Understanding the Key Concepts of Domain-Driven Design in Golang: Aggregates, Entities, and Value Objects
Domain-Driven Design (DDD) is a software development methodology that focuses on creating software solutions based on a deep understanding of the domain it serves, bringing modularity, flexibility, and improved domain understanding to software projects.
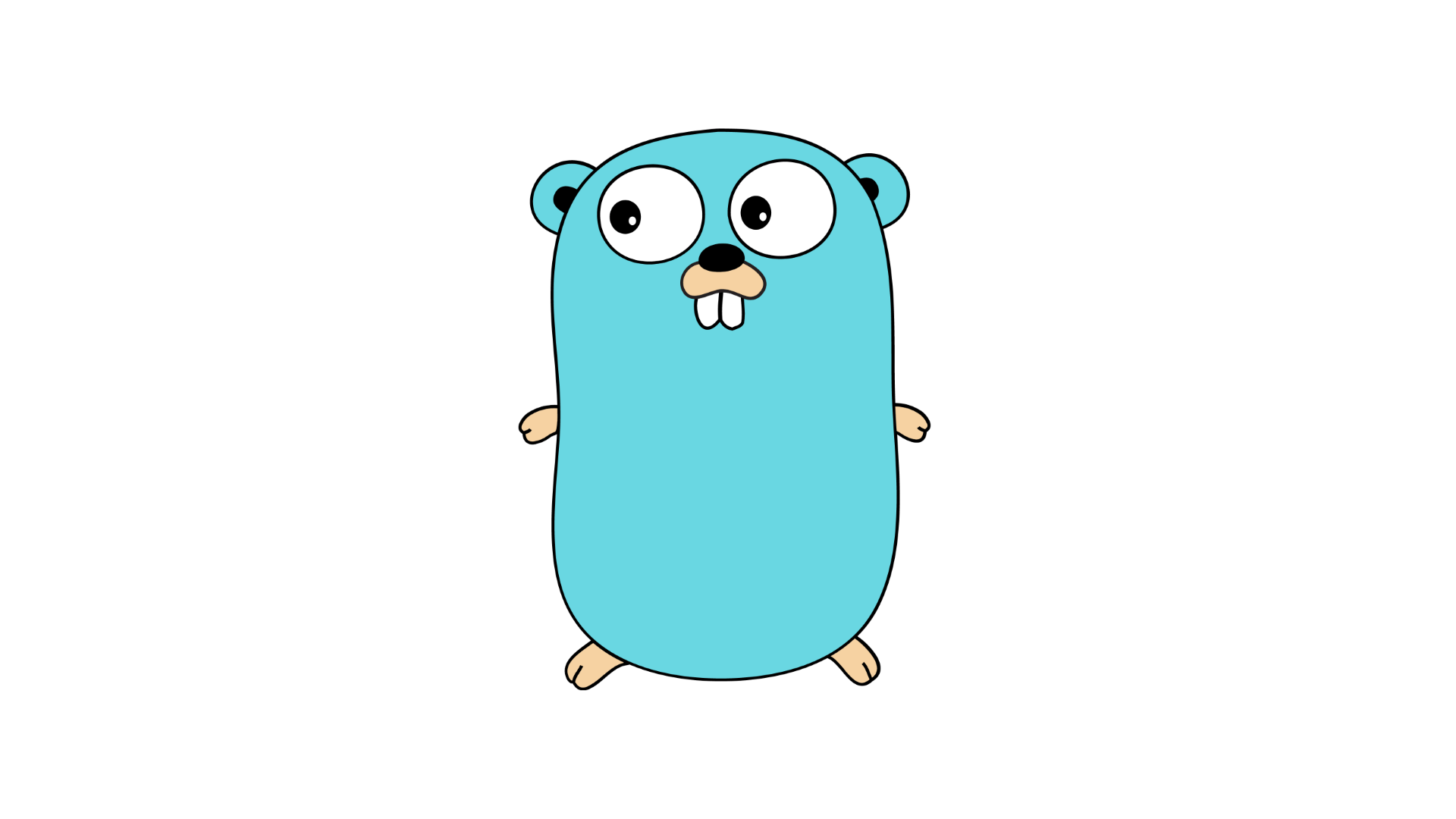
Introduction
Domain-Driven Design (DDD) is a software development methodology that focuses on creating software solutions based on a deep understanding of the domain it serves. It is an approach that emphasizes collaboration between domain experts and software developers to build software systems that are more aligned with the problem space.
