Mastering Golang's Error Handling Mechanisms: Best Practices
Learn best practices for error handling in Golang, including the use of the error interface, custom error types, and error propagation. Mastering error handling is crucial for writing robust and reliable Go applications.
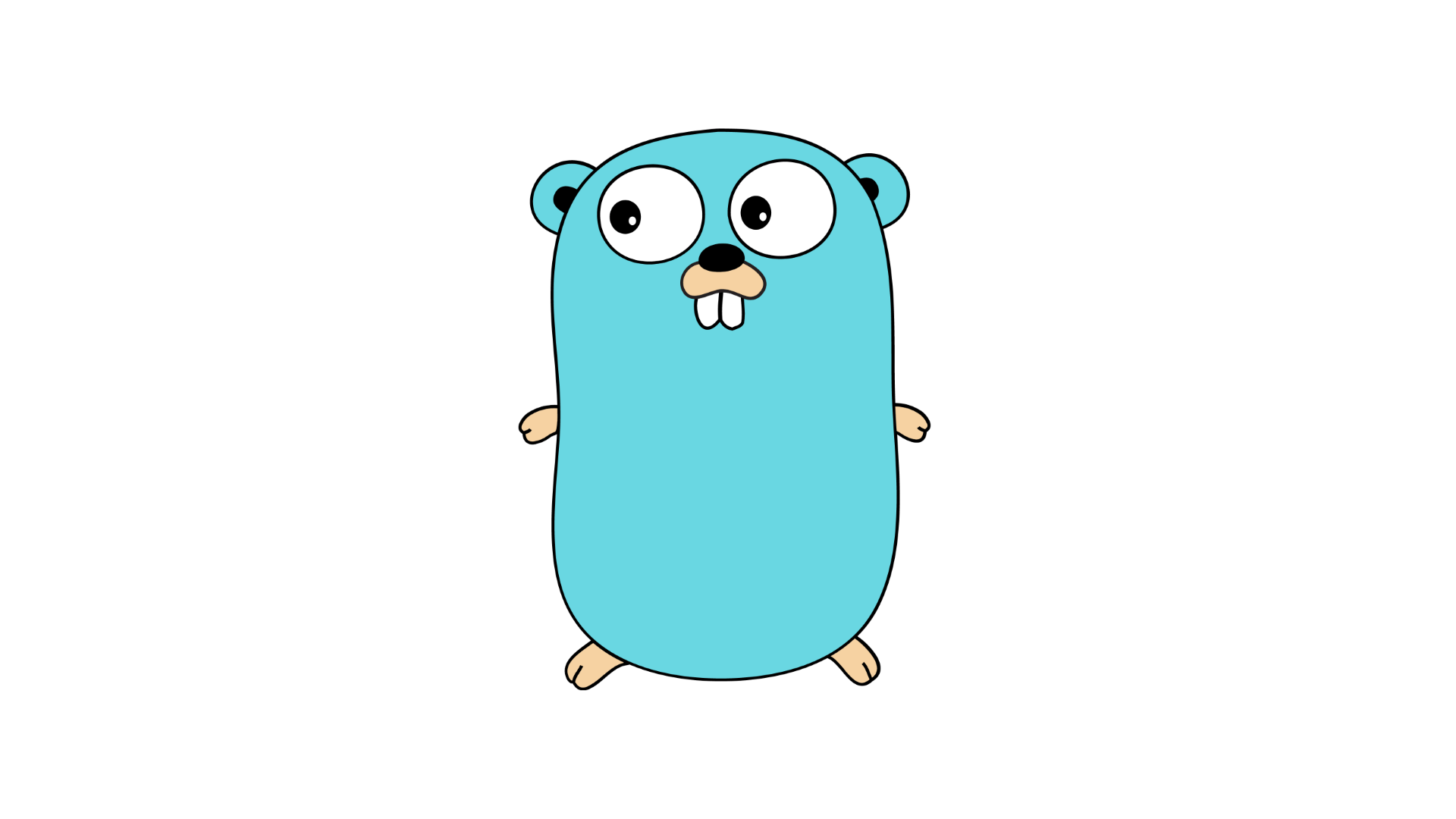
Introduction
Mastering Golang's error handling mechanisms is crucial for writing robust and reliable Go applications. In this comprehensive guide, we will explore best practices for error handling in Go, providing beginners with valuable insights into this important aspect of the language.
Why Error Handling Matters
Error handling plays a vital role in software development. It allows us to identify and handle exceptions, ensuring that our applications gracefully handle unexpected scenarios and recover from errors effectively.
1. Understanding the Error Interface
In Go, error handling revolves around the use of the error
interface. This interface defines the Error()
method, which returns a string value describing the error. By leveraging this interface, we can create custom error types that adhere to Go's error handling conventions.
2. Returning Errors from Functions
Go encourages explicit error handling. It is a common practice to return an error as the last return value from a function call. By convention, if the function fails, it returns a non-nil error value, allowing the caller to handle the error appropriately.
3. Checking for Errors
After calling a function that can potentially return an error, it is essential to check if an error occurred. This can be done using an if
statement to compare the returned error value with nil
. If the error is nil
, the function call was successful. Otherwise, an error occurred, and we need to handle it accordingly.
4. Deferred Functions and Error Handling
Go provides the defer
statement, which allows us to schedule a function call to be executed when the surrounding function finishes executing. This feature can be utilized to handle errors effectively. By using defer
along with recover()
and panic()
, we can catch and handle exceptions at the appropriate level of the call stack.
5. Custom Error Types
While Go provides a built-in error
interface, it is often beneficial to create custom error types that encapsulate additional information about the error. This can allow for more meaningful error messages and better error handling logic.
Best Practices for Error Handling in Go
Now that we have explored the fundamentals of error handling in Go, let's dive into some best practices to follow when implementing error handling in your Go applications:
1. Be Explicit
Always explicitly check for and handle errors. Do not ignore errors or rely on assumptions; this can lead to unexpected behavior and hard-to-debug issues.
2. Don't Repeat Errors
If a function wraps another function call and simply returns the same error, it adds no value. Instead, consider returning the original error directly or add additional information to the error by creating a custom error type.
3. Use Context to Provide Additional Information
When creating custom error types, consider including additional context information that can help with debugging. This can include relevant variable values, function names, or stack traces.
4. Log Errors
Logging errors can be invaluable for troubleshooting and monitoring the health of your application. Consider using a logging framework to record errors along with relevant information for future analysis.
5. Propagate or Wrap Errors
When writing libraries or packages, it is often best to propagate errors up the call stack rather than handle them immediately. This allows the caller to decide on the appropriate error handling strategy.
Conclusion
By mastering Go's error handling mechanisms and following best practices, you will be well-equipped to handle errors effectively in your Go applications. Remember to be explicit, log errors, and propagate or wrap errors when necessary. With these techniques, you will be able to write robust and reliable code that gracefully handles errors and provides a better experience for your users.