Mastering Zero Allocation in Golang: Techniques and Strategies
Learn how to master zero allocation in Go programming, by minimizing unnecessary memory allocations and optimizing your code for maximum performance. Avoid common pitfalls, use pooling techniques, reduce garbage collection pressure, and follow best practices!
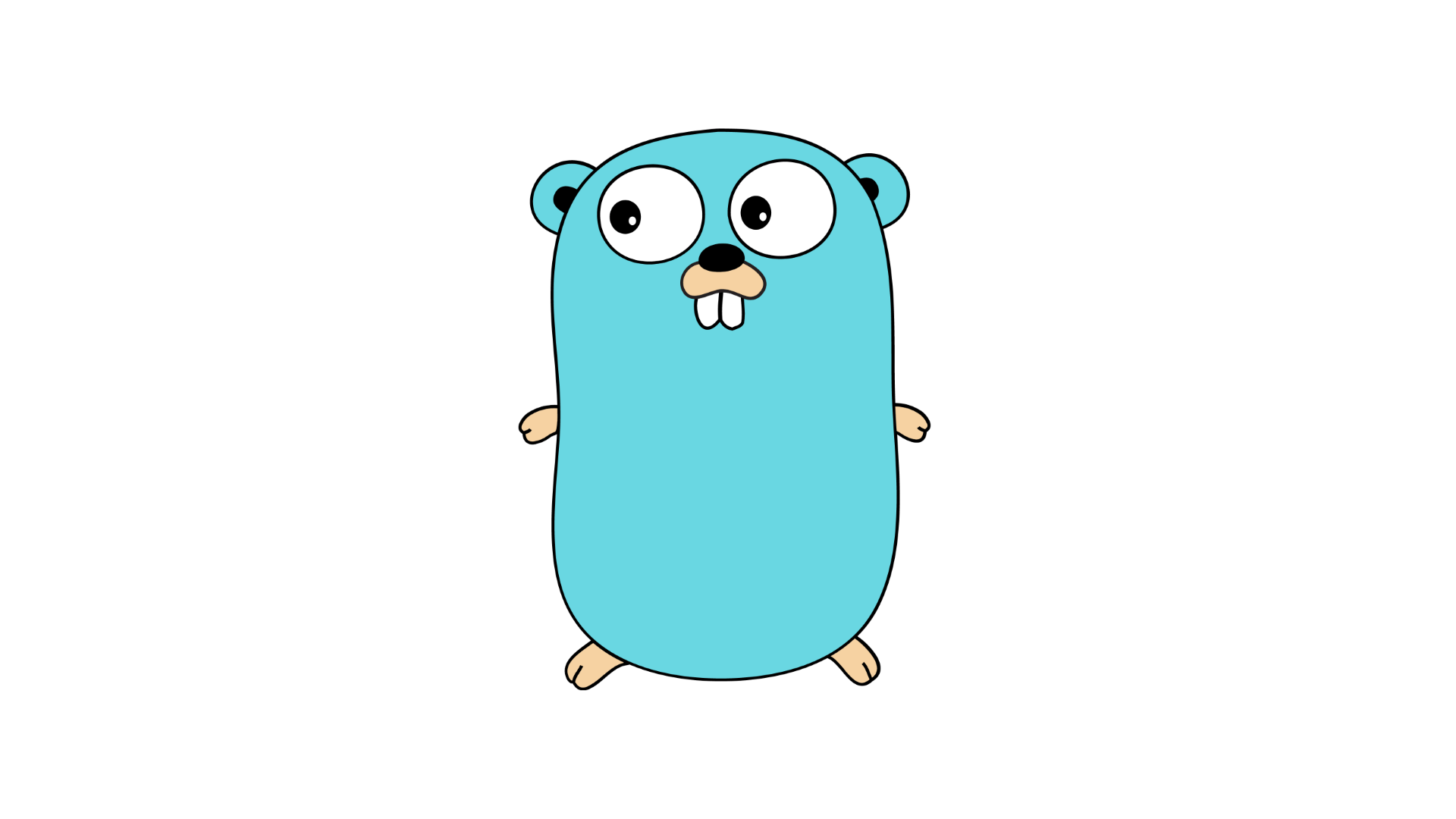
Introduction
Zero allocation is a highly desirable goal in Go programming. By minimizing unnecessary memory allocations, you can improve the performance and efficiency of your applications. In this blog post, we'll explore techniques and strategies to master zero allocation in Golang. We'll cover common pitfalls, memory management techniques, and best practices to help you optimize your Go code and achieve zero allocation. Let's dive in!
What is Zero Allocation?
In Go, memory allocation occurs when you create new objects, such as variables, slices, or maps. This allocation process involves reserving memory on the heap, which requires CPU cycles for allocation and deallocation. However, zero allocation refers to scenarios where no new memory is allocated during the execution of a function or block of code.
