Go by Example: Variadic Functions
In this tutorial, we explore variadic functions in Go, which allow you to work with a variable number of arguments. Learn how to define and call variadic functions, pass slices as arguments, and use them alongside other parameters. A powerful feature for flexible function design.
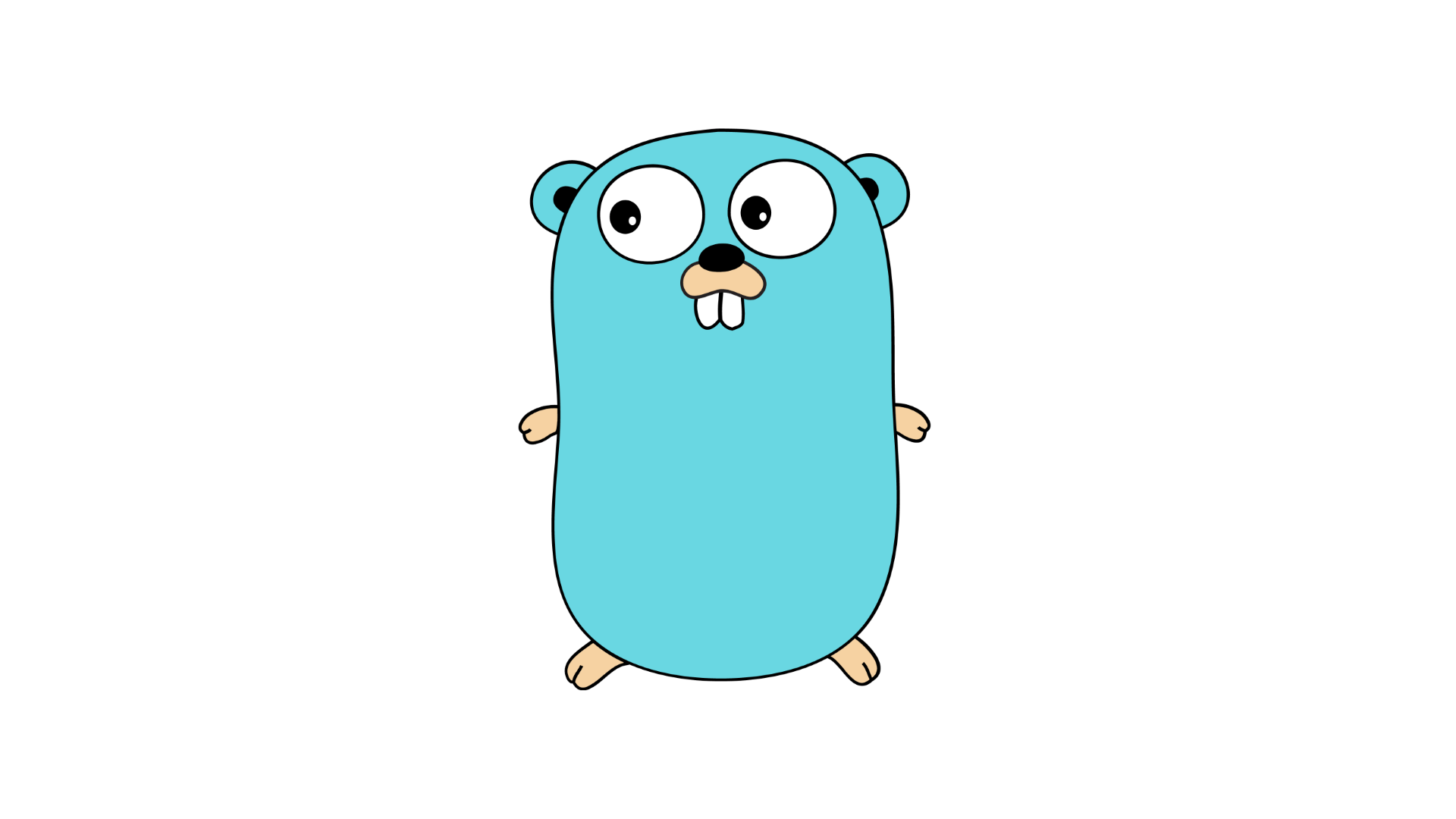
Introduction
Welcome to another installment of our "Go by Example" series! In this tutorial, we will explore a powerful feature of Go called Variadic Functions. Variadic functions allow you to pass an arbitrary number of arguments to a function. This can be incredibly useful in situations where you want to work with a variable number of inputs. By the end of this tutorial, you will have a solid understanding of how to use variadic functions in Go. Let's get started!
What are Variadic Functions?
A variadic function in Go is a function that can accept a variable number of arguments of a specific type. This means you can pass a different number of arguments each time you call the function. The key to defining a variadic function in Go is to use the ellipsis notation (...
) before the type of the parameter that will accept the variable arguments. Let's take a look at an example:
func sum(nums ...int) int {
total := 0
for _, num := range nums {
total += num
}
return total
}
In the above example, we define a function called sum
that accepts a series of integers as its arguments. By using the ellipsis notation (...
) in the function signature, we allow for a variable number of arguments of type int
. Inside the function, we loop through all the arguments and calculate their sum. Finally, we return the total sum.
Calling Variadic Functions
Calling variadic functions is straightforward. You can pass any number of arguments separated by commas. Let's see how we can call our sum
function with multiple arguments:
sum(1, 2, 3)
When we call the sum
function with multiple arguments, Go automatically packs those arguments into a slice of the specified type. In this case, Go will create a slice of type []int
with the arguments 1
, 2
, and 3
.
It is also possible to pass an existing slice to a variadic function. In such cases, you need to use the ellipsis notation when passing the slice argument. Here's an example:
// Create a slice of integers
nums := []int{4, 5, 6}
// Call the sum function with the slice
sum(nums...)
In the above example, we create a slice of integers called nums
with the values 4
, 5
, and 6
. When we call the sum
function, we use the ellipsis notation (nums...
) to pass the slice of integers as variadic arguments.
Variadic Functions with Other Parameters
Variadic functions in Go can have other parameters in addition to the variadic parameter. However, the variadic parameter should be the last one in the function signature. Let's see an example:
func greet(message string, names ...string) {
for _, name := range names {
fmt.Printf("%s, %s!\n", message, name)
}
}
// Call the greet function
greet("Hello", "Alice", "Bob", "Charlie")
In this example, we have defined a function called greet
that takes a message
string parameter followed by as many names
as we want. Inside the function, we iterate over the names
using a for
loop and print a greeting message for each name.
When calling the greet
function, we provide the message
as the first argument followed by the names
"Alice", "Bob", and "Charlie". Go packs these names into a slice of type []string
and passes it to the greet
function.
Conclusion
Variadic functions are a powerful concept in Go that allow you to work with a variable number of arguments. By using the ellipsis notation, you can define variadic parameters and pass any number of arguments to your functions. Whether you need to sum a series of integers or greet a list of names, variadic functions provide an elegant solution. We hope this tutorial has helped you understand variadic functions in Go. Happy coding!
Stay tuned for the next installment of our "Go by Example" series, where we will continue exploring important Go concepts and features.