Golang Heap Allocation: Minimizing with Zero Allocation Techniques
"Learn how to minimize heap allocation in Golang with zero allocation techniques such as sync.Pool, byte slice pooling, and buffer pooling. Reduce overhead and boost performance."
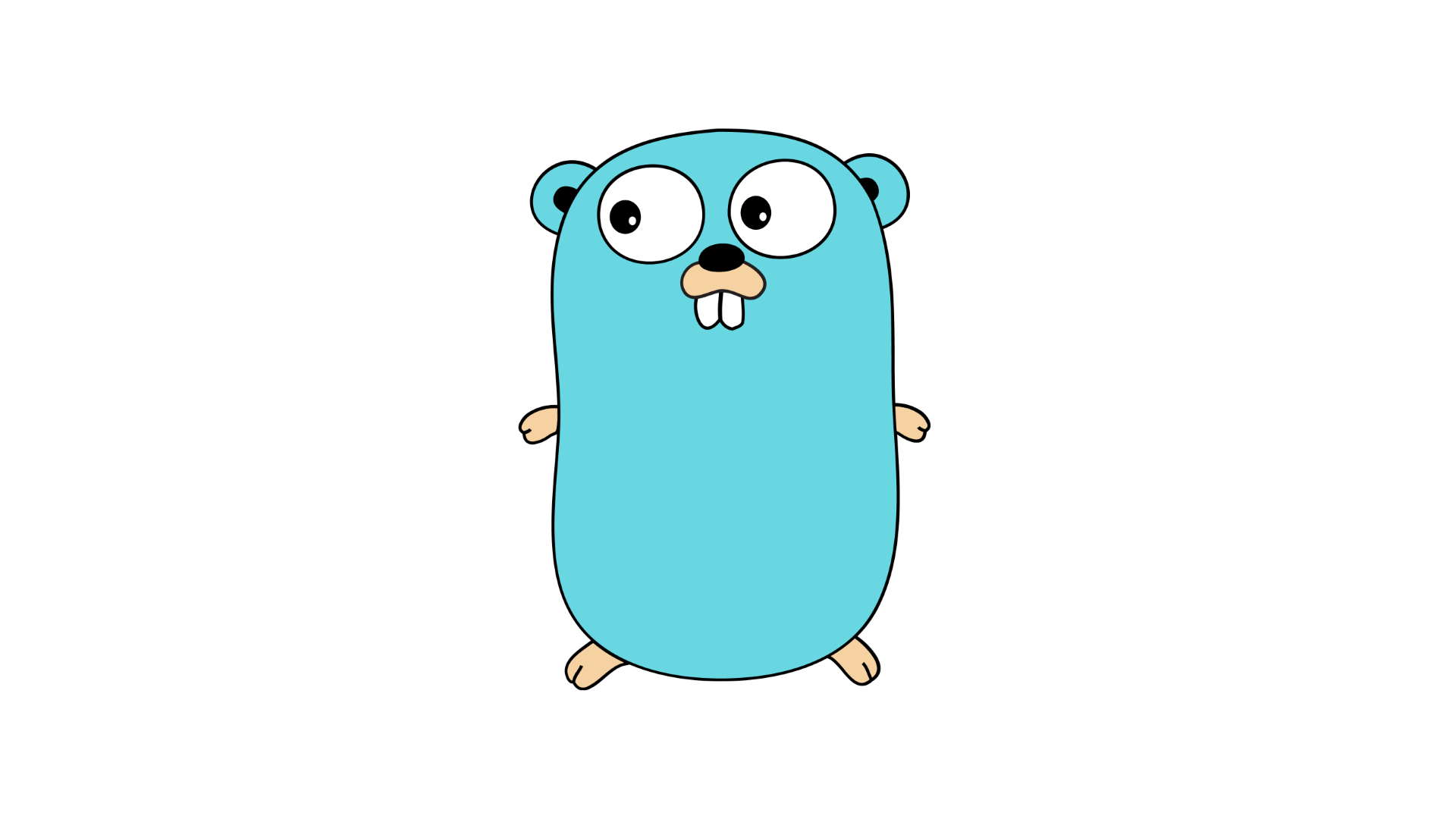
Introduction
Golang is known for its efficient memory management, and it provides various techniques to minimize heap allocation. Heap allocation can be a performance bottleneck, as it involves dynamic memory allocation and garbage collection. In this blog post, we will explore zero allocation techniques in Golang that can help you optimize your code and minimize heap allocation.
