Introduction to Golang: A Beginner's Guide
In this beginner's guide, we will explore the basics of Golang and why it is a great language for beginners to learn. Discover the simplicity and efficiency of Golang, and get started with writing your first Golang program.
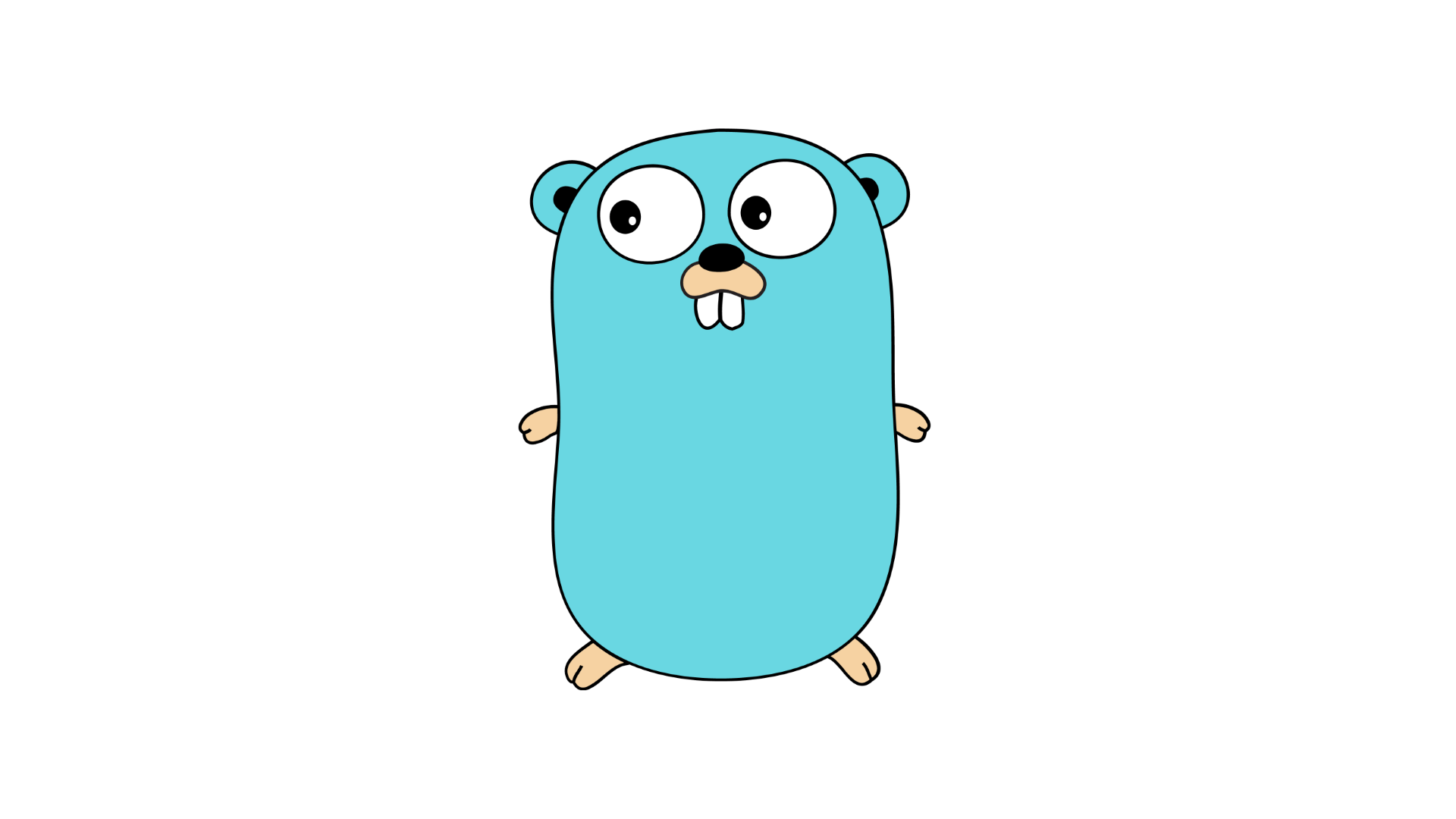
Golang, also known as Go, is a programming language developed by Google. It was designed to be simple, efficient, and easy to use. In this beginner's guide, we will explore the basics of Golang and why it is a great language for beginners to learn.
