Applying Value-Object-Oriented Programming in Golang with Domain-Driven Design
Applying Value-Object-Oriented Programming (VOOP) in Golang with Domain-Driven Design (DDD) can enhance code robustness and maintainability by encapsulating related data and behavior into reusable components. Learn how to implement value objects in Go!
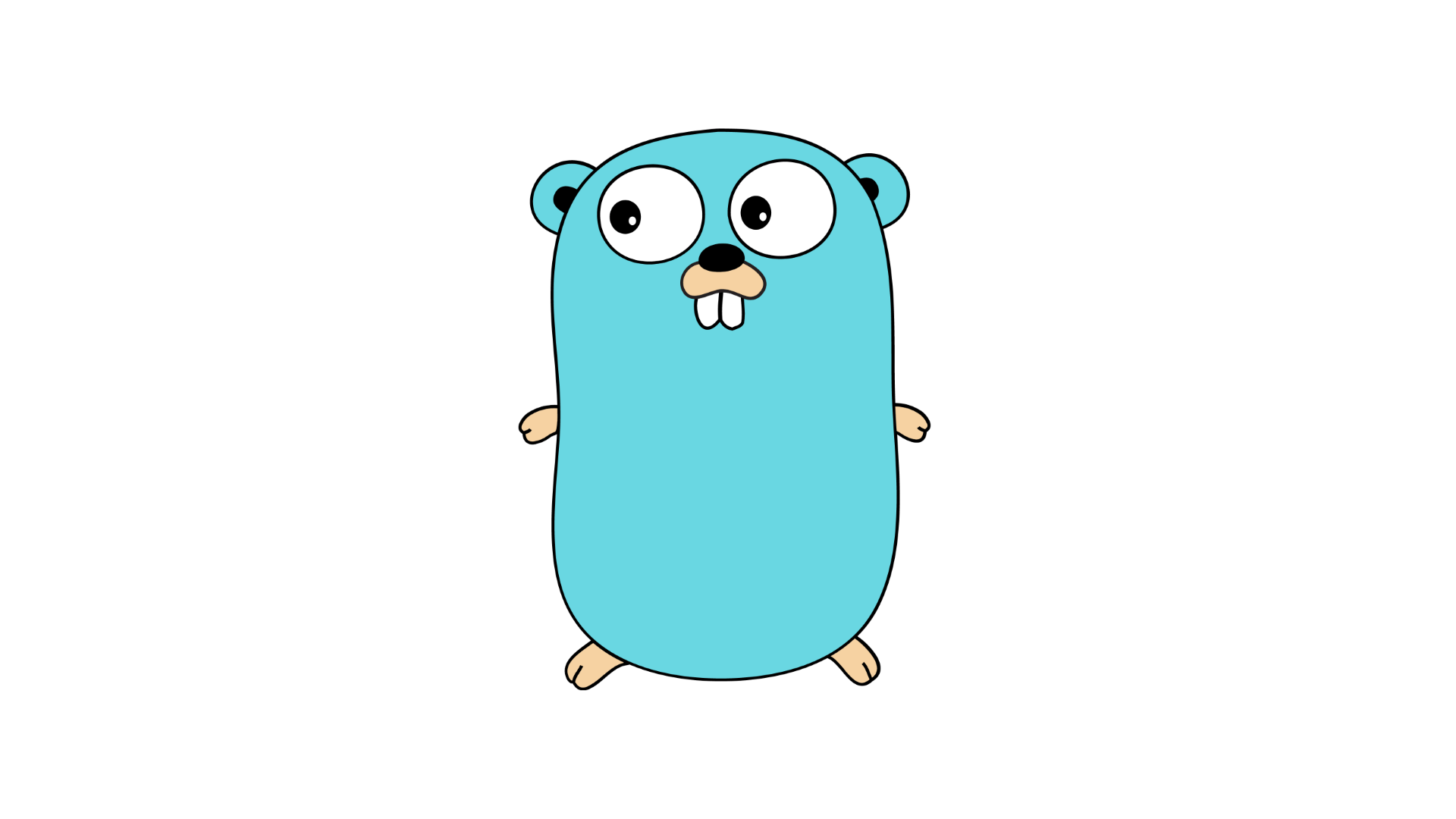
Introduction
Applying Value-Object-Oriented Programming (VOOP) in Golang with Domain-Driven Design (DDD) can significantly enhance the effectiveness and maintainability of your Go applications. By leveraging the power of value objects, you can create more robust and scalable codebases that align with DDD principles. In this blog post, we'll explore the concept of value objects, understand their benefits, and learn how to implement them in Go using DDD. Let's dive in!
