Apache Kafka and Apache Spark Integration: Stream Processing
Learn how to integrate Apache Kafka and Apache Spark for real-time stream processing. Explore the benefits, setup steps, and write a simple Spark Streaming application to consume Kafka data.
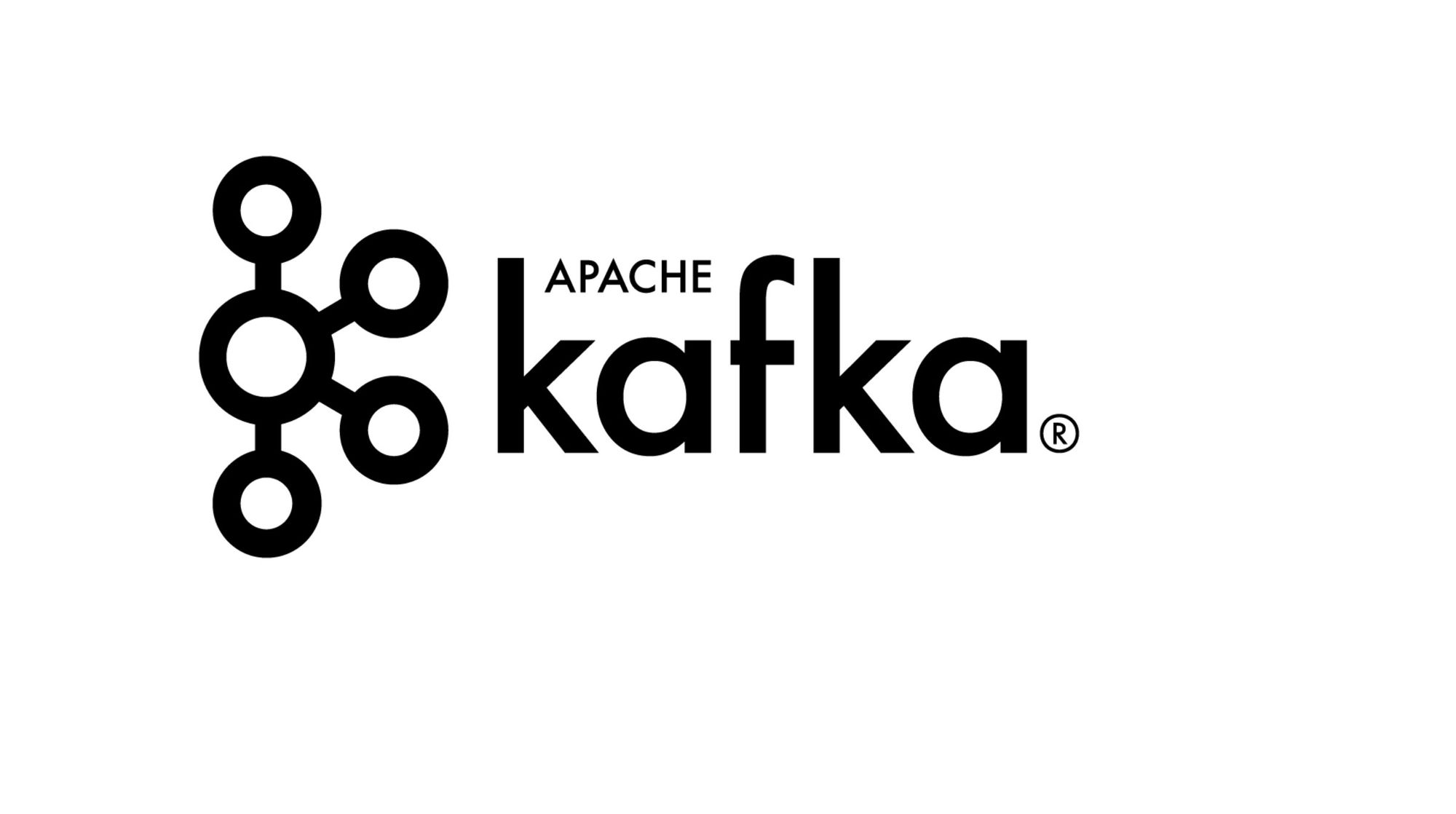
Introduction
In today's era of big data, organizations are constantly collecting vast amounts of data from various sources. Processing and analyzing this data in real-time has become crucial for making informed business decisions. Apache Kafka and Apache Spark are two powerful tools commonly used for data processing and analysis. In this blog post, we will explore the integration of Apache Kafka and Apache Spark for stream processing.
What is Apache Kafka?
Apache Kafka is a distributed streaming platform that enables the publishing and subscribing of real-time data feeds. It provides a fault-tolerant, scalable, and highly available architecture for handling streaming data.