In this blog post, we will explore the key concepts of DDD and how they can be applied in the context of Go (Golang) development. Specifically, we will delve into the concepts of aggregates, entities, and value objects and understand how they contribute to building well-designed and maintainable software systems.
What is Domain-Driven Design?
Domain-Driven Design (DDD) is an architectural style that aims to simplify the complexity in software development by placing the domain at the center of the design process. The core principle of DDD is to focus on creating a rich and meaningful model of the problem domain, which allows developers to deliver software that is highly aligned with the real-world domain it serves.
DDD encourages developers to collaborate closely with domain experts to gain a deep understanding of the problem space and to create models that accurately represent domain concepts and business rules. By doing so, DDD helps to tackle the challenges associated with complex domains and enables the development of software systems that evolve successfully over time.
Aggregates
An aggregate is a core building block of DDD. It is a cluster of related domain objects that are treated as a single unit. Aggregates have a clearly defined boundary that encapsulates a set of related entities and value objects, providing consistency and integrity within the aggregate.
In Go, aggregates can be implemented using struct types that represent the aggregate root, which is the entry point to the aggregate. The aggregate root is responsible for coordinating the operations within the aggregate and ensuring its consistency and invariants are maintained.
Let's consider an example of an e-commerce application. In this application, an Order could be an aggregate root that encapsulates a set of related OrderItems and a Customer entity. The OrderItems and Customer would be entities within the aggregate, and they would be directly accessed and modified through the aggregate root.
Entities
An entity is an object with a unique identity that persists over time. Entities represent concepts that are important in the domain and have a lifecycle independent of their attributes. Identifying and modeling entities correctly is essential in DDD to ensure the integrity and consistency of the domain model.
In Go, entities can be implemented as struct types that have a unique identifier. The unique identifier could be a primitive type like an integer, string, or a custom identifier type that enforces uniqueness and encapsulates the logic for generating and comparing identifiers. By encapsulating the identity within the entity, we can ensure that the entity remains consistent and can easily be linked to other entities within the aggregate or across aggregates.
Value Objects
A value object is an object that represents a concept or a piece of information in the domain, but without a unique identity. Value objects are immutable and are typically compared based on their attributes. Unlike entities, value objects do not have a lifecycle of their own and are generally used to represent concepts that are shared by multiple entities.
In Go, value objects can be implemented as struct types with value semantics. Value objects should be immutable, meaning their attributes cannot be modified after creation. By enforcing immutability, we can ensure that value objects are free from any side effects and that they can be safely shared across entities and aggregates.
Benefits of Domain-Driven Design
Domain-Driven Design brings numerous benefits to software development projects, some of which include:
- Improved domain understanding: DDD helps developers gain a deep understanding of the domain by collaborating closely with domain experts, resulting in more accurate and complete domain models.
- Modularity and maintainability: By organizing software around the concepts of aggregates, entities, and value objects, code becomes more modular and easier to understand and maintain.
- Flexibility and adaptability: DDD enables software systems to evolve more gracefully over time by creating a flexible and powerful domain model that can adapt to changing requirements and business needs.
Conclusion
By understanding the key concepts of Domain-Driven Design, such as aggregates, entities, and value objects, you can significantly improve the design and maintainability of your Go applications. DDD provides a powerful approach to software development that brings the domain to the forefront, resulting in more aligned and successful software solutions.
In the next part of our tutorial series, we will explore other important concepts in DDD, such as repositories, domain events, and bounded contexts, and see how they can be implemented in Go. Stay tuned!