Golang Heap Allocation: Minimizing with Zero Allocation Techniques
Heap allocation impacts performance and memory usage in Go. Learn how to minimize heap allocations and optimize your Go code for efficiency.
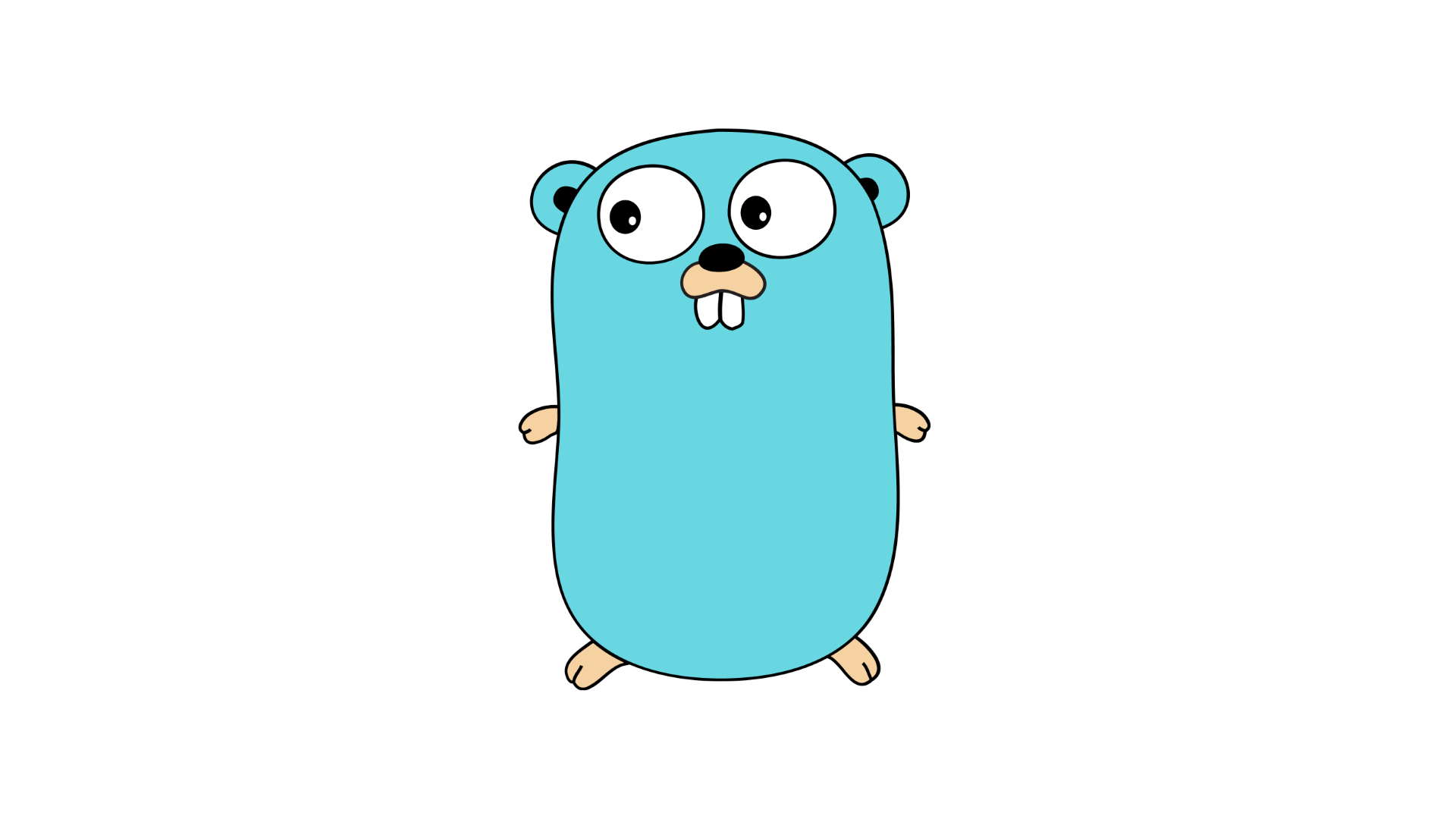
Introduction
Heap allocation is an important aspect of memory management in any programming language, including Go (Golang). Allocating memory on the heap can have a significant impact on your application's performance and efficiency. Excessive heap allocations can lead to increased memory usage, longer garbage collection pauses, and decreased overall application speed.
In this blog post, we'll explore various techniques to minimize heap allocations in Go. We'll learn how to use zero allocation techniques effectively to reduce memory usage, optimize performance, and improve the overall efficiency of your Go programs. Let's dive in and discover how to allocate memory like a pro!
