a byte slice
bytes := []byte(str)
// Modify the byte slice
bytes[7] = 'G'
// Convert the byte slice back to a string
modifiedStr := string(bytes)
fmt.Println(modifiedStr) // Output: Hello, Gorld!
}
3. Use Buffered I/O for I/O Operations
When performing I/O operations, such as reading or writing to a file or network, buffering can significantly reduce the number of system calls and memory allocations. By using buffered I/O, you can batch multiple I/O operations into a single system call, improving performance and reducing context switches.
import (
"fmt"
"os"
"bufio"
)
func main() {
file, err := os.Open("data.txt")
if err != nil {
panic(err)
}
defer file.Close()
// Create a buffered reader
reader := bufio.NewReader(file)
// Read the file line by line
for {
line, err := reader.ReadString('\n')
if err != nil {
break
}
// Process the line
fmt.Println(line)
}
}
Conclusion
Optimizing memory allocation is a critical aspect of writing high-performance Go code. By minimizing unnecessary memory allocations, you can improve the performance, responsiveness, and resource utilization of your applications. Use the techniques and best practices mentioned in this article to write efficient and performant Go code. Happy coding!