What are Value Objects?
In the context of software development, a value object is an immutable object that represents a concept and carries no identity. Unlike entities, which are distinguished by their identity, value objects are defined by their attributes. They are self-contained, meaning that their values fully define their meaning and purpose.
Value objects are typically used to model complex data types with behavior. For example, consider a Date value object that encapsulates date-related functionality like date validation, calculations, and comparisons. With value objects, you can encapsulate related data and behavior into reusable components and improve the overall design of your application.
Benefits of Value Objects
Value objects offer numerous benefits to the development process. Here are some key advantages:
1. Encapsulation
Value objects encapsulate related data and behavior into a single cohesive unit. By bundling attributes and methods together, you improve the organization and maintainability of your code. Encapsulation enables better domain modeling by aligning the code with the conceptual boundaries of your application.
2. Immutable and Side-Effect Free
Value objects are immutable, meaning their internal state cannot be changed after creation. This immutability ensures side-effect-free operations, making value objects predictable and preventing unwanted changes. Immutable value objects simplify concurrency and facilitate safe sharing of data.
3. Equality by Value
Value objects are compared based on their internal values rather than their references. This facilitates easy equality comparisons without the need for complex logic. By using value objects, you can focus on the attributes and behavior rather than tracking identity.
4. Domain Validation
Value objects embody domain logic and can perform internal validation to ensure data integrity. By centralizing validation within value objects, you avoid scattered validation checks across your codebase. This promotes consistent data validation and improves the reliability of your application.
Implementing Value Objects in Golang
Now, let's dive into implementing value objects in Golang. Here's a step-by-step guide:
Step 1: Define the Value Object
Start by defining a new Go struct to represent your value object. For example, let's create a Email
value object:
type Email struct {
value string
}
In this example, the Email
struct has a single field, value
, which represents the email address as a string.
Step 2: Add Validations and Behavior
Next, add the necessary validations and behavior to the value object. For instance, you can add a validation method to ensure the email address is valid:
func (e Email) IsValid() bool {
// Perform email validation logic
}
By encapsulating validation within the value object, you ensure that any instance of the Email
struct can validate its own integrity.
Step 3: Implement Equality Comparison
To enable equality comparisons, implement the Equals
method for your value object:
func (e Email) Equals(other Email) bool {
// Perform equality comparison logic
}
By comparing the internal values of both Email
objects, you can determine if they are equal.
Step 4: Make the Value Object Immutable
For immutability, ensure that the fields of your value object are not directly accessible outside the struct. You can achieve this by making the fields unexported (i.e., lowercase). To provide access to the field's value, add a getter method:
func (e Email) Value() string {
return e.value
}
With this setup, the value object remains immutable, and changes can only be made through controlled methods.
Using Value Objects in Domain-Driven Design
When using DDD, value objects play a pivotal role in modeling the various concepts within your domain. They help define the boundaries, constraints, and behavior of specific concepts, making your codebase more expressive and domain-centric.
Here's an example of how you can leverage value objects in DDD:
type User struct {
ID UserID
Email Email
// Other user-related fields
}
In this example, the User
struct includes the Email
value object, which ensures that the user's email remains valid and can be consistently validated across the application.
Using Value Objects in Domain Logic
Value objects can also be used to encapsulate domain logic. For example, consider a Money
value object that represents a specific amount of money:
type Money struct {
amount float64
currency string
}
func (m Money) Add(other Money) Money {
// Perform money addition logic
}
In this example, the Money
value object encapsulates the logic for adding two instances of Money
together, ensuring currency conversions and handling numerical operations accurately.
Conclusion
By embracing value-object-oriented programming in Golang with Domain-Driven Design, you can create more expressive and maintainable code. Value objects facilitate encapsulation, immutability, equality comparisons, and domain validation, promoting cleaner and more robust domain models. Start leveraging value objects in your Go applications today and see the positive impact they have on your codebase.
Thank you for reading this blog post! Stay tuned for more insightful content on Golang and software development best practices!