Kafka works on the publish-subscribe model, where producers publish messages to topics, and consumers subscribe to these topics to receive the messages. It allows data to be stored durably and in a fault-tolerant manner, enabling multiple consumers to process the data at their own pace.
What is Apache Spark?
Apache Spark is an open-source, general-purpose distributed computing system used for big data processing and analytics. It provides various high-level APIs for handling structured and unstructured data and offers support for batch processing, stream processing, machine learning, and graph processing.
Spark's main programming abstraction is the resilient distributed dataset (RDD), which allows parallel and fault-tolerant processing of data across a cluster of computers.
Why Integrate Apache Kafka and Apache Spark?
The integration of Apache Kafka and Apache Spark brings the benefits of both tools together, creating a powerful stream processing solution. Here are a few reasons why integrating Kafka and Spark is advantageous:
- Real-time data processing: Kafka provides a reliable and scalable method for ingesting and streaming data in real-time, while Spark enables fast and distributed processing of this data, allowing for real-time analytics and insights.
- Scalability: Both Kafka and Spark are horizontally scalable, meaning they can handle large volumes of data by distributing the workload across multiple machines.
- Fault-tolerance: Kafka and Spark are designed to be fault-tolerant, ensuring that data is not lost even in the event of system failures.
- Flexibility: Kafka acts as a buffering layer between data producers and consumers, allowing the decoupling of data ingestion and processing. This gives developers the flexibility to choose the right tools for different stages of data processing.
Integrating Apache Kafka and Apache Spark
Now that we understand the benefits of integrating Apache Kafka and Apache Spark, let's explore how to set up this integration.
Step 1: Set up Apache Kafka
To start, you need to set up Apache Kafka. This involves downloading and installing Kafka on your system, configuring the necessary properties, and starting the Kafka server.
You can download Apache Kafka from the official Apache Kafka website (https://kafka.apache.org/). Follow the installation instructions specific to your operating system.
# Start ZooKeeper server bin/zookeeper-server-start.sh config/zookeeper.properties
# Start Kafka server bin/kafka-server-start.sh config/server.properties
Step 2: Set up Apache Spark
Next, you need to set up Apache Spark. This involves downloading and installing Spark on your system and configuring the necessary properties.
You can download Apache Spark from the official Apache Spark website (https://spark.apache.org/). Follow the installation instructions specific to your operating system.
Step 3: Add the Spark Streaming Kafka Dependency
To integrate Kafka and Spark, you need to add the spark-streaming-kafka
dependency to your Spark project. This enables Spark to consume data from Kafka topics.
Add the following Maven dependency to your pom.xml
file:
<dependency> <groupId>org.apache.spark</groupId> <artifactId>spark-streaming-kafka-0-10_2.12</artifactId> <version>3.1.2</version> </dependency>
Step 4: Write a Spark Streaming Application
Now it's time to write a Spark Streaming application that consumes data from Kafka topics and performs the desired processing and analytics.
Here's an example of how to create a simple Spark Streaming application that consumes Kafka data:
import org.apache.spark.streaming._ import org.apache.spark.streaming.kafka010._ val sparkConf = new SparkConf().setMaster("local[2]").setAppName("KafkaSparkStreaming") val streamingContext = new StreamingContext(sparkConf, Seconds(1)) val kafkaParams = Map[String, Object]( "bootstrap.servers" -> "localhost:9092", "key.deserializer" -> classOf[StringDeserializer], "value.deserializer" -> classOf[StringDeserializer], "group.id" -> "spark-kafka-streaming-example", "auto.offset.reset" -> "latest", "enable.auto.commit" -> (false: java.lang.Boolean) ) val topics = Array("topic1", "topic2") val kafkaStream = KafkaUtils.createDirectStream[String, String]( streamingContext, PreferConsistent, Subscribe[String, String](topics, kafkaParams) ) kafkaStream.foreachRDD { rdd => rdd.foreach { record => val value = record.value() // Process the received data // Perform analytics or any desired operations println(value) } } streamingContext.start() streamingContext.awaitTermination()
Step 5: Run the Spark Streaming Application
Once you have written the Spark Streaming application, you can submit it to the Spark cluster for execution. Use the following command to run the application:
./bin/spark-submit --class com.example.KafkaSparkStreaming --master spark://your-spark-master:7077 path/to/your-spark-streaming-application.jar
Conclusion
Apache Kafka and Apache Spark are two powerful tools for stream processing and data analytics. By integrating Kafka and Spark, you can build scalable and fault-tolerant stream processing pipelines to handle real-time data and derive valuable insights.
In this blog post, we explored the benefits of integrating Kafka and Spark, the steps to set up an integration, and how to write a simple Spark Streaming application that consumes data from Kafka. With this knowledge, you can start building your own real-time stream processing solutions using Kafka and Spark.
Happy stream processing!