Building Real-time Applications with gRPC and WebSockets
Learn how to integrate gRPC and WebSockets to build efficient and scalable real-time applications. Explore the advantages, implementation, and use cases for combining these powerful technologies.
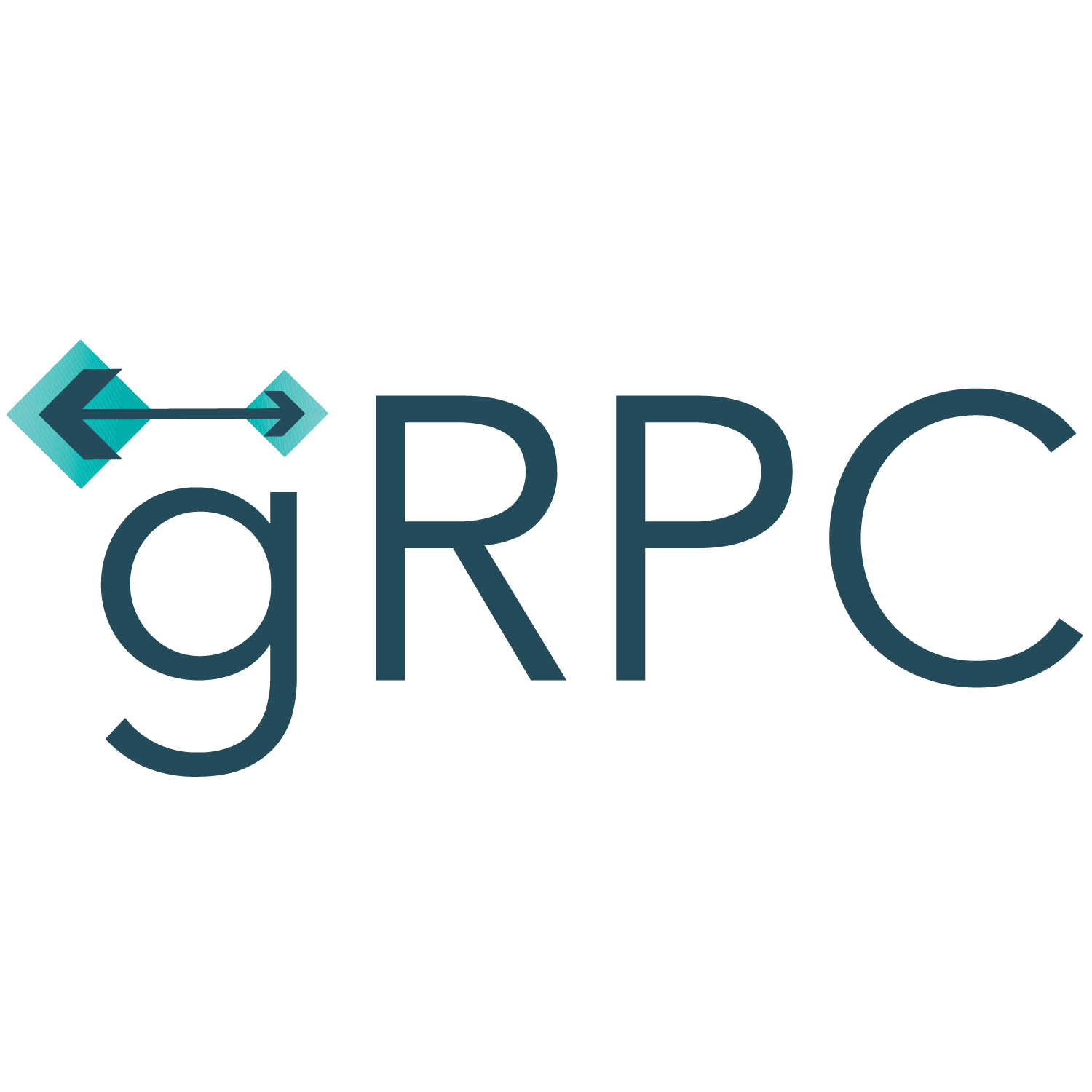
Introduction
Building real-time applications that require high-performance communication between client and server is a common challenge for developers. Two popular technologies that address this challenge are gRPC and WebSockets. In this blog post, we will explore how these two technologies can be used together to build efficient and scalable real-time applications.
What is gRPC?
gRPC is an open-source framework developed by Google for building high-performance and language-agnostic remote procedure call (RPC) systems. It allows you to define the methods and message types for your service using Protocol Buffers, a language-agnostic binary serialization format.
How does gRPC work?
gRPC uses a binary protocol and HTTP/2 as the transport layer. It supports various programming languages, including Java, Go, Python, and C#. When you define your service using Protocol Buffers, gRPC automatically generates client and server stub code in the target language. The client and server can then communicate by making RPC calls using the generated stub code.
