Domain Services in Golang: Enhancing Modularity and Reusability in Domain-Driven Design
Domain Services in Golang enhance modularity, reusability, and testability in Domain-Driven Design. They encapsulate complex logic, making code maintainable and flexible.
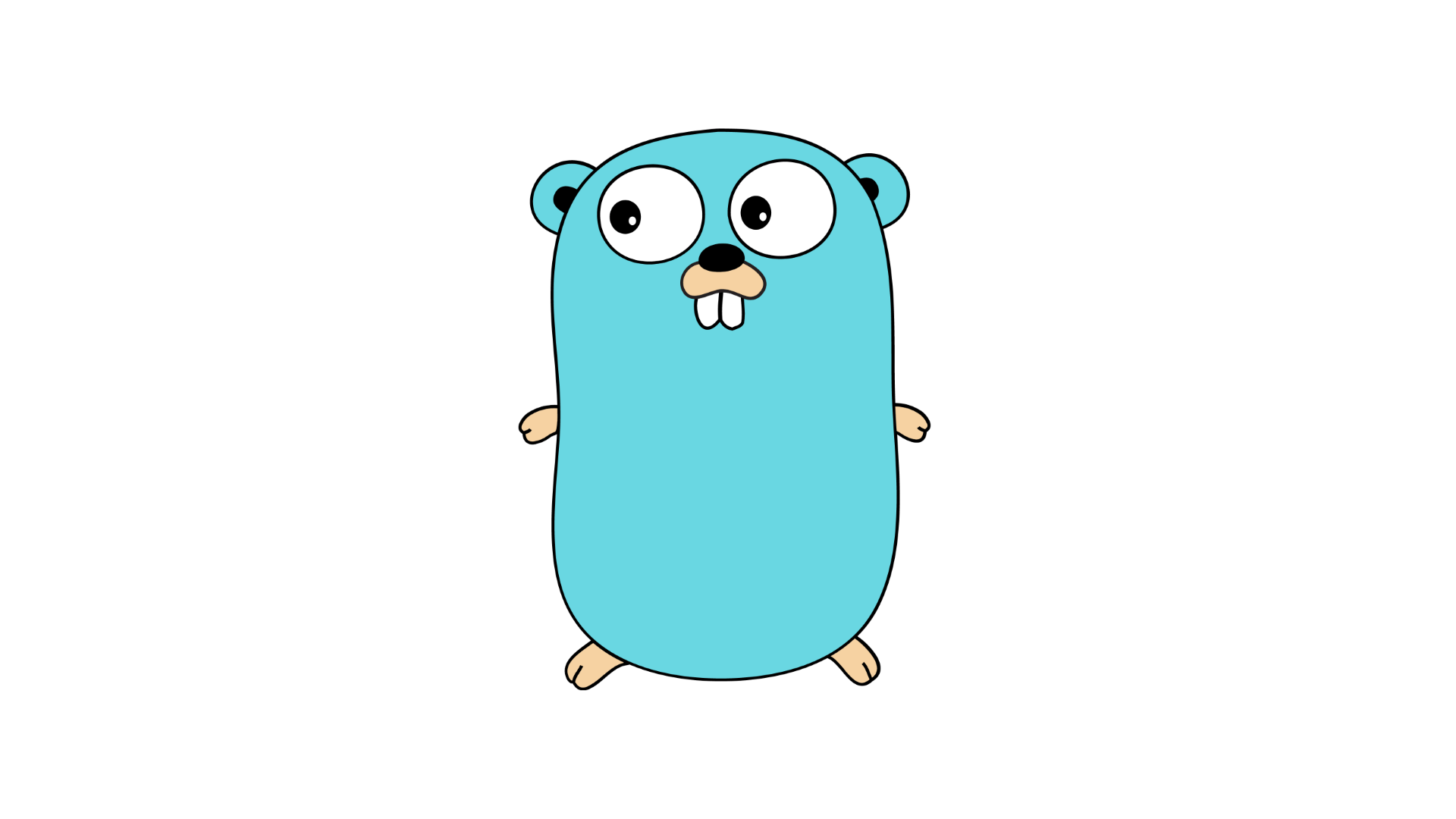
Introduction
Welcome to the world of Go (Golang)! In this blog post, we will explore the concept of Domain Services in Golang and how they enhance modularity and reusability in Domain-Driven Design (DDD). Domain Services play a crucial role in isolating and encapsulating complex business logic, making our codebase more maintainable, reusable, and testable.
