Go by Example: Constants
Learn about constants in Go programming - immutable values that ensure reliability, readability, and maintainability. Discover how to declare and use various types of constants, and the advantages they bring to your code.
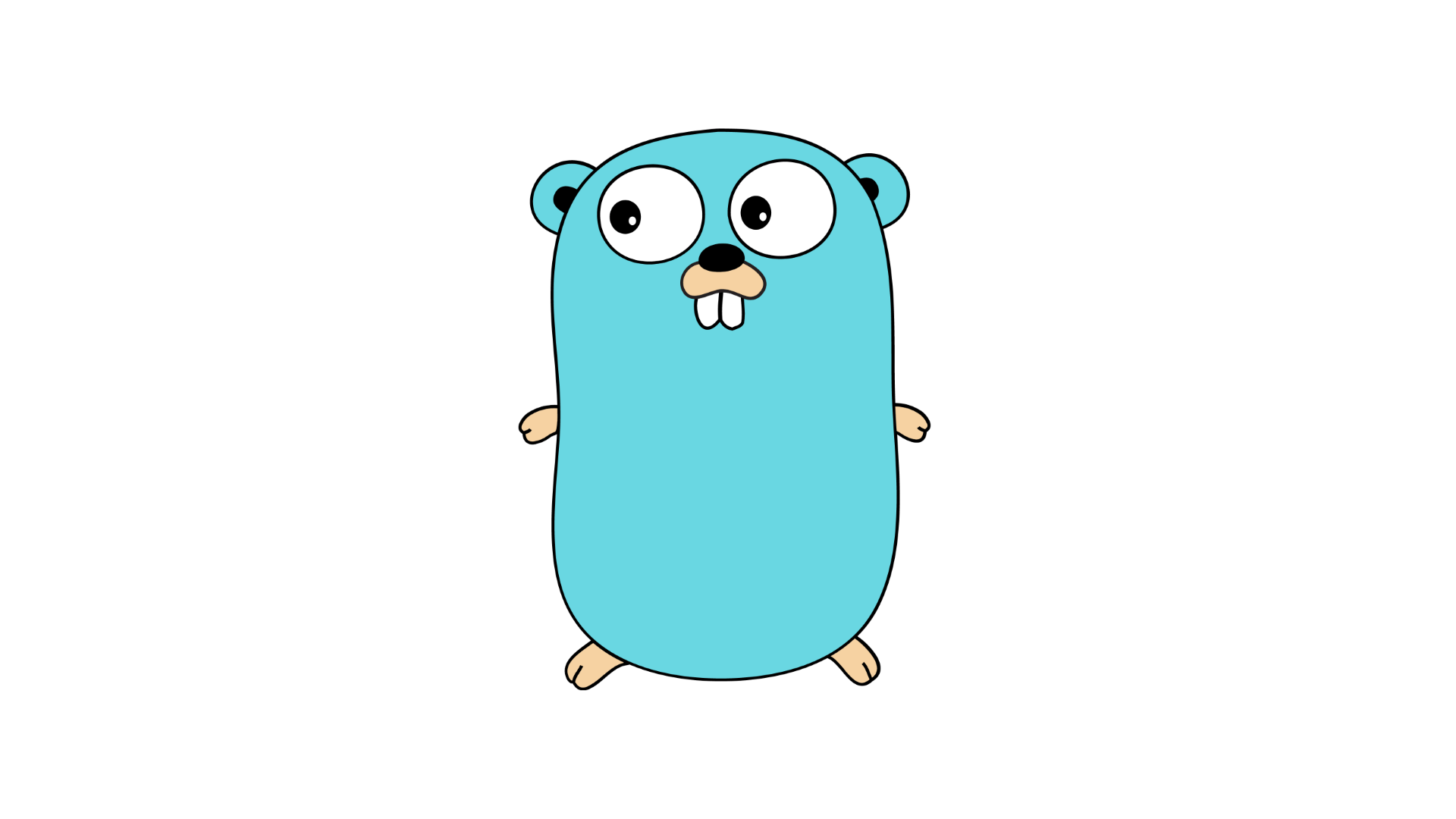
Introduction
Welcome to the world of Go programming! In this tutorial, we'll explore one of the fundamental concepts of Go: Constants. Constants are immutable values that provide a way to store fixed values in your program. They play a significant role in ensuring reliability, readability, and maintainability of your code. By the end of this tutorial, you'll have a solid understanding of constants in Go and how to use them effectively in your programs. Let's get started!