Advantages of gRPC
gRPC offers several advantages over other remote procedure call frameworks:
- Efficiency: gRPC uses Protocol Buffers, which results in smaller message sizes and faster serialization and deserialization compared to text-based formats like JSON or XML.
- Performance: gRPC uses HTTP/2 for communication, which allows multiple RPC calls to be multiplexed over a single TCP connection, reducing latency and improving throughput.
- Code generation: gRPC generates client and server stub code, making it easier to write and maintain client-server communication code.
- Bi-directional streaming: gRPC supports bi-directional streaming, allowing both the client and server to send and receive multiple messages over a single RPC call.
- Language support: gRPC supports a wide range of programming languages, making it suitable for building multi-language applications.
What are WebSockets?
WebSockets is a communication protocol that provides full-duplex communication between a client and a server over a single, long-lived connection. Unlike traditional web protocols such as HTTP, which are request-response based, WebSockets allow for real-time bidirectional communication, making them ideal for building real-time applications.
How do WebSockets work?
WebSockets leverage the WebSocket API in web browsers to establish a persistent connection between the client and server. Once the connection is established, either the client or the server can send messages to the other party at any time. This real-time bi-directional communication allows for instant updates and notifications in real-time applications.
Advantages of WebSockets
WebSockets offer several advantages over traditional HTTP-based communication:
- Real-time communication: WebSockets enable real-time bidirectional communication between client and server, allowing for instant updates.
- Efficiency: WebSockets reduce overhead by eliminating the need to send HTTP headers with each request, resulting in lower latency and reduced bandwidth usage.
- Scalability: WebSockets allow for a large number of concurrent connections, making them suitable for building scalable real-time applications.
- Compatibility: WebSockets are supported by all modern web browsers, making them widely compatible.
Integrating gRPC and WebSockets
Now that we understand what gRPC and WebSockets are, let's explore how they can be integrated to build real-time applications. There are different approaches to integrating gRPC and WebSockets, depending on your use case and requirements. Here are two common approaches:
Approach 1: Forwarding gRPC over WebSockets
In this approach, gRPC is used as the main communication protocol, and WebSockets act as a transport layer for gRPC messages. The client establishes a WebSocket connection with the server and sends gRPC messages over the WebSocket connection. On the server side, the gRPC server receives the WebSocket messages and processes them as regular gRPC requests. This approach allows you to leverage the benefits of both gRPC and WebSockets, combining the efficiency and performance of gRPC with the real-time capabilities of WebSockets.
Approach 2: gRPC-based Communication with WebSocket Support
In this approach, gRPC is used as the main communication protocol, and WebSockets are used as an alternative transport layer when necessary. The client and server communicate using gRPC as the primary communication protocol. However, in certain situations where real-time bidirectional communication is required, the client and server can switch to using WebSockets. This approach allows you to take advantage of the efficiency and performance of gRPC for most communication, while still having the flexibility to use WebSockets when needed.
Implementation Example
Let's walk through a simple implementation example to showcase how gRPC and WebSockets can be integrated to build a real-time application. We will use Go for the server-side implementation and JavaScript for the client-side implementation.
Server-side Implementation
First, let's set up the gRPC server using the Go programming language:
import (
"github.com/golang/protobuf/proto"
"github.com/gorilla/websocket"
"google.golang.org/grpc"
)
type Server struct {
// gRPC server implementation
}
func (s *Server) SomeRPC(request *proto.Request, stream proto.Service_SomeRPCServer) error {
// Handle gRPC requests
}
func main() {
// Set up gRPC server
// Handle WebSocket connections and forward messages to the gRPC server
}
Client-side Implementation
Next, let's implement the client-side using JavaScript:
const socket = new WebSocket("ws://localhost:8080");
socket.onopen = function() {
// WebSocket connection established
}
socket.onmessage = function(event) {
// Handle WebSocket messages
}
socket.onclose = function() {
// WebSocket connection closed
}
function sendWebSocketMessage(message) {
// Send message over WebSocket
}
// Send gRPC request over WebSocket
const request = { ... };
const message = createWebSocketMessage(request);
sendWebSocketMessage(message);
Conclusion
Integrating gRPC and WebSockets allows you to combine the efficiency and performance of gRPC with the real-time capabilities of WebSockets, providing an excellent foundation for building scalable and efficient real-time applications. Whether you choose to forward gRPC over WebSockets or use WebSockets for specific communication scenarios, understanding these technologies opens up new possibilities for building modern and interactive applications.
Now that you have a solid understanding of building real-time applications with gRPC and WebSockets, you can start exploring these technologies further, experimenting with different use cases and architectural patterns. Happy coding!