Working with Go Modules: Managing Dependencies in Golang Projects
"Go modules make managing dependencies in Go projects easy. Learn how to enable modules, add, update, and remove dependencies, and vendor dependencies efficiently."
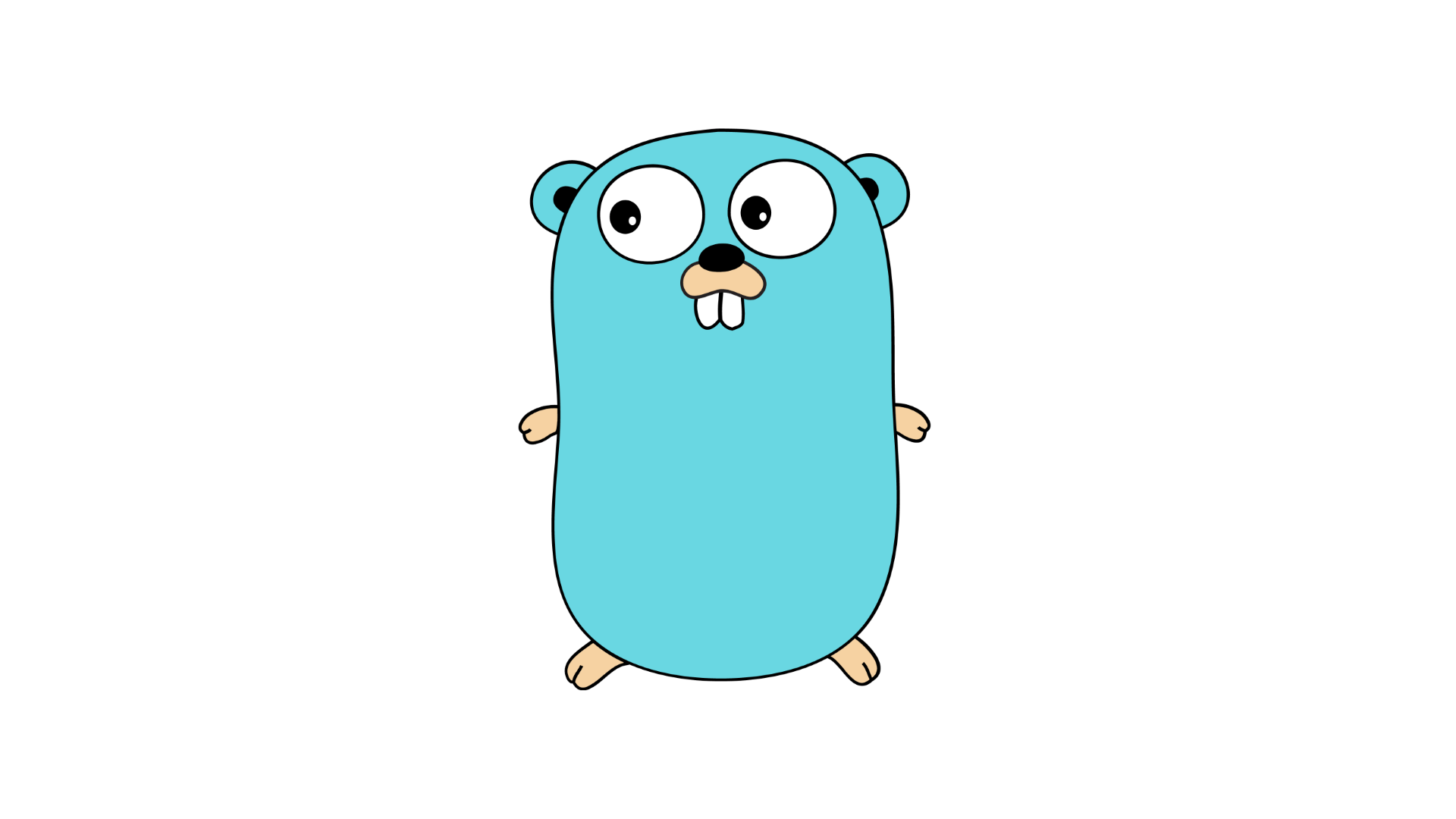
Introduction
Managing dependencies is a critical aspect of software development. As your projects grow in complexity, you'll find it necessary to incorporate external packages and libraries to enhance functionality, improve efficiency, and speed up development. In the Go (Golang) programming language, managing dependencies is made easy with the help of Go modules.
In this tutorial, we'll explore how to work with Go modules and effectively manage dependencies in your Golang projects. Whether you're a beginner or have some experience with Go, this guide will provide you with valuable insights into dependency management in the Go ecosystem.
What are Go Modules?
In Go, a module is a collection of related Go packages that are versioned together as a single unit. Modules provide a way to manage dependencies in your Go projects, ensuring reproducible builds and reliable dependency management. They allow you to define and lock the versions of your dependencies, making it easy to share and collaborate on projects with others.
