Implementing the Repository Pattern in Golang for Domain-Driven Design
Learn how to implement the Repository Pattern in Golang for Domain-Driven Design. Discover the benefits, steps to implement, and code structure for clean and maintainable applications.
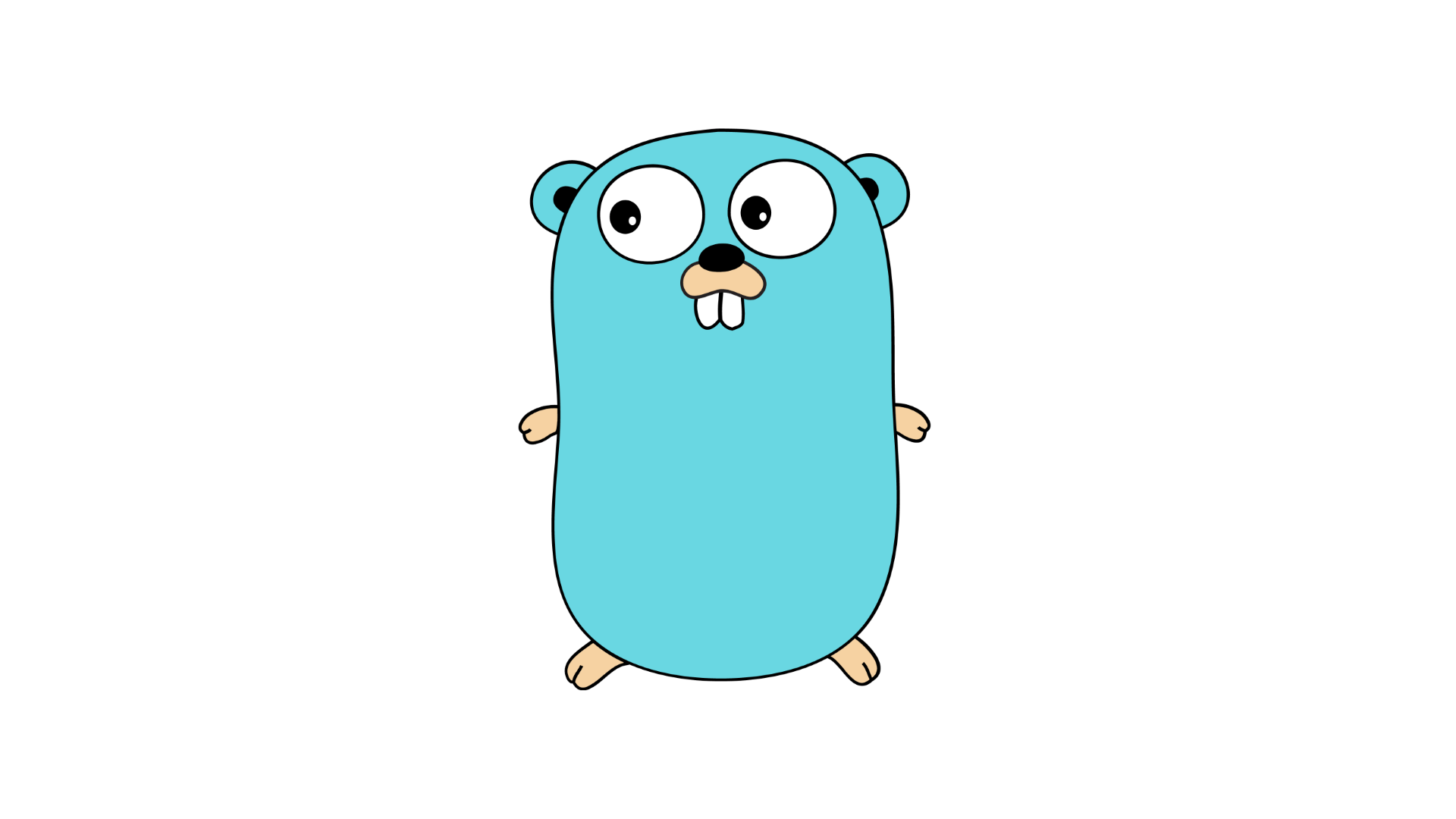
Introduction
The Repository Pattern is a popular design pattern used in software development to decouple the application's business logic from the data access layer. It provides an abstraction layer that allows you to leverage domain-specific objects and operations, while keeping the implementation details of data persistence hidden.