Common Pitfalls to Avoid
Before we delve into zero allocation strategies, let's first explore common pitfalls that can lead to unnecessary memory allocations in Go:
1. Creating Unnecessary Variables
func hello() {
name := "John"
fmt.Println("Hello, " + name)
}
In the above code snippet, the variable name
is unnecessary because it's only used once to concatenate with the string literal. To avoid unnecessary allocations, you can directly concatenate the string literal:
func hello() {
fmt.Println("Hello, John")
}
2. Appending Slices
func appendSlice() {
s1 := []int{1, 2, 3}
s2 := []int{4, 5, 6}
s3 := append(s1, s2...)
fmt.Println(s3)
}
Appending slices can result in memory allocations because the append
function creates a new slice. If you know the size of the final slice, you can allocate the right capacity upfront to avoid unnecessary allocations:
func appendSlice() {
s1 := []int{1, 2, 3}
s2 := []int{4, 5, 6}
s3 := make([]int, 0, len(s1)+len(s2))
s3 = append(s3, s1...)
s3 = append(s3, s2...)
fmt.Println(s3)
}
3. Passing Large Structs by Value
type Point struct {
X int
Y int
}
func calculateDistance(p1, p2 Point) {
// ...
}
In the above code, the calculateDistance
function takes two large structs, p1
and p2
, as parameters. This results in a memory allocation as the structs are passed by value. To avoid unnecessary allocations, you can pass the structs by reference (using pointers) instead:
func calculateDistance(p1, p2 *Point) {
// ...
}
Strategies for Zero Allocation
1. Pooling
Pooling involves reusing memory instead of allocating new memory for each use. The sync.Pool
package provides a pool of objects that can be reused across multiple goroutines. By using pooling, you can minimize memory allocations and improve performance.
type Object struct {
// ...
}
var objectPool = sync.Pool{
New: func() interface{} {
return &Object{}
},
}
func getObject() *Object {
return objectPool.Get().(*Object)
}
func releaseObject(obj *Object) {
objectPool.Put(obj)
}
In the above code, the GetObject
function returns an object from the pool, and the ReleaseObject
function returns the object back to the pool for reuse.
2. Reducing Garbage Collection Pressure
Golang's garbage collector (GC) is responsible for freeing up memory that is no longer needed. By reducing the pressure on the garbage collector, you can minimize the number of garbage collection cycles and improve performance. Here are a few techniques to reduce garbage collection pressure:
a. Use Pointer-Based Data Structures
Using pointer-based data structures, such as pointers to structs, slices, or maps, can reduce memory allocations by allowing objects to be reused or modified in-place.
b. Use Sync Pools and Byte Pools
Sync pools and byte pools, like the sync.Pool
and sync.Pool
packages, can help reduce memory allocations and pressure on the garbage collector.
c. Avoid Unnecessary Value Copies
Copying large values unnecessarily, such as passing structs by value or returning large values from functions, can contribute to garbage collection pressure. Consider passing by reference (using pointers) or using slices to avoid unnecessary value copies.
3. Avoiding Heap Allocations
Heap allocations are more expensive than stack allocations in Go. By avoiding unnecessary heap allocations, you can improve performance and reduce memory pressure. Here are a few strategies to avoid heap allocations:
a. Use Value Receivers Instead of Pointer Receivers
If a method doesn't need to modify the receiver object, use value receivers instead of pointer receivers. This avoids the allocation of a pointer and reduces the pressure on the garbage collector.
b. Use Sync Pools for Small Objects
For objects with small sizes, it's often more efficient to use sync pools, like the sync.Pool
package, to reuse objects instead of allocating new ones.
c. Use Stack Memory
Stack memory is faster and more efficient than heap memory. By utilizing stack memory, you can allocate short-lived objects on the stack instead of the heap. This can be achieved by using arrays or slices with fixed sizes instead of dynamic arrays.
Best Practices for Zero Allocation
1. Profile Your Code
Profiling your code using tools like pprof
can help identify performance bottlenecks and excessive memory allocations. By understanding where memory allocations occur, you can apply zero allocation techniques to optimize your code.
2. Use the Right Data Structures
Choosing the appropriate data structures can significantly impact memory allocation. Use data structures that minimize memory allocations and provide efficient access patterns for your specific use case.
3. Benchmark Your Code
Use Go's benchmarking framework to compare the performance and memory usage of different implementations. Benchmarking can help you identify the most efficient approaches and validate the effectiveness of zero allocation techniques.
4. Regularly Review and Refactor Your Code
As your codebase evolves, regularly review and refactor your code to ensure optimal memory management. Refactoring can help optimize memory usage and identify areas where zero allocation techniques can be applied.
Conclusion
Mastering zero allocation in Golang is an essential skill for writing efficient and high-performing applications. By avoiding common pitfalls, using techniques such as pooling and reducing garbage collection pressure, and following best practices, you can minimize unnecessary memory allocations and optimize your Go code for maximum performance.
As you continue your Golang journey, remember to profile, benchmark, and regularly review and refactor your code to ensure optimal memory management and zero allocation. Happy coding!