Starting from Go 1.11, Go modules were introduced into the language as an official dependency management solution, replacing the older GOPATH-based approach. Go modules bring several advantages, including:
- Versioning: You can specify precise versions of your dependencies, making it easier to track changes and ensure compatibility.
- Reproducibility: Go modules provide a reproducible build process, ensuring that your project builds the same way every time, regardless of external changes.
- Module-awareness: Go modules are aware of their context and can work with other module-aware tools, such as package and dependency managers.
- Dependency isolation: Modules are isolated from each other, meaning that changes to one module won't affect others within your project.
Enabling Go Modules
Before starting with Go modules, ensure that you are using Go version 1.11 or higher. Go modules are enabled by default in these versions, so there's no need to set any environment variables.
If you're using an older version of Go, you can enable modules by running the following command:
go mod init
This command initializes a new module in your project and creates a go.mod
file, which serves as the module definition.
The go.mod
file contains information about your module, including its name, version, and the dependencies it requires. It also tracks any changes to your dependencies and their versions.
If you have an existing project that uses a package manager like dep or glide, you can migrate it to Go modules by running:
go mod init
And then:
go mod tidy
The go mod tidy
command analyzes your code and updates the go.mod
file with the correct dependencies and versions based on your import statements.
Adding Dependencies
Adding dependencies to your Go modules is straightforward. All you need to do is import the desired packages in your code, and Go will automatically resolve and download the necessary dependencies.
Let's say you want to add a dependency to your project. You can import it in your code like this:
import "github.com/example/dependency"
After you've imported the package, run the following command to download the dependency:
go mod tidy
The go mod tidy
command reads the import
statements in your code and updates the go.mod
file with the necessary dependencies and their versions.
You can also specify a specific version or commit of a package by appending it to the import statement. For example:
import v1 "github.com/example/dependency/v2"
This will import version 2 of the dependency
package.
Managing Dependencies
Go modules provide several commands to help you manage your dependencies:
go get
: This command downloads and installs packages and their dependencies.go mod download
: This command downloads the required packages, but does not install them.go mod vendor
: This command creates avendor
directory in your project and copies all the dependencies into it. Using this command ensures that your project has a local copy of its dependencies, making it self-contained and reducing the chances of network errors.go mod edit
: This command edits thego.mod
file. It allows you to add, remove, or change the versions of dependencies manually.go mod why
: This command shows why a particular package is necessary. It helps you understand the import graph and why certain dependencies are pulled into your project.
Updating Dependencies
Updating dependencies in Go modules is easy. Simply run the following command:
go get -u
This command updates all the dependencies in your project to their latest versions. If you want to update a specific package, you can specify it in the command:
go get github.com/example/dependency@latest
This will update the dependency
package to the latest version.
Removing Dependencies
If you want to remove a dependency from your project, you can use the go mod tidy
command:
go mod tidy
This command analyzes your codebase, removes unused dependencies, and updates the go.mod
file accordingly.
You can also manually remove a dependency from the go.mod
file and run go mod tidy
to update the dependencies.
Vendoring Dependencies
Go modules also provide support for vendoring dependencies. Vendoring is the practice of including copies of your project's dependencies within your codebase, making it self-contained and independent of external sources.
To create a vendor directory and copy the dependencies into it, run the following command:
go mod vendor
This command creates a vendor
directory in your project and copies all the necessary packages into it. The packages in the vendor
directory will be used instead of the ones in the Go module cache.
To use the vendored dependencies in your project, you need to set the GOFLAGS
environment variable to include the -mod=vendor
flag. For example:
GOFLAGS="-mod=vendor" go run main.go
This ensures that Go uses the vendored dependencies instead of downloading them from the internet.
Cleaning Up
As you work on your project and update dependencies, the Go module cache can become quite large. To clean up the module cache and remove unused dependencies, run the following command:
go mod tidy
This command analyzes your codebase, removes unused dependencies, and updates the go.mod
and go.sum
files accordingly.
Additionally, you can run the following command to remove the entire module cache:
go clean -modcache
This will delete all the downloaded packages and clean up disk space.
Conclusion
Go modules provide a powerful and efficient way to manage dependencies in your Golang projects. By using Go modules, you can easily add, update, and remove dependencies, ensuring reproducible builds and efficient dependency management.
In this tutorial, we covered the basics of working with Go modules, including enabling modules, adding dependencies, managing dependencies, updating dependencies, and vendoring dependencies. With this knowledge, you'll be able to effectively manage dependencies in your Golang projects.
Remember to keep your go.mod
and go.sum
files versioned in your source control to ensure reproducibility and easier collaboration with other developers.
Happy coding with Go modules!