What is Heap Allocation?
Heap allocation is a process in which memory is dynamically allocated from the heap at runtime. When you create variables or objects using the new
keyword or when you use composite literals, heap memory is allocated. This memory is managed by the garbage collector and can result in overhead due to the allocation and deallocation process.
Stack vs Heap Memory
In Golang, memory can be allocated on the stack or the heap. Stack memory is faster to allocate and deallocate, as it simply involves moving the stack pointer. Heap memory, on the other hand, requires more time for allocation and deallocation, as it involves interacting with the garbage collector.
Stack memory is used for small, scoped variables with a known lifetime, while heap memory is used for larger objects or variables with a longer lifetime. Minimizing heap allocation can lead to significant performance improvements, as it reduces the overhead of garbage collection and memory management.
Zero Allocation Techniques
1. Use sync.Pool for Object Pooling
The sync.Pool
package provides a way to reuse objects without incurring the overhead of heap allocation. It maintains a pool of temporary objects that can be reused instead of allocating new objects from the heap. This can be particularly useful in scenarios that involve frequent object creation and deletion.
Here's an example of how to use the sync.Pool
package:
import (
"sync"
)
type MyObject struct {
// ...
}
var objectPool = sync.Pool{
New: func() interface{} {
return &MyObject{}
},
}
func getObject() *amp;MyObject {
return objectPool.Get().(*MyObject)
}
func releaseObject(obj *amp;MyObject) {
objectPool.Put(obj)
}
In the above code, we create a sync.Pool with a New function that returns a new MyObject. The getObject function retrieves an object from the pool, and the releaseObject function returns the object back to the pool. By reusing objects, we can minimize heap allocation and improve performance.
2. Use Pool for Byte Slice Reuse
Byte slices are commonly used to store variable-length data. However, creating new byte slices for each operation can lead to unnecessary heap allocations. By reusing byte slices, you can minimize heap allocation and improve performance.
Golang provides the sync.Pool
package, which can be used to pool byte slices. Here's an example:
import (
"sync"
)
var byteSlicePool = sync.Pool{
New: func() interface{} {
return make([]byte, 0, 4096)
},
}
func getByteSlice() []byte {
return byteSlicePool.Get().([]byte)
}
func releaseByteSlice(slice []byte) {
byteSlicePool.Put(slice[:0])
}
In the above code, we create a sync.Pool with a New function that returns a new byte slice. The getByteSlice function retrieves a byte slice from the pool, and the releaseByteSlice function returns the byte slice back to the pool by resetting its length to zero. By reusing byte slices, we can minimize heap allocations and improve performance.
3. Use Buffer Pooling for I/O
Performing I/O operations in Golang involves reading or writing data to a buffer. Allocating a new buffer for each I/O operation can result in unnecessary heap allocations. By reusing buffers, you can minimize heap allocation and improve performance.
The bufio
package provides a way to pool buffers for I/O operations. Here's an example:
import (
"bufio"
"sync"
)
var bufferPool = sync.Pool{
New: func() interface{} {
return make([]byte, 4096)
},
}
func readData(reader *bufio.Reader) ([]byte, error) {
buffer := bufferPool.Get().([]byte)
defer bufferPool.Put(buffer)
return reader.Read(buffer)
}
In the above code, we create a sync.Pool with a New function that returns a new buffer. The readData function retrieves a buffer from the pool, reads data into the buffer using the bufio.Reader, and returns the buffer to the pool. By reusing buffers, we can minimize heap allocations and improve performance.
Conclusion
Minimizing heap allocation is crucial for optimizing the performance of your Golang applications. By using zero allocation techniques such as sync.Pool for object pooling, pool for byte slice reuse, and buffer pooling for I/O operations, you can reduce the overhead of heap allocation and improve the overall performance of your code.
Remember, heap allocation should be avoided when possible, especially in performance-critical scenarios. By carefully managing memory allocation and reusing objects, you can build more efficient and high-performing Golang applications.
That's it for this blog post! I hope you found it informative and that it helps you in minimizing heap allocation in your Golang projects. Happy coding!