Event-Driven Architecture in Golang: A Domain-Driven Approach
Event-driven architecture in Golang enables scalable, decoupled, and resilient systems. Learn how to implement it using a domain-driven approach for flexible and maintainable applications.
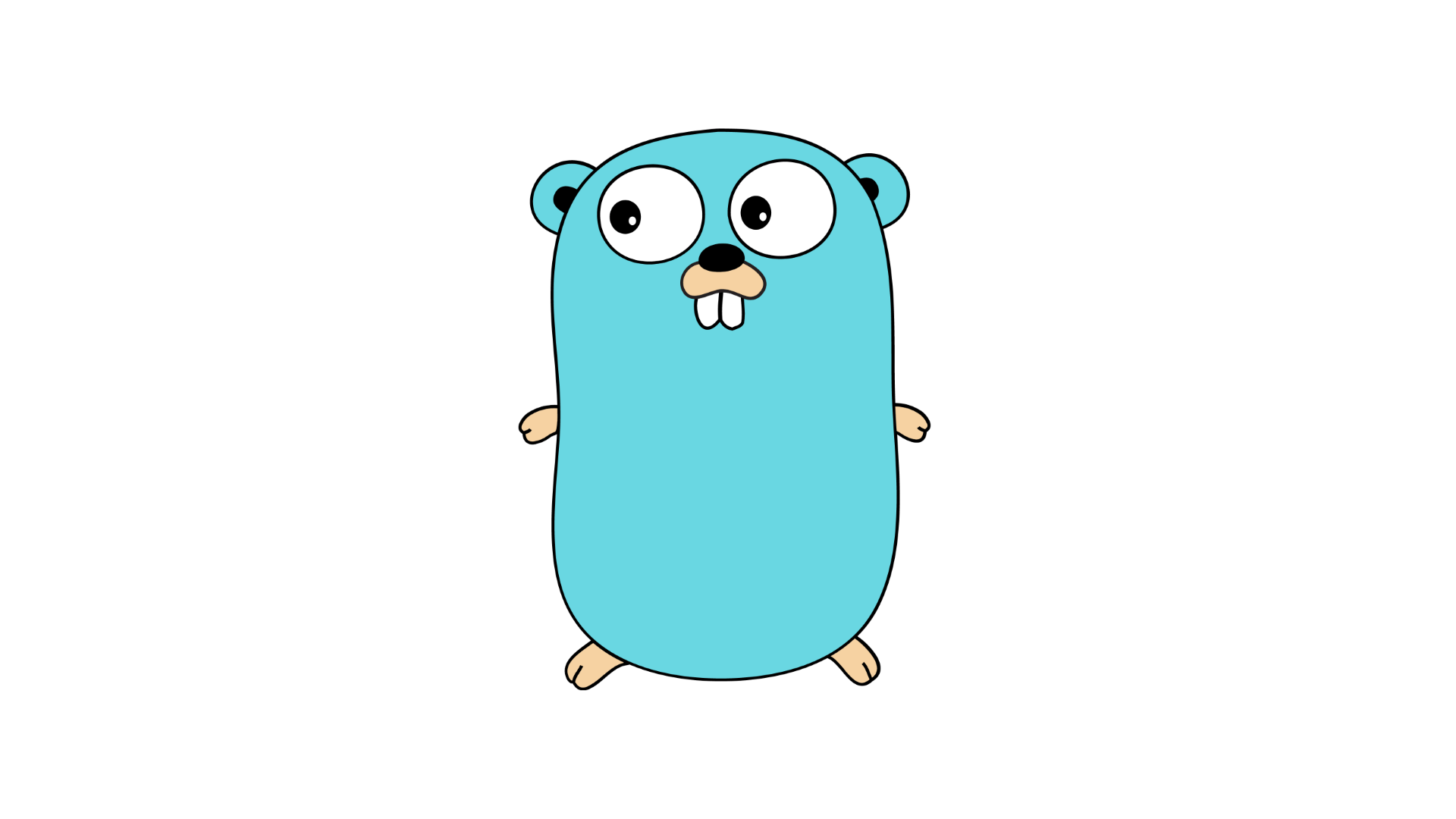
Introduction
Event-driven architecture (EDA) is a popular approach in software development that enables loosely coupled and scalable systems. By leveraging events, EDA focuses on the flow of information between different components of an application, allowing them to react and respond to changes effectively. In this blog post, we'll explore how event-driven architecture can be implemented in Golang with a domain-driven approach. Let's dive in!
