Using Redis with Node.js: Building High-Performance Applications
Learn how to integrate Redis with Node.js to build high-performance applications. Discover Redis caching, session management, and real-time analytics. Boost your app's performance and scalability.
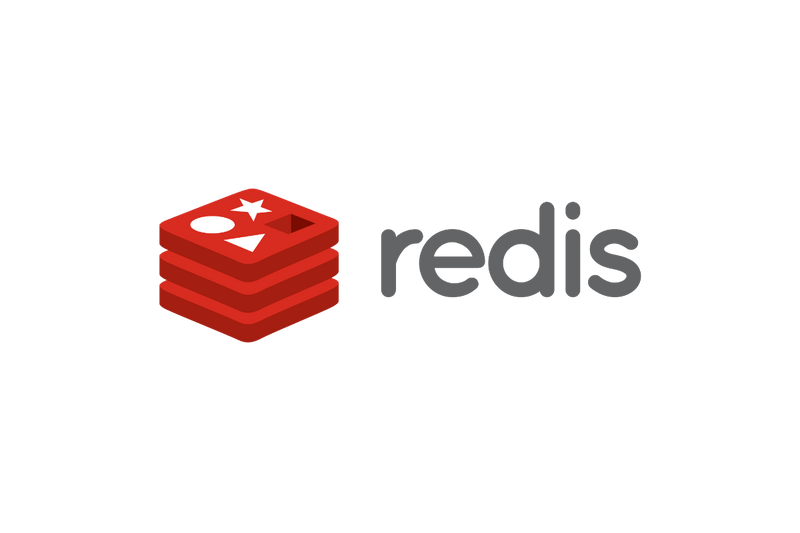
Introduction
Welcome to our guide on using Redis with Node.js to build high-performance applications. Redis is an open-source, in-memory data structure store that is commonly used for caching, session management, and real-time analytics. When combined with Node.js, Redis can greatly enhance the performance and scalability of your applications. In this tutorial, we'll explore the basics of Redis, how to integrate it with Node.js, and some best practices for building high-performance applications.
What is Redis?
Redis is an in-memory data structure store that can be used as a database, cache, and message broker. It supports various data types such as strings, hashes, sets, sorted sets, lists, and more. Redis is known for its exceptional speed and performance, making it an excellent choice for applications that require low-latency data access.
Why Use Redis with Node.js?
Node.js is a popular JavaScript runtime built on Chrome's V8 JavaScript engine. It is known for its non-blocking, event-driven architecture, which makes it highly scalable and efficient. When combined with Redis, Node.js applications can leverage Redis's in-memory caching capabilities to enhance performance and reduce database load.
Here are a few reasons why using Redis with Node.js is beneficial:
- Caching: Redis can be used as a cache for frequently accessed data, reducing the need to make expensive database queries. By storing data in Redis, you can retrieve it quickly, resulting in faster response times for your applications.
- Session Management: Redis can be used to store and manage session data. By keeping session data in Redis, you can easily scale your application across multiple servers without the need for sticky sessions.
- Real-time Analytics: Redis provides support for publish-subscribe messaging, which makes it ideal for building real-time analytics applications. You can use Redis's pub-sub functionality to stream events and update real-time dashboards or perform real-time analytics on the data.
Installing Redis
Before we dive into the integration of Redis with Node.js, let's first install Redis on our system.
To install Redis, follow these steps:
- Visit the Redis download page and download the latest stable version of Redis.
- Extract the downloaded tar file using the following command:
wget http://download.redis.io/releases/redis-x.x.x.tar.gz
tar xzf redis-x.x.x.tar.gz
cd redis-x.x.x
- Compile Redis using the following command:
make
- Run Redis using the following command:
src/redis-server
Once Redis is up and running, you can interact with it using the Redis command-line interface. Open a new terminal window and run the following command:
src/redis-cli
Now that Redis is installed, let's move on to integrating it with Node.js.
Integrating Redis with Node.js
To use Redis with Node.js, we need a Redis client library. There are several available options, but in this tutorial, we'll use node-redis - the official Redis client for Node.js.
Follow these steps to integrate Redis with Node.js:
- Create a new Node.js project using the following command:
mkdir my-redis-app
cd my-redis-app
npm init -y
- Install the
node-redis
library usingnpm
:
npm install redis
Now that we have the Redis client library installed, we can start using Redis in our Node.js application.
Connecting to Redis
The first step is to establish a connection to the Redis server. Use the following code snippet to connect to Redis:
const redis = require('redis');
const client = redis.createClient();
client.on('connect', () => {
console.log('Connected to Redis');
});
client.on('error', (err) => {
console.error('Error connecting to Redis:', err);
});
In the above code, we require the redis
module and create a Redis client using redis.createClient()
. The createClient
method establishes a connection to the default Redis server running on localhost:6379
. We then listen to the connect
and error
events to handle the success and failure of the connection.
Basic Redis Operations
Once the connection to Redis is established, we can perform various operations like setting values, getting values, deleting keys, and more.
Here are some basic Redis operations:
Setting Values
To set a value in Redis, use the set
method:
client.set('key', 'value', (err) => {
if (err) {
console.error('Error setting value in Redis:', err);
} else {
console.log('Value set in Redis');
}
});
The above code sets a value of 'value'
for the key 'key'
in Redis. We pass a callback function to handle any errors that may occur during the operation.
Getting Values
To get a value from Redis, use the get
method:
client.get('key', (err, value) => {
if (err) {
console.error('Error getting value from Redis:', err);
} else {
console.log('Value from Redis:', value);
}
});
The above code retrieves the value for the key 'key'
from Redis. The value is passed as an argument to the callback function.
Deleting Keys
To delete a key in Redis, use the del
method:
client.del('key', (err) => {
if (err) {
console.error('Error deleting key from Redis:', err);
} else {
console.log('Key deleted from Redis');
}
});
The above code deletes the key 'key'
from Redis.
These are just a few of the basic Redis operations. You can explore the node-redis documentation for more advanced operations and features.
Best Practices for Building High-Performance Applications
When using Redis with Node.js, it's important to keep a few best practices in mind to ensure the best performance for your applications.
- Use Caching Strategically: Identify the parts of your application that can benefit from caching and implement caching mechanisms using Redis. Cache frequently accessed data to reduce database load and enhance response times.
- Manage Connections: Redis connections can be costly. Reuse connections by keeping them open as long as possible. Implement connection pooling to improve performance and scalability.
- Use Pipelining and Transactions: Redis allows pipelining multiple commands and executing them as a batch. Use pipelining to reduce round trips and improve performance. Additionally, use Redis transactions to ensure atomicity and consistency.
- Monitor and Optimize: Monitor Redis performance using tools like
redis-cli monitor
andredis-stat
. Analyze performance bottlenecks and optimize Redis usage accordingly.
Conclusion
Using Redis with Node.js can significantly improve the performance and scalability of your applications. Redis's in-memory caching capabilities, when combined with Node.js's event-driven architecture, create a powerful combination for building high-performance applications. In this tutorial, we covered the basics of Redis, how to integrate it with Node.js, and some best practices for building high-performance applications. Armed with this knowledge, you can start incorporating Redis into your Node.js projects and take advantage of its speed and efficiency.
Happy coding!