The Concept of Heap Allocation
Before we dive into minimizing heap allocations, let's quickly understand the concept of heap allocation in Go. When you create a new variable or data structure in Go, it is typically allocated on the heap or the stack.
The stack is a region of memory that stores variables and data structures in a last-in-first-out (LIFO) manner. It is generally faster to allocate and deallocate memory on the stack, but it has limited space and a fixed size.
On the other hand, the heap is a larger region of memory that can dynamically allocate and deallocate memory. Variables and data structures allocated on the heap have a longer lifespan and can be accessed from different parts of your program. However, allocating memory on the heap is slower and requires garbage collection to free up unused memory.
Common Heap Allocation Scenarios
Understanding common scenarios that trigger heap allocations can help you identify areas where you can optimize your code. Here are some common scenarios that result in heap allocations:
- Creating new instances of structs
- Concatenating strings using the plus (+) operator
- Appending elements to slices
- Using the
append
function to concatenate slices - Passing arguments by value
These scenarios often lead to unnecessary heap allocations, which can be fine-tuned to improve performance and memory usage. Let's explore some techniques to minimize heap allocations in each of these scenarios.
Minimizing Heap Allocations
1. Reusing Variable Memory
One of the simplest methods to avoid heap allocations is by reusing variables or data structures. Instead of creating new instances every time you need them, reuse existing variables and update their values as necessary. This not only reduces heap allocations but also improves performance by avoiding unnecessary memory allocation and deallocation overhead.
For example, if you have a slice that you need to populate with new values, you can clear the slice and append new elements to it instead of creating a new slice each time:
// Bad approach: Creates a new slice each time
func getValues() []int {
return []int{1, 2, 3}
}
// Good approach: Reuses an existing slice
var values []int
func getValues() []int {
values = values[:0] // Clear the existing slice
return append(values, 1, 2, 3) // Append new elements
}
2. Using byte.Buffer for String Concatenation
String concatenation using the plus (+) operator can result in frequent heap allocations. To avoid this, you can use the bytes.Buffer
type to efficiently concatenate strings without allocating new memory on the heap:
// Bad approach: String concatenation with the plus operator
func concatenateStrings(firstName, lastName string) string {
return "Hello, " + firstName + " " + lastName
}
// Good approach: String concatenation with bytes.Buffer
import "bytes"
func concatenateStrings(firstName, lastName string) string {
var buffer bytes.Buffer
buffer.WriteString("Hello, ")
buffer.WriteString(firstName)
buffer.WriteByte(' ')
buffer.WriteString(lastName)
return buffer.String()
}
Using bytes.Buffer
minimizes heap allocations by reusing the underlying byte slice, resulting in improved performance and reduced memory usage.
3. Pooling
The sync.Pool
type in Go provides a way to create and manage a pool of reusable objects. By using object pooling, you can reduce heap allocations by reusing allocated objects instead of creating new ones.
Here's an example of how to use sync.Pool
to minimize heap allocations:
import "sync"
// Define a struct type to be pooled
type MyStruct struct {
// Struct fields
}
// Create a pool of reusable MyStruct objects
var myStructPool = sync.Pool{
New: func() interface{} {
return &MyStruct{}
},
}
// Get a MyStruct object from the pool
func getObjectFromPool() *MyStruct {
obj := myStructPool.Get().(*MyStruct)
// Reset the object
// ...
return obj
}
// Put a MyStruct object back into the pool
func putObjectBackToPool(obj *MyStruct) {
// Reset the object
// ...
myStructPool.Put(obj)
}
By utilizing pooling, you can minimize heap allocations by reusing objects and reduce the load on the garbage collector.
4. Passing Arguments by Pointer
Go supports passing arguments by value and by pointer. When passing large structures or slices, passing them by value can result in unnecessary heap allocations. To avoid this, consider passing the arguments by pointer instead, allowing you to modify the original object without creating a new copy:
// Bad approach: Passing a slice by value
func processSlice(slice []int) {
// Modify the slice
// ...
}
// Good approach: Passing a slice by pointer
func processSlice(slice *[]int) {
// Modify the slice using pointers
// ...
}
By passing arguments by pointer, you can avoid unnecessary heap allocations and improve performance when dealing with large data structures.
Conclusion
By minimizing heap allocations, you can optimize the memory usage, improve the performance, and make your Go programs more efficient. The techniques we explored in this blog post, such as reusing variable memory, using bytes.Buffer
for string concatenation, pooling, and passing arguments by pointer, can help you reduce heap allocations and enhance the overall efficiency of your Go code.
Remember, understanding the impact and minimizing heap allocations is crucial for any Go developer. By following these zero allocation techniques, you'll be on your way to building high-performance Go applications!