Integrating gRPC with Kubernetes for Seamless Container Orchestration
Discover how to integrate gRPC, a high-performance RPC framework, with Kubernetes for seamless container orchestration. Achieve scalability and fault tolerance in distributed systems.
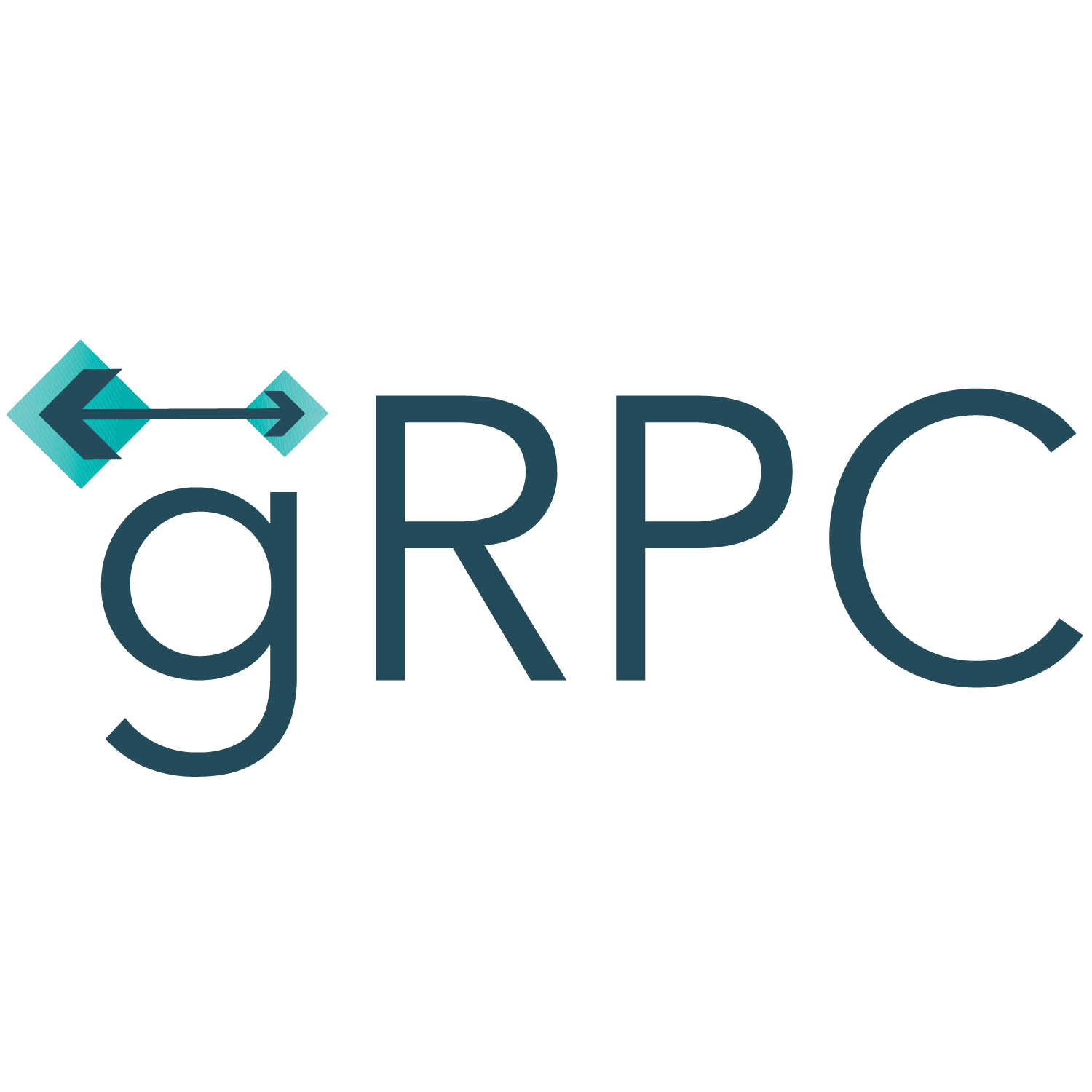
Introduction
Container orchestration has become a fundamental aspect of modern software development. Kubernetes has emerged as the de facto standard for managing containerized applications, offering powerful features for scalability, fault tolerance, and resource allocation. Additionally, gRPC has gained popularity as a high-performance, language-agnostic remote procedure call (RPC) framework. In this blog post, we will explore how to integrate gRPC with Kubernetes to achieve seamless container orchestration. By leveraging both technologies, you can create distributed systems that are scalable, reliable, and efficient.
What is gRPC?
gRPC is an open-source framework developed by Google that allows you to build high-performance and scalable services for connecting distributed systems. It uses the Protocol Buffers (protobuf) serialization format, providing a language-agnostic, platform-independent way to define the structure of your API and generate code for multiple programming languages. With gRPC, you can define your service interfaces using an Interface Definition Language (IDL), and gRPC will generate client and server code that handles all the networking complexities for you.