What are Constants?
In Go, a constant is a variable whose value cannot be changed once it is assigned. It is a fixed value that remains the same throughout the execution of a program. Constants are used to store values that are known and do not change, such as mathematical constants (e.g., π) or configuration parameters.
Declaring and Initializing Constants
To declare a constant in Go, you need to use the const
keyword followed by the name of the constant and its value. Here's an example:
const pi = 3.14159
In the above example, we declared a constant named pi
and assigned it the value 3.14159
. Once assigned, the value of pi
cannot be changed throughout the program.
It's important to note that Go supports various types of constants, including numeric constants, string constants, boolean constants, and more. Let's explore each type in more detail.
Numeric Constants
Numeric constants in Go can be integers, floating-point numbers, or complex numbers. You can declare a numeric constant without explicitly specifying its type, and Go will infer it based on the value you assign. Here are some examples:
const daysInWeek = 7 // integer constant
const pi = 3.14159 // floating-point constant
const imaginaryUnit = 0i // complex number constant
In the above examples, daysInWeek
is an integer constant, pi
is a floating-point constant, and imaginaryUnit
is a complex number constant. Once assigned, these values cannot be modified.
String Constants
String constants, also known as string literals, are created by enclosing a sequence of characters in double quotes ("
). Here's an example:
const greeting = "Hello, World!"
The above example declares a constant named greeting
and assigns it the value "Hello, World!"
. Like other constants, the value of greeting
cannot be changed throughout the program.
Boolean Constants
Boolean constants in Go represent the truth values true
and false
. They are declared using the true
or false
keywords. Here's an example:
const isActive = true
const isExpired = false
In the above example, isActive
is a boolean constant with the value true
, and isExpired
is a boolean constant with the value false
. Once assigned, these values cannot be changed.
Typed and Untyped Constants
In Go, constants can be either typed or untyped. An untyped constant is a constant that does not have an explicit type specified. When working with untyped constants, Go allows implicit conversions to compatible types. Here's an example:
const apple = 3 // untyped integer constant
const banana = 2.5 // untyped floating-point constant
var total = apple + banana // implicit conversion to float64
In the above example, apple
is an untyped integer constant, banana
is an untyped floating-point constant, and total
is a variable of type float64
that stores the sum of apple
and banana
. The untyped constants are implicitly converted to float64
for the addition operation.
On the other hand, a typed constant is a constant with an explicit type specified. Typed constants are used when you want to enforce the type of the constant explicitly. Here's an example:
const (
a int = 5
b int = 7
)
var sum = a + b // no implicit conversion, sum is of type int
In the above example, the constants a
and b
are explicitly typed as int
. The variable sum
is also of type int
, and no implicit conversions are performed.
Using Constants in Go
Constants can be used throughout your Go programs, just like variables. They provide a way to store fixed values that should not change. Constants are useful in situations where you have values that remain constant across different parts of your program but need to be easily updated or modified in a single place.
Here's an example that demonstrates the usage of constants:
package main
import "fmt"
func main() {
const daysInWeek = 7
const hoursInDay = 24
fmt.Println("There are", daysInWeek, "days in a week.")
fmt.Println("There are", hoursInDay, "hours in a day.")
}
In the above example, we declared two constants: daysInWeek
and hoursInDay
. We then used these constants to print the number of days and hours in a week and a day, respectively.
Advantages of Using Constants
Constants offer several advantages when used in your Go programs:
- Readability: Constants make the code more readable by providing meaningful names to fixed values.
- Maintainability: Constants allow you to modify a value in a single place, making it easier to update or modify the value if needed.
- Type safety: Typed constants enforce the type explicitly, preventing unexpected type conversions.
- Compile-time errors: Any attempts to modify the value of a constant will result in a compile-time error, ensuring the integrity of your code.
Conclusion
Congratulations! You now have a solid understanding of constants in Go. You learned how to declare and initialize constants, explored various types of constants, and discovered the advantages of using constants in your programs. By leveraging constants effectively, you can enhance the reliability and maintainability of your Go code.
Now that you're familiar with constants, you're ready to dive deeper into Go programming. Stay tuned for the next part of our tutorial series, where we'll explore another important concept: Variables in Go. Happy coding!