What are Domain Services?
In Domain-Driven Design, a Domain Service is a key building block that represents a piece of business logic or behavior that doesn't naturally fit within an Entity or a Value Object. It encapsulates operations that are related to the domain but are not directly associated with any specific entity.
Domain Services are responsible for coordinating and orchestrating complex operations involving multiple entities or value objects. They encapsulate business logic that doesn't belong in any single entity but is still crucial to the domain model.
For example, consider an e-commerce application where customers can purchase products. The process of calculating the total cost of an order, including taxes, discounts, and shipping charges, is a complex operation that involves multiple entities like products, customers, and settings. A Domain Service, such as an OrderService, can encapsulate this logic and provide a clean interface for calculating the total cost.
The Benefits of Domain Services
Using Domain Services in your Golang projects offers several benefits:
Modularity
Domain Services help in achieving modular code by organizing complex business logic into separate units. This allows different parts of the system to be developed, tested, and maintained independently.
Reusability
Domain Services promote code reuse by encapsulating reusable business logic. These services can be shared and utilized across multiple entities within the domain model.
Testability
Domain Services facilitate testability by isolating complex business logic from the rest of the system. Since they act as pure functions, taking inputs and producing outputs, they can be easily tested with unit tests, without the need for complex setup or dependencies.
Implementing Domain Services in Golang
Now let's dive into implementing Domain Services in Golang. Here are a few steps to get you started:
Step 1: Identify the Complex Business Logic
Identify the specific piece of complex business logic that doesn't fit into any single entity or value object. This logic usually involves multiple entities, calculations, validations, or external integrations.
Step 2: Define the Domain Service Interface
Create an interface that defines the contract of the Domain Service. This interface should include the necessary methods that encapsulate the desired behavior. For example:
type OrderService interface {
CalculateTotalCost(order *Order) (float64, error)
// Other methods...
}
Step 3: Implement the Domain Service
Create a concrete implementation of the Domain Service interface. The implementation should contain the actual business logic that performs the required operations. For example:
type orderService struct {
// Dependencies, if any...
}
func (s *orderService) CalculateTotalCost(order *Order) (float64, error) {
// Complex business logic to calculate the total cost...
// Use the order, entities, settings, etc.
// Return the calculated total cost or an error, if any.
}
Step 4: Use the Domain Service
Finally, use the Domain Service in your codebase. Inject the service as a dependency into the required entities or other services.
type ProductService struct {
OrderService OrderService
// Other dependencies...
}
func (s *ProductService) SomeMethod() {
// Use Domain Service methods inside ProductService or other entities...
totalCost, err := s.OrderService.CalculateTotalCost(order)
// Perform other operations...
}
By following these steps, you can effectively implement Domain Services in your Golang projects and enhance modularity and reusability in Domain-Driven Design.
Wrapping Up
Domain Services are powerful tools that enhance modularity and reusability in Domain-Driven Design. By encapsulating complex business logic that doesn't naturally fit within entities or value objects, Domain Services help in organizing code, promoting code reuse, and improving testability. Incorporating Domain Services into your Golang projects can greatly enhance the maintainability and flexibility of your codebase.
Now it's your turn to leverage the power of Domain Services in your Golang projects. Start by identifying the complex business logic that can be encapsulated into a Domain Service, define the interface, implement the service, and inject it into your codebase. Happy coding!