In this article, we will explore how to implement the Repository Pattern in Golang for Domain-Driven Design. We will cover the benefits of using this pattern, the core components involved, and how to structure your code for a clean and maintainable implementation.
What is the Repository Pattern?
The Repository Pattern is a part of Domain-Driven Design (DDD) and is commonly used to achieve separation of concerns between the business logic and the data access layer. It provides a way to encapsulate the underlying data operations and abstracts them behind interfaces that are specific to the application's domain. This creates a clear separation between the domain objects and the way they are persisted.
The core idea behind the Repository Pattern is to create a layer of repositories that act as a collection-like abstraction for querying and manipulating domain objects. These repositories then encapsulate the details of data persistence, such as querying a database or making requests to an external API.
Benefits of Using the Repository Pattern
Implementing the Repository Pattern in your Golang applications brings several benefits:
- Separation of Concerns: By encapsulating data access behind repositories, you can isolate domain logic from persistence-related concerns.
- Testability: Repositories can be easily mocked or stubbed, allowing you to write unit tests without accessing the actual data storage.
- Flexibility: With the Repository Pattern, you can easily switch between different data storage technologies or APIs without affecting the rest of your application. This makes your code more adaptable to changes in requirements or technologies.
- Maintainability: Centralizing data access logic in repositories promotes code reuse and helps maintain a clean and consistent codebase.
Implementing the Repository Pattern in Golang
Now let's dive into the implementation details. We will go through the steps to implement the Repository Pattern in Golang:
Step 1: Define the Repository Interface
The first step in implementing the Repository Pattern is to define the repository interface. The interface should include the common CRUD (Create, Read, Update, Delete) operations relevant to your domain:
type UserRepository interface {
GetByID(id int) (*User, error)
GetAll() ([]*User, error)
Create(user *User) error
Update(user *User) error
Delete(id int) error
}
Here, we define a UserRepository interface that specifies the methods for performing operations on user objects. You can customize this interface based on the specific needs of your application.
Step 2: Implement the Repository
Once the repository interface is defined, you can start implementing the repository. The repository implementation will handle the actual data access and manipulation:
type UserRepositoryImpl struct {
db *sql.DB
}
func NewUserRepository(db *sql.DB) *UserRepositoryImpl {
return &UserRepositoryImpl{db: db}
}
func (r *UserRepositoryImpl) GetByID(id int) (*User, error) {
// Implementation logic to fetch a user by ID from the database
}
// Implement the other repository methods: GetAll, Create, Update, and Delete
In the above code, we create a UserRepositoryImpl struct that contains the database connection object. We also define a NewUserRepository function to create a new instance of the UserRepositoryImpl by passing in the database connection. Each method of the UserRepositoryImpl implements the corresponding method from the UserRepository interface and handles the data access accordingly.
Step 3: Wire Up the Repository
Finally, we need to wire up the repository in our application. This involves injecting the repository instance into the appropriate parts of your codebase. One common approach is to utilize a dependency injection container or a package like wire
to manage the dependencies:
func InitializeUserAPI(db *sql.DB) *UserAPI {
userRepo := NewUserRepository(db)
userAPI := &UserAPI{
UserRepository: userRepo,
}
return userAPI
}
func main() {
// Initialize the database connection
db := initializeDatabase()
// Initialize the user API with the UserRepository dependency
userAPI := InitializeUserAPI(db)
// Use the userAPI instance in your application logic
// ...
}
In this example, we create an InitializeUserAPI
function that takes in the database connection and initializes the user repository (UserRepository
) and the user API (UserAPI
). We can then use the userAPI
instance in our application logic.
Structuring Your Code for DDD
When implementing the Repository Pattern for Domain-Driven Design, it's important to consider the overall structure of your code. Here's a recommended structure:
- domain/
- user.go // User domain object
- user_repository.go // UserRepository interface
- infrastructure/
- user_repository_impl.go // UserRepository implementation
- main.go
In this structure, the domain
package contains the domain objects and the repository interface. The infrastructure
package contains the repository implementation. This separation ensures that the domain logic remains independent of the data access implementation details.
Conclusion
In this article, we explored how to implement the Repository Pattern in Golang for Domain-Driven Design. By following the steps outlined, you can achieve clean separation of concerns, improve testability, and make your codebase more maintainable and adaptable.
Remember to always analyze your application's requirements and design your repository interfaces accordingly. This will ensure that you create a repository layer that suits your application's specific needs.
Happy coding!