Go by Example: Slices
Learn how to work with slices in Go, a flexible and dynamic way to store and manipulate arrays. Discover different methods to create and modify slices, and explore various operations you can perform on them. Enhance your Go programming skills with this comprehensive tutorial.
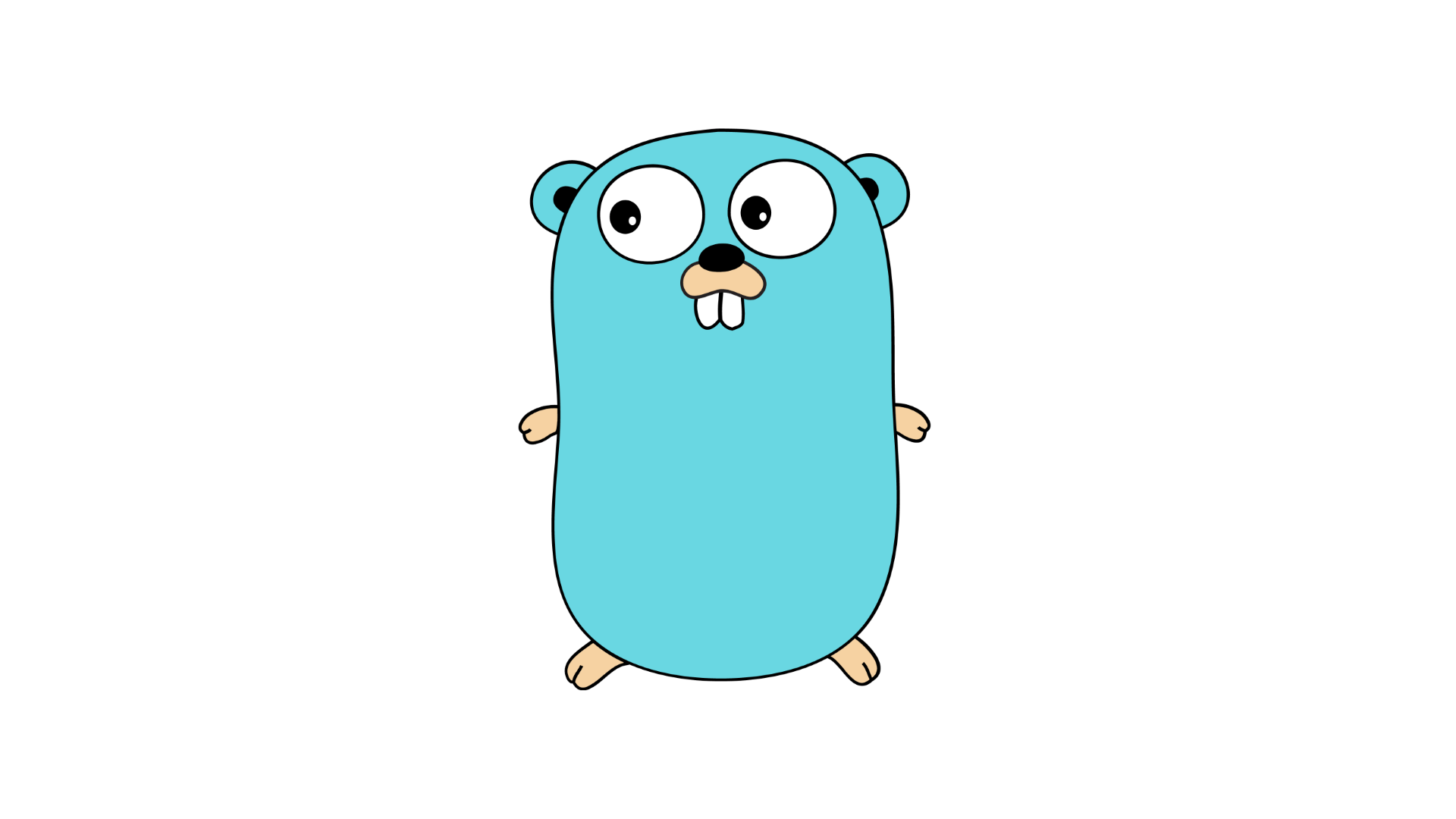
Introduction
Welcome to another installment of our Go by Example series! In this tutorial, we'll be diving into the concept of slices in Go. Slices are essential for working with collections of data, providing a flexible way to store and manipulate dynamically sized arrays. Understanding how to use slices effectively will greatly enhance your Go programming skills. So, let's get started!
What are Slices?
In Go, a slice is a dynamically sized and contiguous segment of an array. Slices are similar to arrays, but unlike arrays, their size can change dynamically. Slices are commonly used to represent and manipulate collections of data.
One important thing to note is that slices do not store any data themselves. Instead, they provide a view or window into an underlying array. This means that when you modify a slice, you are actually modifying the underlying array.
Creating Slices
There are several ways to create slices in Go. Let's explore each method:
Method 1: Using an Array as a Source
numbers := [5]int{1, 2, 3, 4, 5}
slice := numbers[1:4]
fmt.Println(slice) // Output: [2 3 4]
In this example, we create an array called numbers
with 5 elements. We then create a slice called slice
using the syntax numbers[startIndex:endIndex]
. The resulting slice includes elements at indices 1, 2, and 3 of the numbers
array.
Method 2: Using the make() Function
slice := make([]int, 3)
fmt.Println(slice) // Output: [0 0 0]
In this example, we use the make()
function to create a slice called slice
of type []int
with a length of 3. The make()
function initializes the slice's underlying array with zero values.
Method 3: Using a Literal Syntax
slice := []int{1, 2, 3}
fmt.Println(slice) // Output: [1 2 3]
Here, we use the literal syntax to create a slice called slice
with the elements 1, 2, and 3. The length of the slice is determined by the number of elements specified in the literal.
Modifying Slices
One of the key advantages of using slices is their dynamic nature. Let's explore how we can modify slices in Go:
Adding Elements to a Slice
slice := []int{1, 2, 3}
slice = append(slice, 4)
fmt.Println(slice) // Output: [1 2 3 4]
In this example, we use the append()
function to add an element (4) to the end of the slice. The append()
function takes care of resizing the underlying array if necessary.
Removing Elements from a Slice
slice := []int{1, 2, 3, 4, 5}
slice = append(slice[:2], slice[3:]...)
fmt.Println(slice) // Output: [1 2 4 5]
Here, we remove an element from the slice by using the slicing syntax to exclude the element we want to remove. In this case, we remove the element at index 2 (value 3) from the slice.
Operations on Slices
Slicing a Slice
numbers := []int{1, 2, 3, 4, 5}
slice := numbers[1:4]
fmt.Println(slice) // Output: [2 3 4]
Similar to arrays, we can also create a new slice from an existing slice by using the slicing syntax.
Copying a Slice
slice1 := []int{1, 2, 3}
slice2 := make([]int, len(slice1))
copy(slice2, slice1)
fmt.Println(slice2) // Output: [1 2 3]
If you need to create a new slice with the same elements as an existing slice, you can use the copy()
function. This ensures that the new slice has its own independent underlying array.
Conclusion
Congratulations on making it through this tutorial on slices in Go! Slices are a powerful tool for working with dynamic collections of data. By understanding how to create and manipulate slices, you'll have the ability to build more flexible and efficient Go programs.
Stay tuned for our next tutorial in the Go by Example series, where we'll explore another important concept in Go programming. Happy coding!