Integrating gRPC with Kubernetes
Integrating gRPC with Kubernetes involves several steps. Let's walk through the process:
Step 1: Define your gRPC Service
The first step is to define your gRPC service using the protobuf IDL. Define your service methods, data structures, and any required message types. Let's assume you have defined a gRPC service called "UserService" with methods for creating, reading, updating, and deleting user records. Save this protobuf file with a .proto
extension, such as user.proto
.
// user.proto
syntax = "proto3";
package user;
service UserService {
rpc CreateUser(CreateUserRequest) returns (CreateUserResponse) {}
rpc GetUser(GetUserRequest) returns (GetUserResponse) {}
rpc UpdateUser(UpdateUserRequest) returns (UpdateUserResponse) {}
rpc DeleteUser(DeleteUserRequest) returns (DeleteUserResponse) {}
}
message CreateUserRequest {
string name = 1;
string email = 2;
}
message CreateUserResponse {
string userId = 1;
}
message GetUserRequest {
string userId = 1;
}
message GetUserResponse {
string name = 1;
string email = 2;
}
message UpdateUserRequest {
string userId = 1;
string name = 2;
string email = 3;
}
message UpdateUserResponse {}
message DeleteUserRequest {
string userId = 1;
}
message DeleteUserResponse {}
Step 2: Generate Code for your Service
Once you have defined your gRPC service, you need to generate code for your client and server. The protoc
compiler, which is included in the gRPC installation, can generate code in multiple languages. Run the following command to generate the code for your gRPC service:
$ protoc --go_out=plugins=grpc:. user.proto
This command generates Go code for your gRPC service, including the client and server stubs required for communication.
Step 3: Implement the Server
Next, you need to implement the server for your gRPC service. In Go, you can do this by creating a new Go program and importing the generated code for your gRPC service. Implement the methods defined in your protobuf file to fulfill the contract of your gRPC service. Here's an example server implementation for the "UserService" gRPC service:
// server.go
package main
import (
"context"
"log"
"net"
"google.golang.org/grpc"
userpb "path/to/your/generated/code" // Import the generated code
// Implement the UserService server
type userServiceServer struct {
}
// CreateUser implementation
func (*userServiceServer) CreateUser(ctx context.Context, req *userpb.CreateUserRequest) (*userpb.CreateUserResponse, error) {
// Implement the logic to create a user
return &userpb.CreateUserResponse{UserId: "123"}, nil
}
// GetUser implementation
func (*userServiceServer) GetUser(ctx context.Context, req *userpb.GetUserRequest) (*userpb.GetUserResponse, error) {
// Implement the logic to get a user
return &userpb.GetUserResponse{Name: "John", Email: "[email protected]"}, nil
}
// ... Implement other methods
func main() {
lis, err := net.Listen("tcp", ":50051")
if err != nil {
log.Fatalf("Failed to listen: %v", err)
}
s := grpc.NewServer()
userpb.RegisterUserServiceServer(s, &userServiceServer{})
if err := s.Serve(lis); err != nil {
log.Fatalf("Failed to serve: %v", err)
}
}
Implement the necessary logic for your gRPC service methods. In this example, the CreateUser method creates a user and returns the user ID, while the GetUser method retrieves a user based on the given user ID.
Step 4: Containerize the Server
Once you have implemented the server, the next step is to containerize it using Docker. Begin by creating a Dockerfile
in the same directory as your Go code. Here's an example Dockerfile
for our gRPC server:
# Dockerfile
FROM golang:1.17-alpine AS build
WORKDIR /app
COPY . .
RUN go build -o server .
FROM alpine:latest
WORKDIR /app
COPY --from=build /app/server .
CMD ["./server"]
This Dockerfile sets up a multi-stage build process. It first builds the Go server binary and then copies it to a lightweight Alpine-based image, resulting in a minimal and efficient container.
Step 5: Deploy to Kubernetes
Now that you have containerized your gRPC server, it's time to deploy it to Kubernetes. Create a Kubernetes deployment configuration file, such as server-deployment.yaml
, and define the deployment and service specifications. Here's an example configuration:
# server-deployment.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: grpc-server
spec:
replicas: 1
selector:
matchLabels:
app: grpc-server
template:
metadata:
labels:
app: grpc-server
spec:
containers:
- name: grpc-server
image: your-docker-image
ports:
- containerPort: 50051
---
apiVersion: v1
kind: Service
metadata:
name: grpc-server
spec:
selector:
app: grpc-server
ports:
- name: grpc
protocol: TCP
port: 50051
targetPort: 50051
Make sure to replace your-docker-image
in the image
field with the name of your Docker image.
To deploy the gRPC server to Kubernetes, run the following command:
$ kubectl apply -f server-deployment.yaml
Once the deployment is successful, you can access your gRPC server by using the Kubernetes service name and the specified port. In this example, you can use the service name grpc-server
and port 50051
.
Conclusion
Integrating gRPC with Kubernetes allows you to leverage the power of both technologies to build scalable and efficient distributed systems. By defining your gRPC service, generating the necessary code, implementing the server, containerizing it using Docker, and deploying it to Kubernetes, you can seamlessly orchestrate your gRPC services in a highly scalable and fault-tolerant manner. Get started with gRPC and Kubernetes today and embrace the full potential of container orchestration!