Why should beginners learn Golang?
Golang offers several advantages that make it an ideal choice for beginners. Firstly, its syntax is clean and easy to understand, making it less intimidating for those who are new to programming. Let's take a look at an example to see how Golang's syntax compares to other languages:
// Golang
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
# Python
print("Hello, World!")
As you can see, Golang's syntax is straightforward and easy to read. This simplicity makes it easier for beginners to grasp the concepts and start writing code.
Additionally, Golang has a strong focus on simplicity and readability, which means that beginners can quickly grasp the concepts and start writing code. Golang's standard library provides a wide range of packages and functions that make it easy to accomplish common tasks without having to rely on external libraries.
How to get started with Golang
To get started with Golang, you will need to install the Go compiler and set up your development environment. The official Golang website provides detailed instructions on how to do this for different operating systems. Once you have everything set up, you can start writing your first Golang program.
Understanding the Basics of Golang
Before diving into writing code, it's important to understand the basic syntax and structure of Golang.
Syntax and Structure of Golang
Golang uses a C-like syntax, which means that it uses curly braces to define blocks of code. Here's an example of a simple Golang program:
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
In this example, we have a main
function that is the entry point of our program. The fmt.Println
function is used to print the string "Hello, World!" to the console.
Variables and Data Types in Golang
In Golang, variables are declared using the var
keyword, followed by the variable name and its data type. Golang supports various data types, including integers, floats, strings, booleans, and more. Here's an example of declaring and using variables in Golang:
package main
import "fmt"
func main() {
var name string = "John"
var age int = 25
var isStudent bool = true
fmt.Println("Name:", name)
fmt.Println("Age:", age)
fmt.Println("Is Student:", isStudent)
}
In this example, we declare three variables: name
of type string
, age
of type int
, and isStudent
of type bool
. We then use the fmt.Println
function to print the values of these variables to the console.
Control Flow and Loops in Golang
Control flow refers to the order in which statements are executed in a program. Golang provides several control flow statements, such as if-else
statements and switch
statements, which allow you to control the flow of your program based on certain conditions. Here's an example of using an if-else
statement in Golang:
package main
import "fmt"
func main() {
age := 18
if age >= 18 {
fmt.Println("You are an adult")
} else {
fmt.Println("You are a minor")
}
}
In this example, we use an if-else
statement to check if the age
variable is greater than or equal to 18. If it is, we print "You are an adult", otherwise we print "You are a minor".
Golang also provides loops, such as for
loops and while
loops, which allow you to repeat a block of code multiple times. Here's an example of using a for
loop in Golang:
package main
import "fmt"
func main() {
for i := 1; i <= 5; i++ {
fmt.Println(i)
}
}
In this example, we use a for
loop to print the numbers from 1 to 5 to the console.
Exploring Golang's Key Features
Golang offers several key features that set it apart from other programming languages. Let's take a look at some of these features.
Concurrency and Goroutines
Concurrency is the ability to execute multiple tasks simultaneously. Golang makes it easy to write concurrent programs using goroutines. A goroutine is a lightweight thread of execution that can run concurrently with other goroutines. Here's an example of using goroutines in Golang:
package main
import (
"fmt"
"time"
)
func printNumbers() {
for i := 1; i <= 5; i++ {
fmt.Println(i)
time.Sleep(1 * time.Second)
}
}
func printLetters() {
for i := 'a'; i <= 'e'; i++ {
fmt.Println(string(i))
time.Sleep(1 * time.Second)
}
}
func main() {
go printNumbers()
go printLetters()
time.Sleep(6 * time.Second)
}
In this example, we define two functions printNumbers
and printLetters
that print numbers and letters respectively. We use the go
keyword to start these functions as goroutines. The time.Sleep
function is used to pause the execution of the main goroutine for a certain duration to allow the other goroutines to execute.
