Mastering Angular JavaScript: Best Practices and Tips
Learn the best practices and tips for mastering Angular JavaScript development. Set up your environment, structure your projects, and follow coding best practices. Plus, discover valuable tips and tricks to enhance your Angular skills. Get ready to become an Angular master!
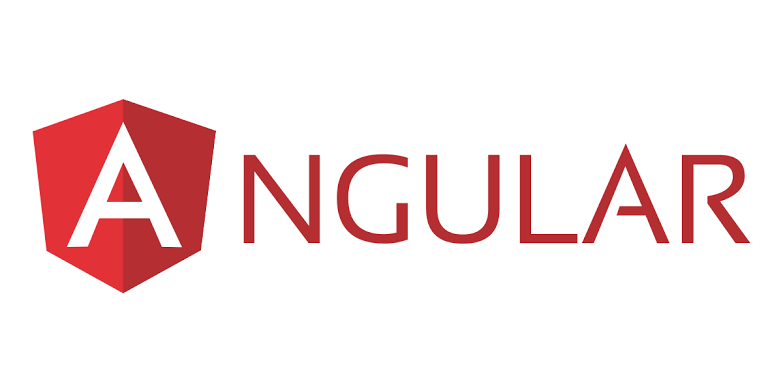
Introduction
Welcome to "Mastering Angular JavaScript: Best Practices and Tips!" In this comprehensive guide, we'll explore the best practices and tips for developing with Angular, a popular JavaScript framework. Whether you're a beginner or an experienced developer, this article will provide valuable insights to help you become a master at Angular development. Let's get started!
Setting Up Your Angular Environment
Before diving into the best practices and tips, let's ensure that you have a properly set up Angular environment. Follow these steps:
- Install Node.js from the official Node.js website. Node.js comes with npm (Node Package Manager), which you'll need to install Angular CLI and manage project dependencies.
- Open your preferred terminal or command prompt and verify that you have successfully installed Node.js by running the following command:
```bash node -v ```
The command should display the version of Node.js you have installed. If no version is displayed or you receive an error, please double-check your installation.
- Next, install Angular CLI by running the following command in your terminal:
```bash npm install -g @angular/cli ```
This command installs Angular CLI globally on your machine, allowing you to create and manage Angular projects from anywhere in the file system. Once the installation is complete, you can verify it by running:
```bash ng version ```
The command should display the Angular CLI version. If the version is displayed, your Angular environment is now set up and ready to go!
Angular Project Structure Best Practices
An organized project structure is crucial for maintaining code maintainability and scalability. Here are some best practices for structuring your Angular projects:
1. Components vs Services
Angular follows a component-based architecture, where components play a crucial role. Components represent UI elements and encapsulate their behavior. On the other hand, services handle data, business logic, and other reusable functionalities.
It's important to keep your components lean and focused on UI-related tasks. Move the business logic and data operations to services, promoting code reusability and separation of concerns.
2. Module Organization
Angular modules help structure your application, bring related components and services together, and provide encapsulation. Here are some recommendations for organizing your modules:
- Create a separate module for each feature or functional area of your application.
- Create a shared module to consolidate commonly used components, directives, and pipes.
- Use the Angular CLI to generate modules, taking advantage of its scaffolding capabilities.
3. Naming Conventions
Consistent naming conventions promote code clarity and ease of maintenance. Consider the following naming conventions:
- Use camel case for file and folder names, e.g.,
myComponent.component.ts
andmyService.service.ts
. - Append the component suffix to components, e.g.,
myComponent.component.ts
. - Use the appropriate suffix for other files such as services, directives, and pipes.
4. Directory Structure
A well-organized directory structure is vital for efficient development. Consider the following suggestions:
- Group related files together. For example, keep components, services, and their corresponding files in the same directory.
- Use barrels (index.ts files) to centralize exports from a directory, allowing for simpler imports and improved maintainability.
- Consider organizing files into subdirectories based on their functionality or feature.
Angular Coding Best Practices
In addition to the project structure, following coding best practices ensures clean and maintainable Angular code. Let's dive into some of these best practices:
1. Use trackBy with ngFor Directive
When using the ngFor directive to render lists, always include a trackBy function to improve performance. This function helps Angular track changes in the list items and only update the necessary DOM elements. Here's an example:
```html
- {{ item.name }}
```
In the component class, define the trackById function:
```typescript trackById(index: number, item: any): number { return item.id; } ```
2. Use Pipe Impure Option Sparingly
By default, Angular pipes are pure, meaning they only execute when their input values change. However, you can make a pipe impure to force it to execute on every change detection cycle. Although this can be useful in specific scenarios, it may result in performance degradation. Use the pure: false
option sparingly and be mindful of the impact on your application's performance.
3. Minimize the Use of ngIf and ngSwitch When Possible
While ngIf and ngSwitch directives provide powerful conditional rendering capabilities, excessive usage can impact performance. Use them judiciously, especially when dealing with large lists or complex templates. Consider alternative approaches such as using CSS or managing conditional rendering in component templates instead.
4. Optimize Change Detection
Angular's change detection mechanism is efficient, but it's essential to be mindful of its impact on performance. Here are some practices to optimize change detection:
- Prefer using
OnPush
change detection strategy for components that don't frequently change. - Avoid using complex expressions or function calls within template bindings as they can trigger unnecessary change detection cycles.
- Use the
ChangeDetectorRef
API to manually trigger change detection when necessary.
Angular Tips and Tricks
Alongside best practices, here are some valuable tips and tricks to enhance your Angular development experience:
1. Use Angular CLI Generators
The Angular CLI provides a set of powerful generators to scaffold components, services, modules, and more. Utilize these generators to save time and reduce boilerplate code. For example, you can create a new component by running:
```bash ng generate component myComponent ```
2. Use Angular Router for Routing
Angular Router makes it easy to implement client-side routing in your application. Leverage Angular Router to create routes, handle navigation, and implement lazy loading of feature modules. Configure your application's routes in the app-routing.module.ts
file to keep your routing logic organized.
3. Check out Observables
Observables are a powerful asynchronous programming feature in Angular. They play a vital role in handling asynchronous data streams and event-based programming. Take the time to understand and utilize Observables to enhance your application's performance and maintainability.
4. Write Unit Tests
Unit tests are essential for maintaining code quality and preventing regressions. Angular has built-in support for unit testing using frameworks like Jasmine and Karma. Write unit tests for your components, services, and other critical parts of your application to ensure they function as intended.
Conclusion
Congratulations on completing this guide to mastering Angular JavaScript! By following the best practices and implementing the tips and tricks discussed here, you'll become a proficient Angular developer. Remember to regularly update your knowledge of Angular, as the framework evolves with new features and enhancements. Happy coding!
Stay tuned for the next part of our guide, where we'll deep dive into advanced Angular concepts, such as reactive forms, dependency injection, and routing. Exciting things are ahead!