What is Event-Driven Architecture?
Event-driven architecture is a software design pattern where system components communicate through the exchange of events. An event is a significant occurrence or change in the system, such as the creation of a new record in a database, a user action, or an external system integration. Instead of traditional synchronous communication, where components explicitly call each other's methods, event-driven systems rely on events to trigger actions.
Event-driven architecture offers several benefits:
- Decoupling: Components are decoupled, meaning they can evolve independently and be replaced or added without affecting the entire system.
- Scalability: Event-driven systems are inherently scalable as events can be processed in parallel.
- Resilience: If a component fails, events can be captured and reprocessed.
- Flexibility: New functionalities or integrations can be easily added by listening to new or existing events.
Event-Driven Architecture in Golang
Golang's simplicity, concurrency model, and robust standard library make it a great choice for implementing event-driven architectures. Let's explore how we can apply event-driven architecture principles in Golang:
1. Define Domain Events
In a domain-driven design approach, we start by identifying the main concepts (entities) and their interactions (aggregate roots, value objects, etc.) within the domain. Once we have a clear understanding of the domain, we can define domain events. Domain events represent significant occurrences or changes in the domain.
For example, in an e-commerce application, we might have the following domain events:
type OrderCreated struct {
OrderID string
CustomerID string
Total float64
// ... additional fields
}
type OrderPaid struct {
OrderID string
// ... additional fields
}
type OrderCancelled struct {
OrderID string
// ... additional fields
}
These events capture essential business events in our e-commerce domain. Each event contains relevant data necessary for processing it.
2. Define Event Handlers
Event handlers are responsible for reacting to specific events and performing the necessary actions. In Golang, event handlers can be implemented as methods on event listeners or separate functions.
Let's define event handlers for our e-commerce scenario:
func HandleOrderCreated(event OrderCreated) {
// Perform actions on order creation event
// e.g., send confirmation email, update inventory, etc.
}
func HandleOrderPaid(event OrderPaid) {
// Perform actions on order paid event
// e.g., mark order as paid, send receipt, etc.
}
func HandleOrderCancelled(event OrderCancelled) {
// Perform actions on order cancellation event
// e.g., refund customer, update inventory, etc.
}
These event handlers encapsulate the logic associated with each event. They can be separate functions or methods on specific handlers or services.
3. Dispatch Events
Once we have defined our domain events and event handlers, we need to dispatch events when relevant actions occur.
Following our e-commerce example, when an order is created, we dispatch the OrderCreated
event:
func CreateOrder(orderID, customerID string, total float64) {
// Perform order creation logic
// Dispatch OrderCreated event
event := OrderCreated{
OrderID: orderID,
CustomerID: customerID,
Total: total,
}
eventBus.Dispatch(event)
}
The eventBus.Dispatch
method ensures that the event is delivered to the appropriate event handlers. It can be implemented using channels, message brokers, or a predefined event bus.
4. Handler Registration
To connect events with their corresponding event handlers, we need to register the handlers with the event bus. This step allows the event bus to know which handlers should receive specific events.
For example, we can register our event handlers like this:
func RegisterEventHandlers() {
eventBus.Register(OrderCreated{}, HandleOrderCreated)
eventBus.Register(OrderPaid{}, HandleOrderPaid)
eventBus.Register(OrderCancelled{}, HandleOrderCancelled)
}
The Register
method associates an event type with its corresponding handler function. This registration ensures that when the event occurs, the handler is invoked automatically.
Conclusion
By implementing event-driven architecture using a domain-driven approach in Golang, you can build flexible, scalable, and maintainable systems. Golang's simplicity and concurrency features provide a solid foundation for developing event-driven applications. Remember to identify relevant domain events, define event handlers, dispatch events, and register the handlers with the event bus. With these practices in place, you'll be able to harness the power of event-driven architecture in your Golang projects.
Stay tuned for the next part of our series, where we'll explore advanced event-driven patterns and techniques in Golang. Happy coding!