Error Handling in Golang
Error handling is an important aspect of any programming language, and Golang provides a simple and effective way to handle errors. Golang uses the built-in error
type to represent errors. Functions that can return an error typically have a return type of (result, error)
. Here's an example of error handling in Golang:
package main
import (
"fmt"
"os"
)
func main() {
file, err := os.Open("myfile.txt")
if err != nil {
fmt.Println("Error:", err)
return
}
defer file.Close()
// Code to read and process the file goes here
}
In this example, we use the os.Open
function to open a file. If an error occurs, the function returns an error value, which we check using the if err != nil
statement. If an error occurs, we print the error message and return from the function.
Packages and Imports in Golang
Golang uses packages to organize code into reusable units. A package is a collection of Go source files that are compiled together. Golang provides a standard library with a wide range of packages that you can use in your programs. To use a package, you need to import it using the import
keyword. Here's an example of importing and using a package in Golang:
package main
import (
"fmt"
"math/rand"
)
func main() {
fmt.Println("Random number:", rand.Intn(100))
}
In this example, we import the fmt
package, which provides functions for formatted I/O, and the math/rand
package, which provides functions for generating random numbers. We then use the fmt.Println
function to print a random number to the console.
Building Your First Golang Program
Now that you have a good understanding of the basics of Golang, it's time to build your first Golang program. Setting up the development environment is the first step, and the official Golang website provides detailed instructions for different operating systems. Once you have everything set up, you can start writing your first Golang program.
Resources for Learning Golang
There are plenty of resources available online to help you learn Golang. Online tutorials and documentation provided by the official Golang website are a great place to start. Additionally, the Golang community is very active, and there are several forums and discussion boards where you can ask questions and get help. If you prefer learning from books, there are also some recommended books for Golang beginners.
Conclusion
In conclusion, Golang is a beginner-friendly programming language that offers simplicity, efficiency, and powerful features. By understanding the basics of Golang and exploring its key features, beginners can quickly start building their own Golang programs. So, don't hesitate to dive into Golang and start your programming journey today!
Introduction to Golang: A Beginner's Guide - FAQ
Toggle the headers to reveal the answers to the most frequently asked questions about Golang and this beginner's guide.
Q: What is Golang?
A: Golang, also known as Go, is a programming language developed by Google. It is designed to be simple, efficient, and easy to use.
Q: Why should beginners learn Golang?
A: Beginners should learn Golang because it offers a clean and easy-to-understand syntax, making it less intimidating for those new to programming. Golang also has a strong focus on simplicity and readability, allowing beginners to quickly grasp the concepts and start writing code.
Q: How do I get started with Golang?
A: To get started with Golang, you need to install the Go compiler and set up your development environment. The official Golang website provides detailed instructions for different operating systems.
Q: What are the basics of Golang that I should understand?
A: The basics of Golang include understanding its syntax and structure, variables and data types, and control flow and loops. Golang uses a C-like syntax, variables are declared with their respective data types, and control flow statements like if-else and loops are used to control the flow of the program.
Q: What are some key features of Golang?
A: Golang offers several key features, including built-in support for concurrency using goroutines, effective error handling using the error type, and the use of packages and imports to organize and reuse code.
Q: How can I build my first Golang program?
A: To build your first Golang program, you need to set up the development environment, write the code using Golang's syntax and features, and then run the program using the Go compiler.
Q: Are there any resources available for learning Golang?
A: Yes, there are plenty of resources available for learning Golang. Online tutorials and documentation provided by the official Golang website are a great place to start. Additionally, the Golang community is very active, and there are several forums and discussion boards where you can ask questions and get help. Recommended books for Golang beginners are also available.
Q: Why is Golang a beginner-friendly programming language?
A: Golang is considered beginner-friendly because of its clean syntax, focus on simplicity and readability, and the availability of extensive documentation and resources. These factors make it easier for beginners to understand and start writing code in Golang.
Q: Can I use Golang for building real-world applications?
A: Yes, Golang is widely used for building real-world applications. It is known for its efficiency, scalability, and ability to handle concurrent tasks, making it suitable for various applications, including web development, networking, and system programming.
Q: Is Golang a popular programming language?
A: Yes, Golang has gained popularity in recent years due to its simplicity, efficiency, and strong support for concurrency. It is widely adopted by companies like Google, Uber, and Dropbox, and has a growing community of developers.