Integrating Databases in Golang: A Complete Guide
Discover how to integrate databases in Golang with this complete guide. Learn about popular databases, installing drivers, connecting to databases, performing operations, and handling errors. Perfect for beginners in Golang development.
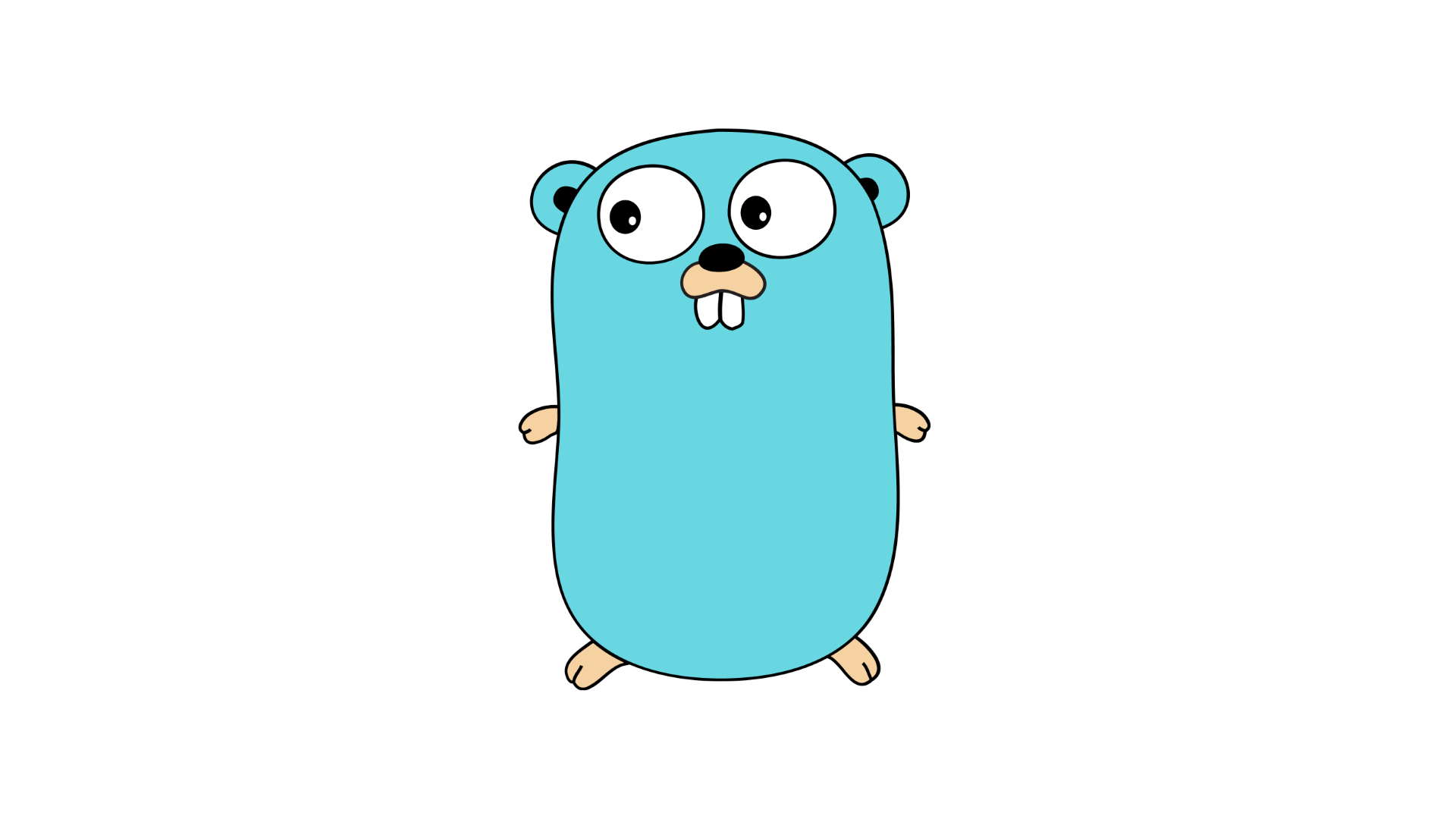
Introduction
Integrating databases in Golang can be a complex task, especially for beginners. However, with the right guidance, you can overcome the challenges and effectively integrate databases into your Golang applications. In this complete guide, we will walk you through the process of integrating databases in Golang, providing you with the knowledge and tools you need to succeed.
Choosing the Right Database
Before we begin integrating databases in Golang, it is important to choose the right database for your project. There are several popular databases that are commonly used with Golang, including MySQL, PostgreSQL, and MongoDB. Each database has its own strengths and weaknesses, so it is important to evaluate your project's requirements and choose the database that best fits your needs.
