Implementing Rate Limiting with Redis: Throttling API Requests
Rate limiting with Redis helps control incoming requests, prevent abuse, and maintain system stability. Learn how to implement it in this blog post using Python and Redis.
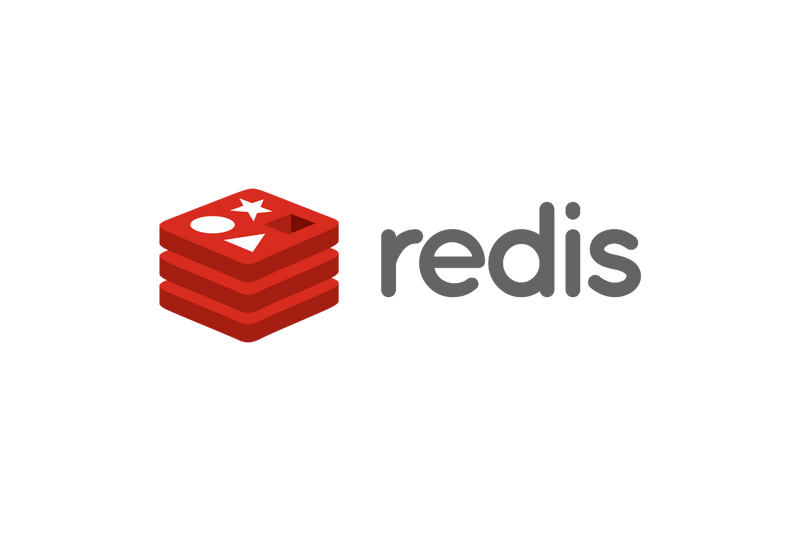
Introduction
Rate limiting is an important technique used to control the flow of incoming requests to a server. It helps prevent abuse, protect server resources, and maintain overall system stability. In this blog post, we will explore how to implement rate limiting with Redis, a popular in-memory data store.
What is Rate Limiting?
Rate limiting is a mechanism that restricts the number of requests a client can make to a server within a specified time period. It helps protect the server from being overwhelmed by a sudden influx of requests, and ensures fair usage of resources.
Rate limiting is commonly used in various scenarios:
- APIs: To prevent abuse and maintain quality of service.
- Login and registration systems: To prevent brute-force attacks.
- Content delivery networks (CDNs): To manage bandwidth usage.
- Payment gateways: To prevent fraud and reduce risk.
To implement rate limiting, we need a system that can store and track request information, such as the number of requests made by a client and the time at which each request was made. Redis, with its fast in-memory operations and built-in data structures, provides an excellent solution for this purpose.
Implementing Rate Limiting with Redis
Let's see how we can use Redis to implement rate limiting. We will build a simple rate limiter for an API endpoint, limiting clients to a certain number of requests per minute.
Step 1: Configure Redis
First, make sure you have Redis installed and running on your system. You can download it from the official Redis website and follow the installation instructions.
Once Redis is up and running, you can connect to it using the Redis CLI by running the following command:
redis-cli
If Redis is running on a different host or port, you can specify the connection details using the following command:
redis-cli -h <hostname> -p <port>
Step 2: Implement the Rate Limiter
Now let's write some code to implement the rate limiter using Redis.
We will use the SETNX (set if not exists) command in Redis to keep track of the number of requests made by each client. We will create a Redis key for each client, with a TTL (time-to-live) set to one minute. This key will store the number of requests made by the client.
Here's an example implementation in Python:
import redis
import time
def is_allowed(api_key):
client = redis.Redis(host='localhost', port=6379, db=0)
key = f"rate_limit:{api_key}"
# Check if rate limit key exists
if client.exists(key):
# Increment the request count
client.incr(key)
else:
# Create the rate limit key
client.setex(key, 60, 1)
# Get the current request count
request_count = int(client.get(key))
# Check if the request count exceeds the limit
if request_count > 100:
return False
return True
# Example usage
api_key = "your_api_key"
if is_allowed(api_key):
print("Request allowed")
else:
print("Request blocked")
This code uses the redis-py
library to interact with Redis. Make sure you have it installed by running pip install redis
.
The is_allowed
function checks if the client's rate limit key exists. If it does, it increments the request count. If not, it creates the rate limit key with an initial count of 1 and a TTL of one minute.
The function then gets the current request count from Redis and checks if it exceeds the limit (in this example, 100 requests per minute). If the limit is exceeded, the function returns False
, indicating that the request should be blocked. Otherwise, it returns True
, allowing the request to proceed.
You can customize the rate limit by changing the limit value in the code. You can also modify the Redis connection details as per your setup.
Conclusion
Implementing rate limiting with Redis provides an efficient and scalable solution for controlling the flow of incoming requests to your server. By using Redis's fast in-memory operations and built-in data structures, you can easily track and limit the number of requests made by clients.
In this blog post, we learned how to implement a simple rate limiter using Redis and Python. However, this is just a basic example, and there are many other techniques and approaches you can explore to implement rate limiting.
Remember to experiment, test, and fine-tune your rate limiting implementation based on your specific requirements. Rate limiting can play a crucial role in maintaining the stability, security, and performance of your applications and services.
So go ahead, give it a try, and start implementing rate limiting with Redis today!