MySQL
MySQL is a widely-used relational database management system that is known for its reliability and scalability. It supports complex queries and has a large community of developers, making it a popular choice for Golang projects. To integrate MySQL with Golang, you will need to install the necessary MySQL driver and configure your Golang application to connect to the MySQL database.
PostgreSQL
PostgreSQL is another powerful and feature-rich relational database management system. It is known for its advanced features, such as support for complex data types and full-text search. Integrating PostgreSQL with Golang is similar to integrating MySQL, but you will need to use the appropriate PostgreSQL driver and configure your Golang application accordingly.
MongoDB
MongoDB is a popular document-oriented database that is designed for flexibility and scalability. It is known for its ability to handle unstructured data and its support for horizontal scaling. To integrate MongoDB with Golang, you will need to install the MongoDB driver and configure your Golang application to interact with the MongoDB database.
Installing the Database Drivers
Once you have chosen a database, the next step is to install the necessary database drivers for Golang. Each database has its own driver, which allows Golang to communicate with the database.
Installing the MySQL Driver
To install the MySQL driver for Golang, you can use the following command:
go get -u github.com/go-sql-driver/mysql
Installing the PostgreSQL Driver
To install the PostgreSQL driver for Golang, you can use the following command:
go get -u github.com/lib/pq
Installing the MongoDB Driver
To install the MongoDB driver for Golang, you can use the following command:
go get -u go.mongodb.org/mongo-driver/mongo
Connecting to the Database
Once you have installed the necessary drivers, you can start connecting to the database in your Golang application. The process of connecting to the database will vary depending on the database you are using.
Connecting to MySQL
To connect to a MySQL database in Golang, you will need to provide the necessary connection details, such as the host, port, username, password, and database name. You can use the following code snippet as a starting point:
// import the required packages
import (
"database/sql"
_ "github.com/go-sql-driver/mysql"
)
func main() {
// open a connection to the database
db, err := sql.Open("mysql", "user:password@tcp(host:port)/database")
if err != nil {
panic(err.Error())
}
defer db.Close()
// perform database operations
// ...
}
Connecting to PostgreSQL
Connecting to a PostgreSQL database in Golang is similar to connecting to MySQL. You will need to provide the appropriate connection details, such as the host, port, username, password, and database name. Here is an example:
// import the required packages
import (
"database/sql"
_ "github.com/lib/pq"
)
func main() {
// open a connection to the database
db, err := sql.Open("postgres", "host=host port=port user=user password=password dbname=database")
if err != nil {
panic(err.Error())
}
defer db.Close()
// perform database operations
// ...
}
Connecting to MongoDB
Connecting to a MongoDB database in Golang requires the MongoDB driver. You will need to provide the appropriate connection details, such as the MongoDB URI. Here is an example:
// import the required packages
import (
"context"
"go.mongodb.org/mongo-driver/mongo"
"go.mongodb.org/mongo-driver/mongo/options"
)
func main() {
// create a context
ctx := context.TODO()
// connect to the database
client, err := mongo.Connect(ctx, options.Client().ApplyURI("mongodb://localhost:27017"))
if err != nil {
panic(err.Error())
}
defer client.Disconnect(ctx)
// perform database operations
// ...
}
Performing Database Operations
Once you have connected to the database, you can start performing various database operations, such as inserting, updating, and querying data. The specific operations will depend on your project's requirements and the database you are using.
Inserting Data
To insert data into the database, you will need to create a SQL or MongoDB query and execute it using the appropriate methods provided by the database driver.
Updating Data
To update data in the database, you will need to create a SQL or MongoDB query that specifies the data you want to update and execute it using the appropriate methods provided by the database driver.
Querying Data
To query data from the database, you will need to create a SQL or MongoDB query that specifies the data you want to retrieve and execute it using the appropriate methods provided by the database driver.
Handling Errors
When integrating databases in Golang, it is important to handle errors properly. Database operations can fail for various reasons, such as network issues, incorrect credentials, or server errors. By handling errors correctly, you can provide a better user experience and ensure the stability of your application.
Conclusion
Integrating databases in Golang can be a challenging task, especially for beginners. However, with the right guidance and knowledge, you can effectively integrate databases into your Golang applications. By choosing the right database, installing the necessary drivers, connecting to the database, performing database operations, and handling errors, you will be able to create robust and reliable Golang applications that interact with databases seamlessly.
We hope this complete guide has provided you with the necessary information to get started with integrating databases in Golang. Happy coding!